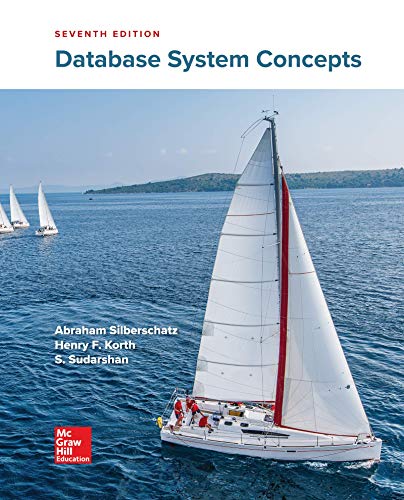
Your program must use command line options:
$ python3 gene_information_query.py --help
- Your program submission must have a subdirectory assignment5 folder inside your assignment5 working directory (like I did in assignment #4- see below). Note the __init__.py, there are 3 of them.assignment5 ├── assignment5 │ ├── __init__.py │ ├── config.py │ └── my_io.py ├── gene_information_query.py └── tests ├── __init__.py └── unit ├── __init__.py ├── test_config.py └── test_my_io.py
- Implement an assignment5.my_io.py to open any files. And also a test script (test_my_io.py) for the module to be tested by pytest.
- Implement an assignment5.config.py module to be a small configuration module. Also write a test script (test_config.py) for the module to be tested by pytest.
- You can use modules argparse (Links to an external site.), os (Links to an external site.), re (Links to an external site.), sys, (Links to an external site.)or collections (Links to an external site.) if you like. You cannot use any other Python module
- See additional notes at the bottom of the page.
-
Write a function (call it modify_host_name) that receives one argument: 1). A host name. This function take the host name and checks for the available conversions from common to scientific names and return the scientific name (this will be the eventual species to be used in the directory name). This function should call get_host_keywords to get the dictionary of mapped terms from the assignment5.config.py module. If the host passed in does not exist then it should call the function _print_host_directories (see below) to alert the user what directories do exist (see outputs below) and the exit the program.
2). Write another function (Put this in my_io.py) (call it is_valid_gene_file_name) which receives one argument. 1). A file name. This function will check to make sure the given file name exists, if it does it return True else it will return FalseSee the os.path.exists (Links to an external site.) in Python

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Look at the code below and use the following comments to incdicate the scope of the static variables or functions. Place the comment below the relevant line. Module scope Class scope Function scope The code runs. Use onlinegdb.com to see it run. Module scope means global but only known in this source file. Class scope means global but only known by the class. Function scope means global but only known in the function. Hide Comments 1 #include 2 static const int MAX_SIZE=10; 5 // Return the max value 6 static double max(double d1) 7 { static double lastMax = 0; lastMax = (di > lastMax) ? di : lastMax; return lastMax; 8 10 11 } 12 13 // singleton class only one instance allowed 14 class singleton 15 { public: static Singleton& getsingleton() { return theone; } // Returns the Singleton 16 17 18 19 20 friend std::ostream& operator<< (std::ostream& o, const Singleton& s); private: Singleton() { }; // Prevents more instances 21 22 23 24 25 static Singleton theone; 26 }; 27 Singleton…arrow_forwardWhen a file’s header is not located and the extension is not know the file is reported as a(n) ____. alias unknown Bad Signature non-conventionalarrow_forward# file name: w07_markus.py## Complete the following steps:# (1) Complete the following function according to its docstring.# (2) Save your file after you make changes, and then run the file by# clicking on the green Run button in Wing101. This will let you call your# modified function in the Python shell. An asterisk * on the Wing101# w07_markus.py tab indicates that modifications have NOT been saved.# (3) Test your function in the Wing101 shell by evaluating the examples from# the docstring and confirming that the correct result is displayed.# (4) Test your function using different function arguments.# (5) When you are convinced that your function is correct, submit your# modified file to MarkUs. You can find instructions on submitting a file# to MarkUs in Week *2* Perform -> Accessing Part 2 of the# Week 2 Perform (For Credit) on PCRS.# (6) Verify you have submitted the right file to MarkUs by downloading it# and checking that the…arrow_forward
