you will develop a c++ program to sort the elements of a character array in descending order. Your program should ask the user to input 7 elements (of type char) for the array and sort it. For instance, if the user enters d a b e c x g, your program should display: x g e d c b a Note: You cannot use the built-in sort function. Also, you can assume that the user only enters lower-case characters.
you will develop a c++ program to sort the elements of a character array in descending order. Your program should ask the user to input 7 elements (of type char) for the array and sort it. For instance, if the user enters d a b e c x g, your program should display: x g e d c b a
Note: You cannot use the built-in sort function. Also, you can assume that the user only enters lower-case characters.

#include <iostream>
using namespace std;
int main()
{
char arr[7];
for(int i=0;i<7;i++){
cin>>arr[i];
}
// use bubble sort
for(int i=0;i<7;i++){
for(int j=i+1;j<7;j++){
if(arr[i]<arr[j]){
char temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
cout<<"Sorted array is ";
for(int i=0;i<7;i++){
cout<<arr[i]<<" ";
}
return 0;
}
Step by step
Solved in 2 steps with 2 images

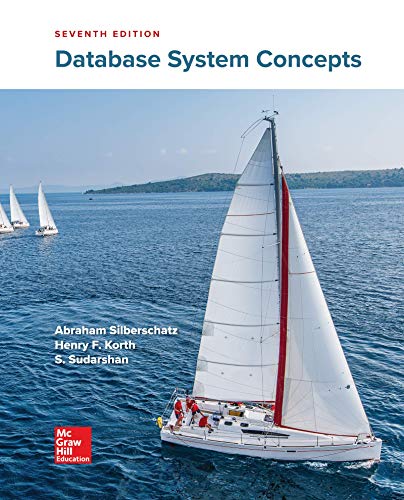
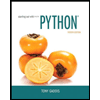
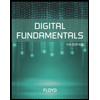
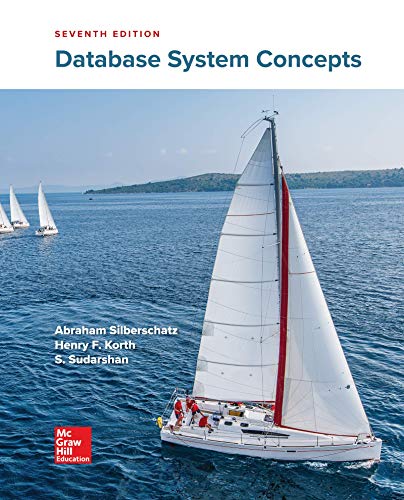
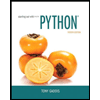
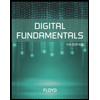
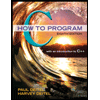
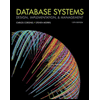
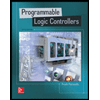