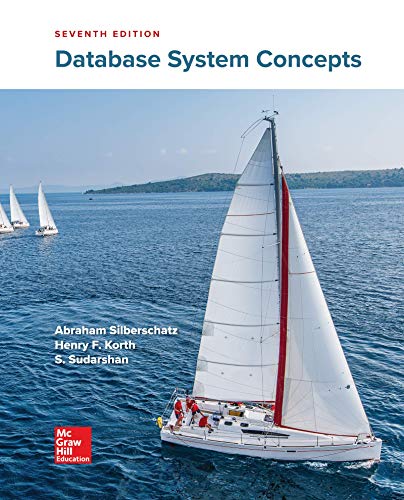
You are tasked with writing a program to process students’ end-of-semester marks. For each student you must enter into your program the student’s id number (an integer), followed by their mark (an integer out of 100) in 4 courses. A student number of 0 indicates the end of the data. A student moves on to the next semester if the average of their 4 courses is at least 60 (greater than or equal to 60).
Write a program to read the data and print, for each student:
The student number, the marks obtained in each course, final mark of the student (average of all the marks), their final grade (A,B,C,D or F) and whether or not they move on to the next semester.
In addition, after all data entry is completed, print
- The number of students who move on to the next semester and the number who do not.
- The student with the highest final mark (ignore the possibility of a tie).
Grades are calculated as follows:
A = 70 and over, B = 60 (inclusive) -70, C = 50(inclusive)-60
D = 40(inclusive)-50, F = less than 40
Hi please write this program in C#.
Let me know if you have any problem. Thank you

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- H Step 6: Descriptive Statistics: Points Scored By Your Time in Home Games The management of your team wants you to run descriptive statistics on the points scored by your team in the games played at your team's venue i 2015. Calculate descriptive statistics including the mean, median, variance, and standard deviation for points scored by your team played at Home. following edits to the code block below: 1. Replace ??MEAN_FUNCTION?? with the name of Python function that calculates the mean. 2. Replace ??MEDIAN_FUNCTION?? with the name of Python function that calculates the median. 3. Replace ??VAR_FUNCTION?? with the name of Python function that calculates the variance. 4. Replace ??STD_FUNCTION?? with the name of Python function that calculates the standard deviation. After you are done with your edits, click the block of code below and hit the Run button above. print("Points Scored by Your Team in Home Games (2013 to 2015)") print(" ") your_team_home_df =…arrow_forwardIn this assignment you will be responsible for writing several string validation and manipulationfunctions. The assumption will be that you are writing functions that will take input from a form andmanipulate or validate the information entered before it is processed into a database. A database is acollection of information but the information in the database must always be entered in a specificformat. Your responsibility will be to take data entered into a program and be sure it is writtencorrectly before it is entered into the database.Each function will have to have a specific name and heading. Each function will also need to includea docstring, the correct logic, and the proper return. Each function will be given a specific set ofpreconditions and postconditions/returns. A precondition is something you may assume to be truewhen the function is executed. A post condition is something you need to be sure is completed whenthe function is executed. Be sure to test each one of your…arrow_forwardPYTHON PROGRAMMING LANGUAGE Create a new text document called names.txt (this is done by hand - not using programming) with the following names: Adam, Bryan, Charlie. Each name should be followed by a hard return (do not store them in a list). Main Function. Write a program where the user enters a name. Using the read function, check to see if the name is in the text document. If it is, respond back to the user the name is found within the list. Read Function. The read function should return the contents of the text document as a list. Write Function. The write function should take the list and override the file with the new names list in reverse alphabetical order with a hard return after each name. EXAMPLEPlease enter a name: AdamAdam is found in the list.Enter another name (Y/N): YPlease enter a name: AndrewSorry, Andrew was not found in the list. Would you like to add it? (Y/N): YAndrew has been added to the list.Enter another name (Y/N): N LIST EXAMPLEadambryancharlie Final…arrow_forward
