What is a computing algorithm?
A computing algorithm is a set of instructions or a process for solving computational problems. In other words, it contains a set of rules that indicate the step-wise process to solve a problem. The algorithm takes a set of inputs, executes the instructions given to it, and produces the desired output.
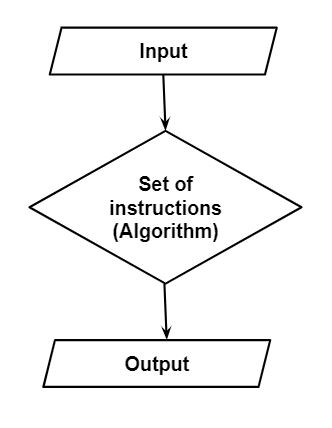
Features of a computing algorithm
In computer programming, algorithms must possess certain characteristics. These include:
- It should be clear and precise.
- The input and output should be mentioned clearly.
- It should never be infinite. The algorithm should terminate after a certain number of finite steps.
- The algorithm should not be dependent on future technology. It should be executable with the available resources.
- Algorithms should be language-independent. This means the algorithm is merely a set of instructions given in natural language, and it execution can be done in any programming language.
Steps to create a computing algorithm
The step-by-step approach for creating a computing algorithm is as follows:
Step 1: Gather the pre-requisite:
- Define the problem.
- Specify the constraints that should be considered for solving the problem.
- Specify the inputs of the problem.
- Specify the desired output.
- The solution to the problem, in the specified constraints.
Step 2: Design the algorithm using the above information.
After designing the algorithm, it can be tested by implementing it in different computer programming languages like C++, C, Java, and Python.
Examples of algorithms
Consider the following computer algorithms:
Example 1: An algorithm that adds two numbers and prints their sum.
Step 1: Gather the pre-requisite:
- Define the problem- The problem is to add two numbers and print the answer.
- Specify the constraints that should be considered for solving the problem- The numbers should be digits and not characters.
- Specify the inputs of the problem- Two numbers that are to be added.
- Specify the desired output- The sum of the two numbers given as input.
- The solution to the problem, in the specified constraints- The solution will contain the addition of the two numbers and will be obtained using the plus (+) operator.
Step 2: Design the algorithm:
The algorithm to add two numbers will be as follows:
Begin
Declare two integer variables a, b.
Read the two variables as inputs.
Declare another integer variable answer to store the result.
Add the two numbers and store the result in the variable answer.
Print the value of the variable answer.
End
Example 2: An algorithm to find the larger of the two numbers.
Step 1: Gather the pre-requisite:
- Define the problem- The problem is to find the larger number from the given two numbers.
- Specify the constraints that should be considered for solving the problem - The numbers should be digits and not characters.
- Specify the inputs of the problem- Two numbers.
- Specify the desired output- The larger number of the two numbers given as input.
- The solution to the problem, in the specified constraints- The solution will contain the number, which is the larger one from the two given numbers. The output will be obtained using the greater than (>) operator.
Step 2: Design the algorithm:
The algorithm to find the larger number amongst two numbers will be as follows:
Begin
Declare two integer variables a, b.
Read the two variables as inputs.
If a > b
Display a is the larger number.
Else
Display b is the larger number.
End
Commonly used algorithms
In computer programming, several types of algorithms are used. Each algorithm has a specific use. Below are the most commonly used computing algorithms:
- Linear search algorithm- This algorithm searches for an element from a given unsorted sequence.
- Binary search algorithm- This algorithm searches for an element from a sorted sequence.
- Dijkstra’s algorithm- It calculates the shortest path in a graph having positive weight edges.
- Floyd-Warshall algorithm- It finds the shortest path in a weighted, directed graph having negative or non-negative edges.
- Longest path problem- This algorithm searches for the path having the maximum length in a graph.
- Divide-and-conquer algorithm- This algorithm recursively divides a problem into several sub-problems until it becomes simple to solve the sub-problems directly. After solving the sub-problems, the solutions of the sub-problems are combined to obtain the solution for the main problem.
- Sorting algorithms- Sorting algorithms are used to arrange elements of a list into a specific order. This order can be ascending, descending, numerical, or any other order. Examples of sorting algorithms include heap sort, merge sort, quick sort, insertion sort, bubble sort, merge sort, and selection sort.
- Breadth-First search- This algorithm traverses a graph level-wise. It begins from the root node and traverses through all nodes at the current depth before moving to the nodes on the next level.
- Depth-First search- This algorithm traverses a graph branch-wise. It begins from the root node and traverses downwards on each branch until it reaches the leaf node and then backtracks.
Advantages of algorithms
The advantages of using algorithms in computer programming include:
- They are easy to understand.
- They give a step-by-step representation of the solution for a specific problem.
- Algorithms break down the problem into smaller steps, making it easier to implement the program.
- Individuals having less knowledge about programming languages can understand algorithms since algorithms are language-independent.
Disadvantages of algorithms
Disadvantages of using computer algorithms are as mentioned below:
- The process of writing algorithms is time-consuming.
- Defining the branching and looping statements is difficult in algorithms.
- It becomes complex to define bigger tasks.
Difference between an algorithm and a pseudocode
Algorithm | Pseudocode |
It is a set of instructions that show how to solve a specific problem. | It is a method used for expressing an algorithm for a computer program. |
Algorithms are often hard to interpret as compared to pseudocodes. | Pseudocodes can be interpreted easily. |
Designing algorithms is complex. | Designing pseudocodes is simpler. |
Debugging algorithms is hard. | Debugging pseudocodes is easier. |
Context and Applications
The topic of computing algorithms is important in data structure and algorithms. The concept is taught in various computer science-related courses such as:
- Bachelors of Science in Computer Science
- Masters of Science in Computer Science
- Bachelors of Technology in Computer Science Engineering
- Masters of Technology in Computer Science Engineering
Practice Problems
1. What is an algorithm?
- A set of instructions that define how to solve a problem.
- Alan Turing machine process
- MIT Press include
- Set of programs
Answer: Option a
Explanation: An algorithm is a set of rules that state how to solve a problem. It defines the process to solve a computational problem.
2. Which of these is a feature of algorithms?
- Machine learning
- It is language-independent
- It is complex
- It is a dynamic programming approach
Answer: Option b
Explanation: One of the features of algorithms is that they are language-independent. This means they are not written based on a specific computer programming language.
3. Which algorithm traverses a tree branch-wise?
- Object-oriented
- Breadth-first search
- Binary search
- Depth-first search
Answer: Option d
Explanation: The depth-first search algorithm traverses a tree branch-wise. It starts traversing from the root node and moves downwards until it reaches the deepest node. Then, it starts backtracking.
4. What is the first step in designing an algorithm?
- Gather pre-requisite
- Design algorithm
- Define variables
- Define output and input data
Answer: Option a
Explanation: The first step in designing an algorithm is to gather the pre-requisite. All the basic requirements needed in designing the algorithm are specified at this stage.
5. Which of these is a type of algorithm?
- Process
- Turning
- Sorting
- Computing
Answer: Option c
Explanation: The different types of algorithms include binary search, Dijkstra's, Linear search, divide-and-conquer, and sorting algorithm. Hence, option c is correct.
Common Mistakes
The terms pseudocode and algorithm are often used interchangeably by students. However, these terms have different meanings. So, students should not mix them or interchange them.
Related Concepts
- Flowcharts
- Greedy algorithms
- Artificial intelligence
- Object-oriented programming
- Analysis of algorithms
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Computing Algorithms Homework Questions from Fellow Students
Browse our recently answered Computing Algorithms homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.