What is JavaScript?
JavaScript is a web programming language. JavaScript is used for the creation and validation of a web page. It is a scripting language that allows users to implement complex features in their web pages. It is used to make dynamic web pages in the website such as showing interactive maps, animated graphics, scrolling video jukeboxes, etc. It is the third most important component of web technology. There are three pillars in web technology which are HTML, CSS, and JavaScript.
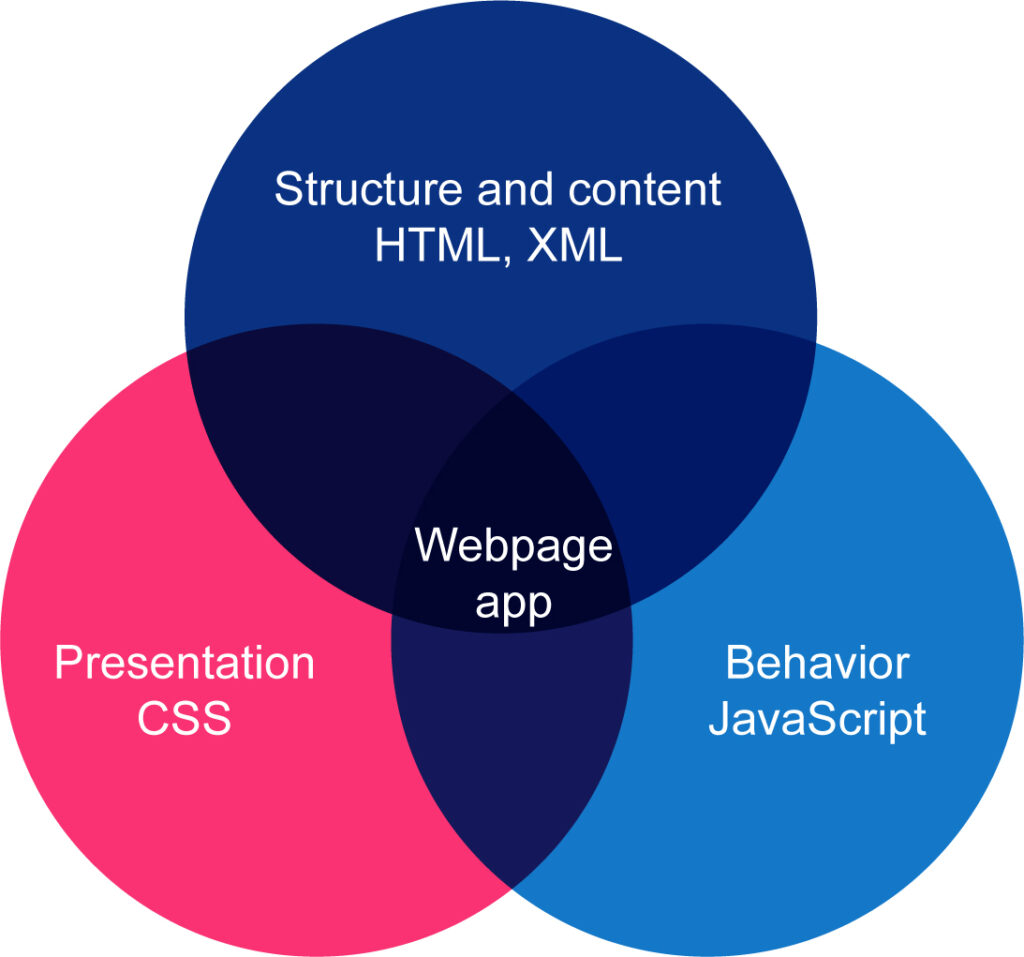
HTML
HTML is an abbreviation of hypertext markup language. It is used to design the structure or layout of web pages. HTML (Hypertext Markup Language) is a computer language that is used to create most web pages and online applications. A hypertext is a piece of text that refers to other pieces of text, whereas a markup language is a set of symbols that instructs web servers about the style and structure of a document.
CSS
CSS stands for Cascading Style Sheets. It is a style sheet language that is used to describe the appearance and formatting of a markup document. It is commonly used in combination with HTML to change the look of web pages and user interfaces. Saving the styles in a separate .css folder will help the web pages to share the same page style. It supports all types of XML documents, including plain XML, SVG, and XUL.
In most websites, CSS is used in combination with HTML and JavaScript to develop user interfaces for web apps and many mobile applications.
JavaScript
JavaScript or JS is a web programming language that has the ability to change or update both HTML and CSS. It is used to calculate, manipulate, and validate data. It is used to create dynamic content on web pages. JS code is stored on a ".js" extension file like javascriptcode.js.
