Your task for this assignment is to use the C++ standard library sort() function to sort numbers in an array. 1. Use the sort() routine from the C++ standard library with an array to sort 10 random numbers. The program initializes an array with 10 random 2-digit integers (positive and negative) using the rand() function. The program then sorts the numbers in the array using the sort() function. 2. The program displays on one line the original values in the array before the sort begins. After the sort, the program displays on one line the numbers in sorted order, lowest to highest. 3. Your C++ program is named csc231_prog4_yourlastname.cpp. Your C++ program contains comments starting on line 1 containing this information: a. the ID, section and name of the course b. your name c. this file name d. the program assignment number and due date e. the program purpose You are always encouraged to add additional comments throughout the program that your feel might be helpful to the reader of your source code. 4. Submit your C++ program source code file as an attachment to an email message to kbyron@bmcc.cuny.edu using a subject in this form: “csc231_prog4_yourlastname”. For 66.7% extra credit, add the sort_heap() function to your program. For each sort algorithm, sort the same random numbers. Display the original numbers on one line before sorting. After each sort, display a message identifying the sort algorithm used followed by the sorted numbers on one line. Ref: sort(): https://www.geeksforgeeks.org/sort-c-stl/ sort_heap(): https://www.geeksforgeeks.org/sort_heap-function-in-c/ sort_heap(): https://www.cplusplus.com/reference/algorithm/sort_heap/ rand(): https://www.cplusplus.com/reference/cstdlib/rand/
Your task for this assignment is to use the C++ standard library sort() function to sort numbers in an array.
1. Use the sort() routine from the C++ standard library with an array to sort 10 random numbers. The
2. The program displays on one line the original values in the array before the sort begins. After the sort, the program displays on one line the numbers in sorted order, lowest to highest.
3. Your C++ program is named csc231_prog4_yourlastname.cpp. Your C++ program contains comments starting on line 1 containing this information:
a. the ID, section and name of the course
b. your name
c. this file name
d. the program assignment number and due date
e. the program purpose
You are always encouraged to add additional comments throughout the program that your feel might be helpful to the reader of your source code.
4. Submit your C++ program source code file as an attachment to an email message to kbyron@bmcc.cuny.edu using a subject in this form: “csc231_prog4_yourlastname”.
For 66.7% extra credit, add the sort_heap() function to your program. For each sort
Ref: sort(): https://www.geeksforgeeks.org/sort-c-stl/
sort_heap(): https://www.geeksforgeeks.org/sort_heap-function-in-c/
sort_heap(): https://www.cplusplus.com/reference/algorithm/sort_heap/
rand(): https://www.cplusplus.com/reference/cstdlib/rand/

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

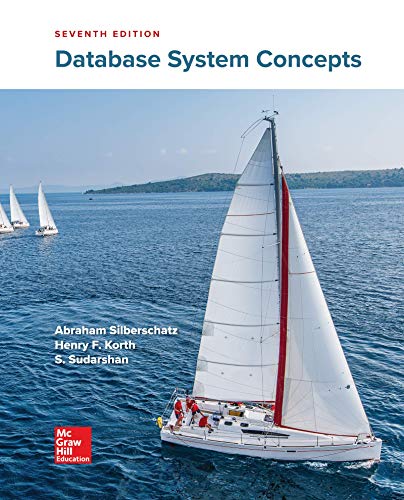
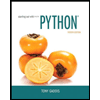
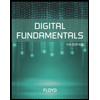
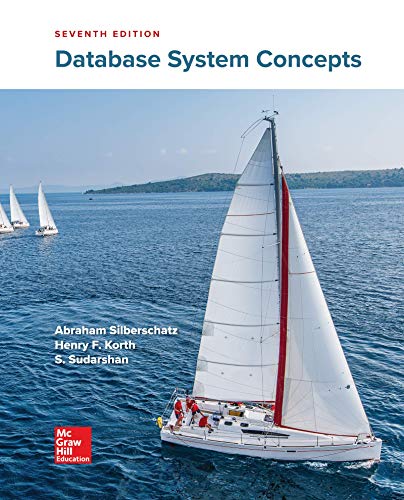
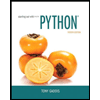
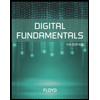
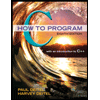
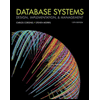
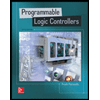