You have been provided with the html file domjs,html that represents the data entry form shown below. Examine this file in your browser. You will notice that some of the form elements have the CSS class hilightable specified in their class attribute. Add listeners to the focus and blur events of all elements that have this hilightable class. In your event handlers for these two events, simply toggle the class highlight (which is in the provided CSS file). This will change their styling of the current form element. Be sure to setup these listeners after the page has loaded. You will notice that some of the form elements have the CSS class required specified in their class attribute. We will not submit the form if these elements are empty. Add an event handler for the submit event of the form. In this handler, if any of the required form elements are empty, add the CSS class error to any of the empty elements. As well, cancel the submission of the form (hint: use the preventDefault () method). Add the Appropriate handler for these required controls that will remove the CSS class error that have changed content. domjs.html Chapter 9 Edit Art Work Details Title Description Genre Subject Medium Year Museum Type Painting Sculpture Image Creative Commons Specification Attribution Noncommercial No Derivative Works Share Alike domjs.js /* add code here */ style.css @import url(https://fonts.googleapis.com/css?family=Open+Sans); @import url(https://fonts.googleapis.com/css?family=Merriweather); /* general text formatting */ h1, h2, h3, legend { font-family: 'Merriweather', serif; } body { font-family: 'Open Sans', Arial, sans-serif; font-size: 16px; } table { width: 90%; margin: 0 auto; } table tbody td{ vertical-align: top; } legend { background-color: #616161 ; color: white; margin: 0 auto; width: 90%; padding: 0.25em; text-align: center; font-weight: bold; font-size: 24px; } fieldset { margin: 1em auto; background-color: #F5F5F5; width: 70%; } form p { margin-top: 0.5em; } form input[type="text"], form select { font-size: 16px; height: 24px; padding: 3px; } form select { height: 30px; } #title, #description { width: 100% } #medium, #museum { width: 90% } #year { width: 40%; } .box { border: 1pt solid #9E9E9E; padding: 0.5em; margin-bottom: 0.4em; } .rectangle { background-color: #BDBDBD; padding: 0.5em; margin-bottom: 5px; } .centered { text-align: center; } .highlight { background-color: #FFE0B2; } .error { background: #FFCDD2 url(error.png) no-repeat 98% center; box-shadow: 0 0 5px #FF5252; border-color: #FF1744; } .btn { -webkit-border-radius: 3; -moz-border-radius: 3; border-radius: 3px; height: 32px; color: black; font-size: 14px; background: #FF9100; padding: 5px 20px 5px 20px; text-decoration: none; } .btn:hover { background: #FFAB40; text-decoration: none; }
- You have been provided with the html file domjs,html that represents the data entry form shown below. Examine this file in your browser.
- You will notice that some of the form elements have the CSS class hilightable specified in their class attribute. Add listeners to the focus and blur events of all elements that have this hilightable class. In your event handlers for these two events, simply toggle the class highlight (which is in the provided CSS file). This will change their styling of the current form element. Be sure to setup these listeners after the page has loaded.
- You will notice that some of the form elements have the CSS class required specified in their class attribute. We will not submit the form if these elements are empty. Add an event handler for the submit event of the form. In this handler, if any of the required form elements are empty, add the CSS class error to any of the empty elements. As well, cancel the submission of the form (hint: use the preventDefault () method).
- Add the Appropriate handler for these required controls that will remove the CSS class error that have changed content.
domjs.html
<!DOCTYPE html>
<html lang="en">
<head >
<meta charset="utf-8">
<title>Chapter 9</title>
<link rel="stylesheet" href="css/styles.css" />
<script type="text/javascript" src="js/domjs.js"></script>
</head>
<body>
<form method="get" action="" id="mainForm">
<fieldset>
<legend>Edit Art Work Details</legend>
<table>
<tr>
<td colspan="2">
<p>
<label>Title</label><br/>
<input type="text" name="title" id="title" class="highlight-required" />
</p>
<p>
<label>Description</label><br/>
<input type="text" name="description" id="description" class="highlight-required">
</p>
</td>
</tr>
<tr>
<td>
<p>
<label>Genre</label><br/>
<select name="genre" class="hilightable">
<option>Choose genre</option>
<option>Abstract</option>
<option>Baroque</option>
<option>Gothic</option>
<option>Renaissance</option>
</select>
</p>
<p>
<label>Subject</label><br/>
<select name="subject" class="hilightable">
<option>Choose subject</option>
<option>Animals</option>
<option>Landscape</option>
<option>People</option>
</select>
</p>
<p>
<label>Medium</label><br/>
<input type="text" name="medium" id="medium" class="hilightable"/>
</p>
<p>
<label>Year</label><br/>
<input type="text" name="year" id="year" class="highlight-required"/>
</p>
<p>
<label>Museum</label><br/>
<input type="text" name="museum" id="museum" class="hilightable"/>
</p>
</td>
<td>
<div class="box">
<label>Type </label><br/>
<input type="radio" name="type" value="1" checked>Painting<br/>
<input type="radio" name="type" value="2">Sculpture<br/>
</div>
<div class="box">
<label>Image Creative Commons Specification </label><br/>
<input type="checkbox" name="cc" >Attribution <br/>
<input type="checkbox" name="cc" >Noncommercial <br/>
<input type="checkbox" name="cc" >No Derivative Works <br/>
<input type="checkbox" name="cc" >Share Alike
</div>
</td>
</tr>
<tr>
<td colspan="2">
<div class="rectangle centered">
<input type="submit" class="btn"> <input type="reset" value="Clear Form" class="btn">
</div
</td>
</tr>
</table>
</fieldset>
</form>
</body>
</html>
domjs.js
/* add code here */
style.css
@import url(https://fonts.googleapis.com/css?family=Open+Sans);
@import url(https://fonts.googleapis.com/css?family=Merriweather);
/* general text formatting */
h1, h2, h3, legend {
font-family: 'Merriweather', serif; }
body {
font-family: 'Open Sans', Arial, sans-serif;
font-size: 16px;
}
table {
width: 90%;
margin: 0 auto;
}
table tbody td{
vertical-align: top;
}
legend {
background-color: #616161 ;
color: white;
margin: 0 auto;
width: 90%;
padding: 0.25em;
text-align: center;
font-weight: bold;
font-size: 24px;
}
fieldset {
margin: 1em auto;
background-color: #F5F5F5;
width: 70%;
}
form p {
margin-top: 0.5em;
}
form input[type="text"], form select {
font-size: 16px;
height: 24px;
padding: 3px;
}
form select {
height: 30px;
}
#title, #description { width: 100% }
#medium, #museum { width: 90% }
#year { width: 40%; }
.box {
border: 1pt solid #9E9E9E;
padding: 0.5em;
margin-bottom: 0.4em;
}
.rectangle {
background-color: #BDBDBD;
padding: 0.5em;
margin-bottom: 5px;
}
.centered {
text-align: center;
}
.highlight {
background-color: #FFE0B2;
}
.error {
background: #FFCDD2 url(error.png) no-repeat 98% center;
box-shadow: 0 0 5px #FF5252;
border-color: #FF1744;
}
.btn {
-webkit-border-radius: 3;
-moz-border-radius: 3;
border-radius: 3px;
height: 32px;
color: black;
font-size: 14px;
background: #FF9100;
padding: 5px 20px 5px 20px;
text-decoration: none;
}
.btn:hover {
background: #FFAB40;
text-decoration: none;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

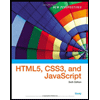
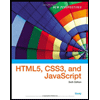