React is a user interface library written in JavaScript. It allows building a complicated user interface out of "components," which are small, isolated pieces of code. Components are reusable HTML elements with content (or data) that may be modified without having to reload the entire website. A user interface (UI) is the place where humans and machines interact in the industrial design field of human-computer interaction. Computer mouse, remote control, virtual reality, ATMs, speedometer are examples of the user interface.
JavaScript variables
A JavaScript variable is nothing more than a storage location's name. When declaring a JavaScript variable, there are some guidelines to follow.
- The name must begin with a letter (a to z or A to Z), underscore(_), or dollar($) sign .
- We can put numerals (0 to 9) after the initial letter, for example value1.
- Variables in JavaScript are case sensitive, so x and X are two separate variables.
- var x = 20;
- var _value="javascript";
Incorrect JavaScript variables
- var 145=78;
- var *jk=367;
In JavaScript, there are two types of variables: local variables and global variables. A local variable in JavaScript is declared within a block or function. It can only be accessed from within the function or block. A global variable in JavaScript can be accessed from any function. A global variable is one that is declared outside of any function or block.
Array
An array is a variable in js code which holds more than one variable. For example:
const fruits = ["apple", "banana', "orange", "papaya", "guava"];
Note: We use a semicolon at the end of each statement in JS code.
Here, fruits is an array variable that holds multiple fruits. An array is defined in a square bracket and string is represented within a single quote or double quote.
Some JavaScript developers do not consider JavaScript as a true object-oriented language due to its lack of class concepts. Let us understand whether JavaScript is object-oriented or not.
Object oriented concepts in JavaScript
JavaScript is widely used in web programming but does JS support object-oriented features. Object-oriented concepts in JavaScript have four main features. The features are:-
- Classes
- Object
- Encapsulation
- Inheritance
Classes
In object-oriented programming, a class is a blueprint for creating objects, providing initial values for the state, and implementations of behavior. But in the JavaScript code, there are no classes defined which means we have only objects in JS code. So we can say that JavaScript is a prototype based on an object-oriented programming language which means it defines the behavior of an object using constructor function and then reuses it.
Example of class in JS:
//Declaring class
class Student
{
//Initializing an object of the class
constructor(id,name)
{
this.id=id;
this.name=name;
}
//Declaring method
detail()
{
document.writeln(this.id+" "+this.name+"<br>")
}
}
//passing object to a variable
var std1=new Student(1001,"Ram");
std1.detail(); //calling method
Object
As we can say that object is the instance of a class. We make many objects using a class template. The object is the real-world entity. Everything is an object such as a car, animal, fruit, etc. Each object has its property and method which defines its characteristics, for example, if 'car' is an object then its characteristics are color, type, model, and it performs certain actions such as driving. These characteristics are called property, and actions are called methods. Objects are present everywhere in JavaScript code, where every element is an object like array, function, string.
An object in created in JS using the below syntax:
var objectname=new Object();
Inheritance
Inheritance is the property of an object-oriented programming language. It is a procedure in which one class inherits the property and methods of another class. The class whose property and methods are inherited by another class is called parent class and the class which inherits parent class property is known as child class. So this is the concept of inheritance.
The extends keyword in JavaScript is used to construct a child class from a parent class. It makes it easier for a child class to inherit all of its parent class's properties and behaviors.
Example of Inheritance:
class Car // it is a parent class
{
constructor()
{
this.company="Tesla";
}
}
class Vehicle extends Car { // it is a child class
constructor(model,price) {
super();
this.model=model;
this.price=price;
}
}
Encapsulation
Encapsulation is defined as the process of wrapping property and function within a single unit. It enables us to manage and validate data. To accomplish encapsulation in JavaScript, do the following:
- To make data members private, use the var keyword.
- Set the data with setter methods and receive the data with getter methods.
let's see an example of encapsulation:
class Employee
{
constructor()
{
var name;
var salary;
}
getName()
{
return this.name;
}
setName(name)
{
this.name=name;
}
getSalary()
{
return this.Salary;
}
setSalary(salary)
{
this.salary=salary;
}
}
Applications of JavaScript
JavaScript is used in many applications. Here are some examples of JS applications:-
- JS is used for game development
- It is used in web development and web technologies.
- It is used to develop server applications and build a web server.
- It allows users to interact with web pages. It makes the web pages user friendly.
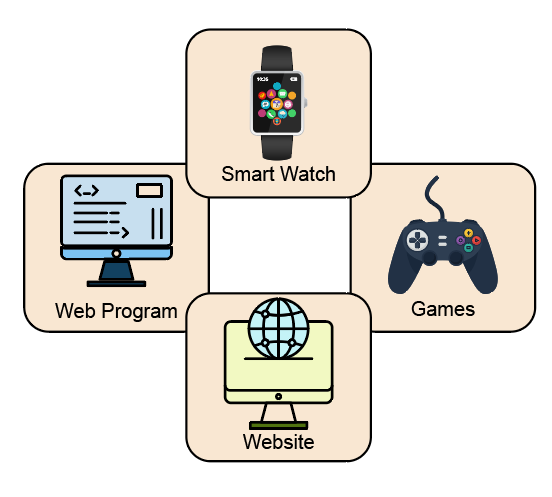
Which browser supports JS?
