You are writing a program to calculate the average overall scores for two divers in a diving competition. The competition has 5 judges, each from a different country. Each judge will award each diver a score between 0 and 10. Your program will include the following functions • getScore: One double reference parameter. In this function, ask for the name of the country giving the score and the score amount. If the score amount is invalid (i.e. not between 0 and 10), ask again. Return the country name and "return" the score (i.e. use a reference parameter). • findSmallest: Five double parameters. The smallest amount is returned. • findLargest: Five double parameters. The largest amount is returned. • getAverage: Five double parameters. The lowest and highest scores should be dropped, and the remaining three should be averaged. You can determine which three should be averaged by adding all five numbers together, then calling findSmallest and findLargest and subtracting both return results from the total. Then, average the remaining score. Return the result. • scoreDive: This function will return the average overall score for a single diver. It should call getScore five times. Afterwards, print out each country's name and corresponding score. Finally, call getAverage (passing the five scores) and return the result. In main, call the scoreDive function twice, once for diver 1 and once for diver 2. Then, print the final score earned by each.
You are writing a program to calculate the average overall scores for two divers in a diving competition. The competition has 5 judges, each from a different country. Each judge will award each diver a score between 0 and 10. Your program will include the following functions • getScore: One double reference parameter. In this function, ask for the name of the country giving the score and the score amount. If the score amount is invalid (i.e. not between 0 and 10), ask again. Return the country name and "return" the score (i.e. use a reference parameter). • findSmallest: Five double parameters. The smallest amount is returned. • findLargest: Five double parameters. The largest amount is returned. • getAverage: Five double parameters. The lowest and highest scores should be dropped, and the remaining three should be averaged. You can determine which three should be averaged by adding all five numbers together, then calling findSmallest and findLargest and subtracting both return results from the total. Then, average the remaining score. Return the result. • scoreDive: This function will return the average overall score for a single diver. It should call getScore five times. Afterwards, print out each country's name and corresponding score. Finally, call getAverage (passing the five scores) and return the result. In main, call the scoreDive function twice, once for diver 1 and once for diver 2. Then, print the final score earned by each.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++ Question
Hello, Please create the correct code for the attached picture. Create the code based on the given requirements. PLEASE DO NOT USE ARRAYS IN THE CODE. There is also a 2nd picture of how the layout of the beginning of the code should look like. Please solve it correctly. Thank you!

Transcribed Image Text:You are developing a program to calculate the average overall scores for two divers in a diving competition. The competition involves five judges, each from a different country, who award scores ranging from 0 to 10. The program includes the following functions:
1. **getScore**: This function takes one double reference parameter. It prompts for the country’s name and the score given. If the score is invalid (i.e., not between 0 and 10), it prompts again. The function returns the country name and the score using a reference parameter.
2. **findSmallest**: This function takes five double parameters and returns the smallest score.
3. **findLargest**: This function takes five double parameters and returns the largest score.
4. **getAverage**: This function accepts five double parameters. The lowest and highest scores are discarded, and the remaining three are averaged. This is done by summing all five scores, subtracting the smallest and largest scores, and then averaging the remaining scores. The result is returned.
5. **scoreDive**: This function calculates the average overall score for a single diver. It calls getScore five times, prints each country’s name along with the score, calls getAverage with the five scores, and returns the average.
In the main function, `scoreDive` is called twice—once for diver 1 and once for diver 2. The final score for each diver is then printed.

Transcribed Image Text:```cpp
#include <iostream>
#include <fstream>
using namespace std;
double scoreDive();
int main()
{
double firstDiver = scoreDive();
double secondDiver = scoreDive();
if (firstDiver > secondDiver)
{
cout << "First Diver won with a score of " << firstDiver << endl;
}
else
{
cout << "Second Diver won with a score of " << secondDiver << endl;
}
}
double scoreDive()
{
// Write function here!
// Call the getScore function 5 times.
// Return the average score for this diver
return 0.0;
}
```
### Explanation:
This C++ program is designed to compare the scores of two divers and declare the winner based on their average scores. Key aspects include:
1. **Headers**: The program includes the `<iostream>` and `<fstream>` headers, enabling input-output stream functionalities.
2. **Namespace**: The `std` namespace is used, so you can use elements like `cout` without prefixing with `std::`.
3. **Function Declaration**:
- `double scoreDive();` is a function prototype, indicating that this function calculates scores for a diver.
4. **Main Function**:
- Calls `scoreDive()` to get scores for `firstDiver` and `secondDiver`.
- Uses an `if-else` statement to compare the scores and outputs which diver won along with their score.
5. **scoreDive Function**:
- Contains placeholder comments indicating where to implement the logic for:
- Calling a `getScore` function five times.
- Calculating and returning the average score for this diver.
- Currently returns `0.0` as a stub.
The program logic needs completion in the `scoreDive()` function to calculate real scores for meaningful output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
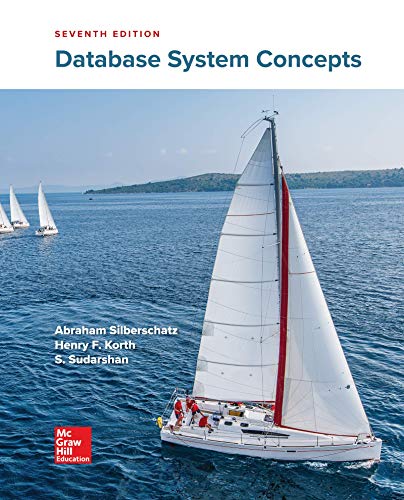
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
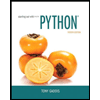
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
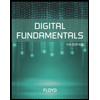
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
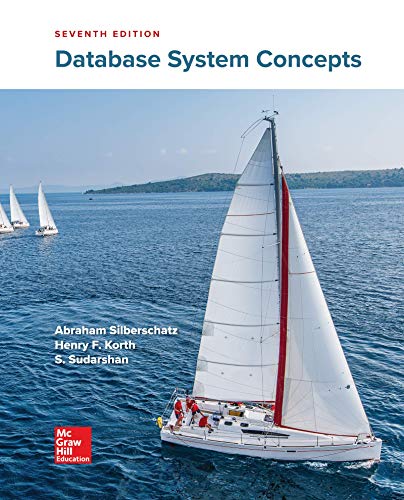
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
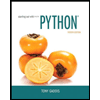
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
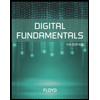
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
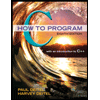
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
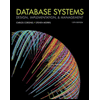
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
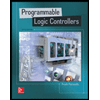
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education