You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back() Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:". Write a function that takes a vector a doubles and reverses the order of all the elements of the vector. Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user. Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each of your calls to 2 and 3. There should be 3 different functions for each step. Than called in to the Main one.
You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back() Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:". Write a function that takes a vector a doubles and reverses the order of all the elements of the vector. Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user. Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each of your calls to 2 and 3. There should be 3 different functions for each step. Than called in to the Main one.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back()
- Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:".
- Write a function that takes a vector a doubles and reverses the order of all the elements of the vector.
- Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user.
- Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each of your calls to 2 and 3.
There should be 3 different functions for each step. Than called in to the Main one.

Transcribed Image Text:You are to write a complete program that implements the following:
You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back()
1. Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:".
2. Write a function that takes a vector a doubles and reverses the order of all the elements of the vector.
3. Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user.
4. Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each of your calls to 2 and 3.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
#include <iostream>
#include <vector >
using namespace std;
//Function 1
void vectorOutput(vector<double> doubleVector) {
cout<<"Current Vector Contents: ";
for (inti = 0; i < doubleVector.size(); i++) {
cout<<doubleVector.at(i) <<", ";
}
cout<<endl;
}
//Function 2
vector<double> reverseVector(vector<double> doubleVector) {
intj = 0;
for (inti = 0, j = (doubleVector.size() - 1); i < (doubleVector.size() / 2); i++, j--) {
doublervector = doubleVector[i];
doubleVector.at(i) = doubleVector.at(j);
doubleVector.at(j) = rvector;
}
returndoubleVector;
}
//Function 3
vector<double> vectorInput(vector<double> doubleVector) {
cout<<"Enter positive double numbers: "<<endl;
while (true) {
doubleposVector;
cin>>posVector;
if (posVector < 0) {
break;
}
else {
doubleVector.push_back(posVector);
}
}
returndoubleVector;
}
int main() {
vector<double> doubleVector;
vector<double> revVector;
unsignedinti = 0;
vectorOutput(doubleVector);
revVector=reverseVector(doubleVector);
vectorOutput(revVector);
doubleVector=vectorInput(doubleVector);
vectorOutput(doubleVector);
return0;
}
This is my code and for some reason, my output is
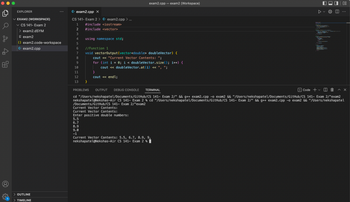
Transcribed Image Text:EXPLORER
EXAM2 (WORKSPACE)
CS 141- Exam 2
> exam2.dSYM
E exam2
{} exam2.code-workspace
G+ exam2.cpp
> OUTLINE
> TIMELINE
G+ exam2.cpp X
CS 141- Exam 2 > G+ exam2.cpp > ...
1 #include <iostream>
2
#include <vector>
3
4
5
6
7
8
9
10
11
12
13
using namespace std;
//Function 1
void vectorOutput (vector<double> doubleVector) {
cout << "Current Vector Contents: ";
for (int i = 0; i < double Vector.size(); i++) {
cout << doubleVector.at (i) << ", ";
}
}
cout << endl;
exam2.cpp - exam2 (Workspace)
Enter positive double numbers:
5.5
6.7
8.9
9.0
-1
sed Teate
Current Vector Contents: 5.5, 6.7, 8.9, 9,
nekshapatel@Nekshas-Air CS 141- Exam 2 %
To BR. 3 Oct 20 (ar) / 200m, 2008
Tarde estar Depar
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL
Code +
cd "/Users/nekshapatel/Documents/GitHub/CS 141- Exam 2/" && g++ exam2.cpp -o exam2 && "/Users/nekshapatel/Documents/GitHub/CS 141- Exam 2/"exam2
nekshapatel@Nekshas-Air CS 141- Exam 2 % cd "/Users/nekshapatel/Documents/GitHub/CS 141- Exam 2/" && g++ exam2.cpp -o exam2 && "/Users/nekshapatel
/Documents/GitHub/CS 141- Exam 2/"exam2
Current Vector Contents:
Current Vector Contents:
cary
Solution
Follow-up Question
Hello, I tried the this code, but I am not getting any output.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
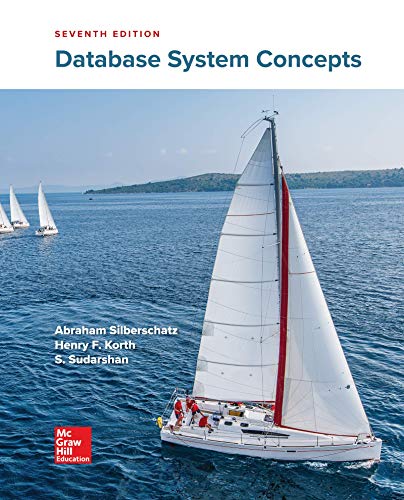
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
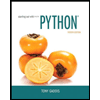
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
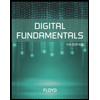
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
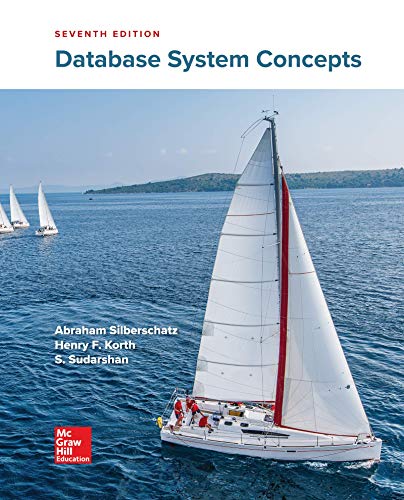
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
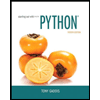
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
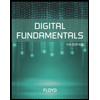
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
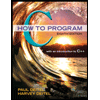
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
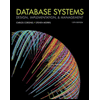
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
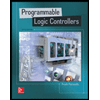
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education