You are working on a text mining project where you have to do some operations on text. Develop a C++ console application that does the following: Prompt for and get two strings from the user (each string has more than 10 characters). Print the length of each string. Convert the 5th character of the first string to an uppercase character and print it. Convert the 10th character of the second string to a lowercase character and print it. Output values should be printed with left and right justified label Look at the sample input and output and check your console with two different inputs.
You are working on a text mining project where you have to do some operations on text. Develop a C++ console application that does the following:
- Prompt for and get two strings from the user (each string has more than 10 characters).
- Print the length of each string.
- Convert the 5th character of the first string to an uppercase character and print it.
- Convert the 10th character of the second string to a lowercase character and print it.
- Output values should be printed with left and right justified label
- Look at the sample input and output and check your console with two different inputs.

Below is the required C++ program: -
Explanation: -
- Importing the essential headers and namespace.
- Defining the main function.
- Declaring the variables.
- Prompts the user to enter two strings.
- Using the while-loop that continuously ask the user to enter the strings with more than 10 characters.
- Displaying the length of both strings.
- Using the two for-loop to iterate over the both strings and displaying the 5th character of first string as uppercase and 10th character of second string as lowercase letter.
Code: -
//headers
#include <iostream>
#include <iomanip>
#include <string.h>
//namespace
using namespace std;
//Defining the main function
int main()
{
//Declaring the variables
string str1; //to store first string
string str2; //to store second string
//Prompts the user to enter two strings
cout<<"Enter two strings with more than 10 characters:\n";
//Storing the strings
cin>>str1>>str2;
//continuously ask the user to enter the string with more than 10 characters
while(!(str1.length() > 10 && str2.length() > 10))
{
cout<<"Please enter the both strings with more than 10 characters:\n"<<endl;
//Storing the strings
cin>>str1>>str2;
}
//Displaying the length of both strings
cout<<"The length of both string are: "<<left<<str1.length()<<setw(10)<<right<<str2.length()<<endl;
//cout<<left<<setw(10)<<"The length of second string is: "<<str2.length()<<endl;
//Using the first for-loop to iterate over the first string
for(int i = 0; i<str1.length(); i++)
{
//for the character stored at 4 index(5th character)
if(i==4)
{
//Displaying the 5th character as uppercase
cout<<"The fifth character as uppercase is: "<<left<<setw(10)<<(char)toupper(str1[i])<<right<<endl;
}
}
for(int i = 0; i<str2.length(); i++)
{
//for the character stored at 9 index(10th character)
if(i==9)
{
//Displaying the 10th character as lowercase
cout<<"The tenth character as lowercase is: "<<left<<setw(10)<<(char)tolower(str2[i])<<right<<endl;
}
}
}
Step by step
Solved in 3 steps with 1 images

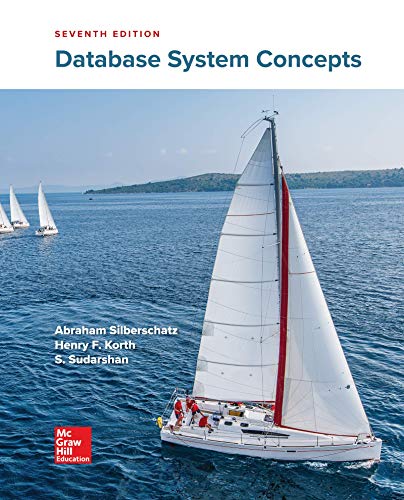
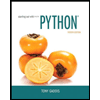
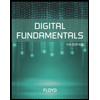
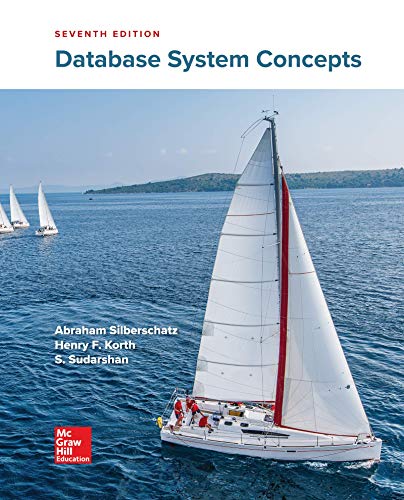
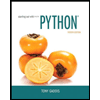
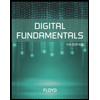
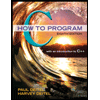
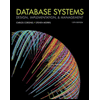
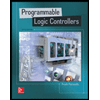