3. Write Python code to define the Ellipse class with a reasonable number of properties and methods. For this assignment, we are learning about inheritance. For the first question, I created a class Circle to inherit from the parent class, Ellipse, using pass. For this question, I had to write a working code using class Ellipse, showing some properties and methods. I wanted to make sure I did it correctly and commented correctly. Any help would be appreciated, thanks. ---------------------------------------------------------------------------------------------------------- import math # create parent class - ellipse class Ellipse: # parent class instance attribute def __init__(self, length, width): self.length = length self.width = width # use math.pi for value of pi # calculate and return area of ellipse def area(self): return math.pi * self.length * self.width # calculate and return perimeter of ellipse def perimeter(self): return math.pi * (self.length + self.width) # create child class - circle class Circle(Ellipse): # child class instance attribute def __init__(self, radius): # super function used to inherit from parent class super().__init__(radius, radius) # to get radius def getRadius(self): return self.length def main(): # enter and read length and width of the ellipse length = float(input('Enter the length of ellipse: ')) width = float(input('Enter the width of ellipse: ')) # create an object of the ellipse class e = Ellipse(length, width) # display ellipse length/width/area/perimeter print('Ellipse Length: ', e.length) print('Ellipse Width: ', e.width) print('Ellipse Area: ', e.area()) print('Ellipse Perimeter: ', e.perimeter()) # enter and read radius of the circle radius = float(input('Enter the radius of circle: ')) # create an object of the circle class c = Circle(radius) # display circle radius/area/perimeter print('Circle Radius: ', c.getRadius()) print('Area: ', c.area()) print('Perimeter: ', c.perimeter()) # call main function to start executing program main()
3. Write Python code to define the Ellipse class with a reasonable number of properties and methods.
For this assignment, we are learning about inheritance. For the first question, I created a class Circle to inherit from the parent class, Ellipse, using pass. For this question, I had to write a working code using class Ellipse, showing some properties and methods. I wanted to make sure I did it correctly and commented correctly. Any help would be appreciated, thanks.
----------------------------------------------------------------------------------------------------------
import math
# create parent class - ellipse
class Ellipse:
# parent class instance attribute
def __init__(self, length, width):
self.length = length
self.width = width
# use math.pi for value of pi
# calculate and return area of ellipse
def area(self):
return math.pi * self.length * self.width
# calculate and return perimeter of ellipse
def perimeter(self):
return math.pi * (self.length + self.width)
# create child class - circle
class Circle(Ellipse):
# child class instance attribute
def __init__(self, radius):
# super function used to inherit from parent class
super().__init__(radius, radius)
# to get radius
def getRadius(self):
return self.length
def main():
# enter and read length and width of the ellipse
length = float(input('Enter the length of ellipse: '))
width = float(input('Enter the width of ellipse: '))
# create an object of the ellipse class
e = Ellipse(length, width)
# display ellipse length/width/area/perimeter
print('Ellipse Length: ', e.length)
print('Ellipse Width: ', e.width)
print('Ellipse Area: ', e.area())
print('Ellipse Perimeter: ', e.perimeter())
# enter and read radius of the circle
radius = float(input('Enter the radius of circle: '))
# create an object of the circle class
c = Circle(radius)
# display circle radius/area/perimeter
print('Circle Radius: ', c.getRadius())
print('Area: ', c.area())
print('Perimeter: ', c.perimeter())
# call main function to start executing program
main()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

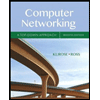
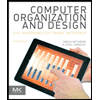
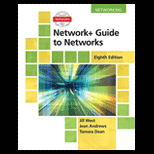
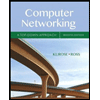
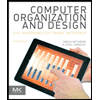
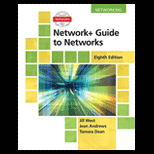
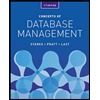
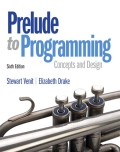
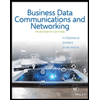