- 40. The value of the data item prior to the write is called as _________ a. Transaction identifier b. Data-item identifier c. Old value d. New valuearrow_forwardIn the following pseudocode of creating a trigger, the first UPDATE is the ____ of the trigger, and the second UPDATE is the ____ of the trigger. CREATE TRIGGER MyTrigger AFTER UPDATE OF attr ON MyTable ... WHEN ... UPDATE MyTable .... Group of answer choices Action; action Condition; action Event; action Event; conditionarrow_forwardI have to create a database using python to show geographical points. Create a program the will create your database and insert at least five points. Use your own data, something like: 100, 200, 123, Main Campus 120, 133, 142, Montoya 153, 123, 322, Rio Rancho 133, 123, 143, STEMULUS Center 153, 142, 122, ATC Run your initialization code to create the database.arrow_forward
- Your application will demonstrate the ability to retrieve 10 numbers from a user and determine the smallest and largest number from the list. Incorporate the following requirements into your application: The program will consist of one file - the main application class Name the class appropriately and name the file Program.cs Include documentation at the top of the file that includes Your name Date of development Assignment (e.g., CIS214 Midterm Practical Exam) Description of the class The main application class must meet the following requirements Print a line that states "Your Name - Midterm Practical Exam" Get 10 integer values from the user and store them in an array or a List Write a method to determine the smallest element in the collection Write a method to determine the largest element in the collection Display the following information for the collection All the elements in the collection and the index of the element (displayed in columns as shown in the example…arrow_forwardWhen the Search button is pressed in the screen depicted, the getSales() function from the PosDAO.java class is called, the parameters of this function are the from date and to date.The getSales () function should return a list of Pos objects that matches the criteria specified, Note this function has to perform two INNER JOINs in to combine all three tables in order to get all the needed data to fill the Pos object. The database schema is also depicted. The PosDAO, Product, SalesDetails and Pos classes are shown BELOW Write the getSales() function public class PosDAO { private Connection conn; PreparedStatement stmt = null; public boolean openConnection(){ try { // db parameters String url = "jdbc:mysql://localhost:4306/swen2005"; String user = "root"; String password = ""; // create a connection to the database conn = DriverManager.getConnection(url, user, password); if (conn!= null){ return true;…arrow_forward1) Create a folder (directory) called "sqlite" (without the quotes) in your base drive (eg. C:\sqlite) 2) Create a Database called "test.db" (without the quotes) in C:\sqlite using Sqlite DBBrowser (Make sure the extension is .db – not .sqlite) 3) In test.db create a table called book. The table should have the following columns: Id – Integer, Not Null, Unique, Primary Key, Auto Increment Author – Text, Not Null Title - Text Not Null Year - Integer, Not Null Price - Real, Not Null - Add some rows with data for the above table. Create the user interface shown below in WindowBuilder. Write a WindowBuilder Java program to do the following: (Everything should be in a SINGLE Java program). To make connection to the Database, use the SqliteConnection.java and sqlite-jdbc.jar file provided in Canvas under Modules->Chapter Code (Do not use your own files for these two). Display Records Author: Title: Year: Price: Find Updatearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
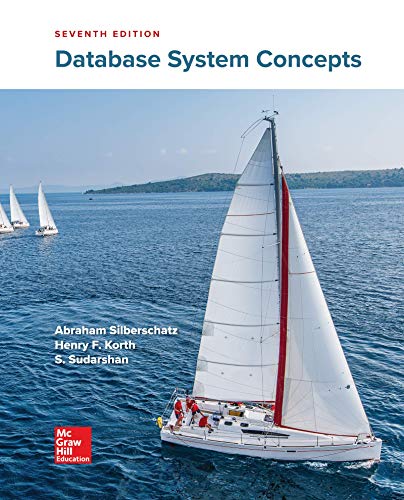
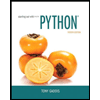
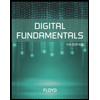
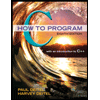
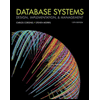
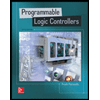