There are many browsers available that support JS. Some browsers are Chrome, Safari, Microsoft Edge, Opera, etc. But some browsers like IE do not support modern JS language.
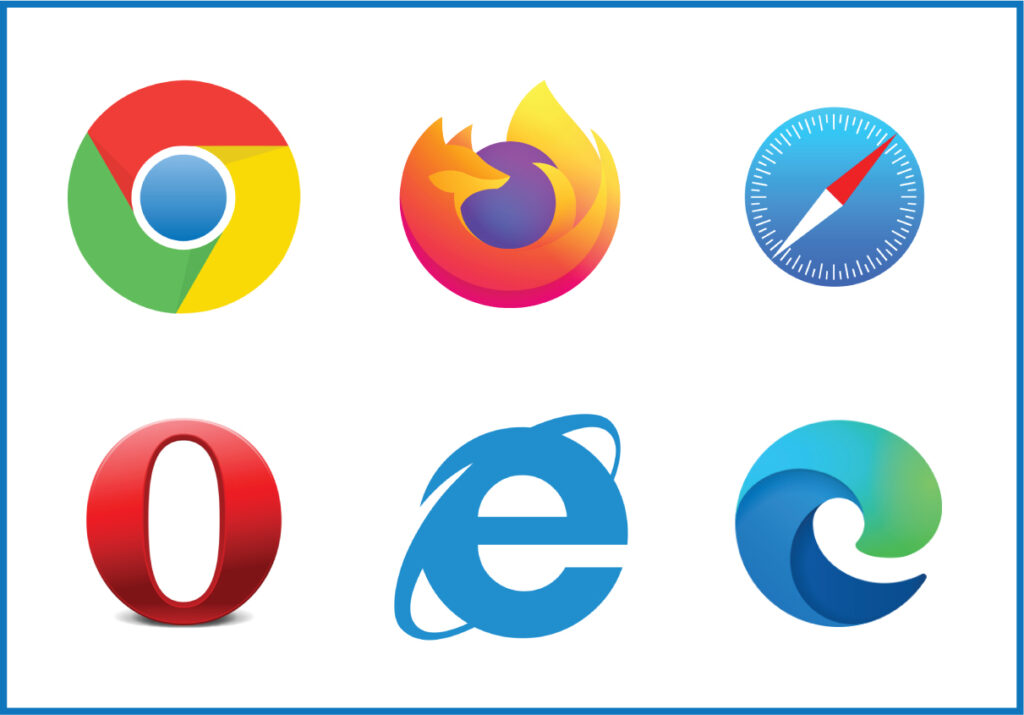
Common Mistakes
Generally, students assume that JS is not an object-oriented language because it does not have class but it is not true. JS is a prototype object-oriented language. It is true that JS is not a class-based object-oriented language but it uses the object as a template from which we get the initial properties for a new object.
Concepts and Applications
This topic is significant in the professional exams for both graduate and postgraduate courses, especially for:
- Bachelor of Technology in Computer science
- Master of Technology in Web Technology
Related Topics
- Client-side JavaScript
- Eloquent JavaScript
- Clouser in JavaScript
- Hoisting in JavaScript
Practice Problems
Q1. Which framework is used for JavaScript?
- Angular
- Django
- Both
- None
Correct answer- Angular
Explanation- Angular framework is the most popular framework used in JS. It is used to develop single page application (SPA).
Q2. JavaScript is a
- Prototype object oriented language
- Scripting language
- Both 1 and 2
- None
Correct answer: Both 1 and 2
Explanation- JavaScript is both scripting language as well as prototypes object oriented language.
Q3. Why JS is called lightweight programming language?
- It does not use class in object oriented concepts.
- It can include programing functionality but does not perform stronger operations like Java.
- It performs stronger operations like C++ .
- It is used in many non-browser environments as well.
Correct answer: 1 and 4.
Explanation- JavaScript is a lightweight programming language because it can contain programming functions, but it cannot contain more complex ones like Java or C ++. In JavaScript, there are no classes in the sense of class-based OOP. JavaScript works with objects.
Q4- JavaScript code is placed in which HTML element?
- <script>
- <body>
- <style>
- <head>
Correct answer: <script>
Explanation- In an HTML document, the javascript code is written inside the <script> tag.
Q5. JS creates dynamic web pages.
- True
- False
correct answer- True
Explanation- JavaScript can query and modify the page's state using the Document Object Model. Thus, JS creates dynamic web pages.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
JavaScript Homework Questions from Fellow Students
Browse our recently answered JavaScript homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.