- Create a function named fnTuition that calculates the tuition for a student. This function accepts one parameter, the student ID, and it calls the fnStudentUnits function that you created in task 2. The tuition value for the student calculated according to the following pseudocode: if (student does not exist) or (student units = 0) tuition = 0 else if (student units >= 9) tuition = (full time cost) + (student units) * (per unit cost) else tuition = (part time cost) + (student units) * (per unit cost) Retrieve values of FullTimeCost, PartTimeCost, and PerUnitCost from table Tuition. If there is no student with the ID passed to the function, the function should return -1. Code two tests: 1) a student who has < 9 student units, and 2) for a student who has >= 9 student units. For each test, display StudentID and the result returned by the function. Also, run supportive SELECT query or queries that prove the results to be correct.arrow_forwardLe Chef Heureux Restaurant has 20 tables that can be reserved at 5 p.m., 7 p.m., or 9 p.m.Design a program that accepts reservations for specific tables at specific times; the user entersthe number of customers, the table number, and the time. Do not allow more than four guests pertable or invalid table numbers or times. If an attempt is made to reserve a table already taken,reprompt the user. Continue to accept reservations until the user enters a sentinel value or allslots are filled. Then display all empty tables in each time slot. Pseudocode and flowchart (RAPTOR please) if possible. Do not write it in any particular language, that is not required.arrow_forwardWrite a split_check function that returns the amount that each diner must pay to cover the cost of the meal. The function has four parameters: . bill: The amount of the bill. people: The number of diners to split the bill between. tax_percentage: The extra tax percentage to add to the bill. tip_percentage: The extra tip percentage to add to the bill. ● ● The tax or tip percentages are optional and may not be given when calling split_check. Use default parameter values of 0.15 (15%) for tip_percentage, and 0.09 (9%) for tax_percentage. Assume that the tip is calculated from the amount of the bill before tax. Sample output with inputs: 25 2 Cost per diner: $15.50 Sample output with inputs: 100 2 0.075 0.21 Cost per diner: $64.25 Learn how our autograder works 461710.3116374.qx3zqy7 1 # FIXME: Write the split_check function. HINT: Calculate the amount of tip and tax, 2 # add to the bill total, then divide by the number of diners. 3 4 Your solution goes here''' 5 6 bill float(input()) 7…arrow_forward
- Python code Screenshot and output is mustarrow_forwardIn C languagearrow_forwardTask 2 Use function to calculate Computer Skills. Both skills Yes: show "Sufficient", only one skill: show "Good", no skill: show "Poor" Computer Skills Student ID 521370 811298 780223 528112 427747 207676 158877 154194 480967 962948 701444 803700 268544 975048 204401 846716 127466 621984 285594 826758 972888 739477 857713 339297 249521 868320 872950 198271 702356 726662 365857 136850 492928 850557 987114 776815 666031 904552 161237 268716 Software Skill Yes Yes Yes No Yes Yes No Yes No No Yes No No Yes Yes No No No Yes Yes Yes Yes Yes Yes Yes Yes No Yes No Yes No Yes No Yes No Yes No Yes Yes Yes Hardware Skill No Yes Yes No Yes Yes No No Yes No Yes No No No No Yes No No No No Yes Yes Yes Yes No No Yes No Yes No Yes Yes | No Yes Yes Yes No Yes Yes Yesarrow_forward
- Write the function truck_adjustment_factor(truck_proportion, terrain_type) that takes the proportion of trucks using the motorway and the terrain type as inputs and returns the adjustment factor that is used for calculating the highway capacity. The adjustment factor is calculated according to the following formula: adju stment factor = tprupurtime x(furtor-1) Where proportion is the proportion of trucks and the factor is the car equivalent factor for the given terrain. You should be calling your car_equivalent_factor function to get the factor - don't reinvent the wheel! For example, for rolling terrain and a truck proportion equal to 0.12: adjustment factor = 1/(1 + 0.12 x (4.0 - 1)) = 0.735 Notes: You should include (and call/use) your car_equivalent_factor function in your answer o In general, you should paste in your whole module so far in each question as later functions will call earlier functions. • You can assume the function will always be called with one of the three valid…arrow_forwardPaytonarrow_forwardUsing Python: The credit plan at TidBit Computer Store specifies a 10% down payment and an annual interest rate of 12%. Monthly payments are 5% of the listed purchase price, minus the down payment. Write a program that takes the purchase price as input. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance × rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed. An example of the program input and output is shown below:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
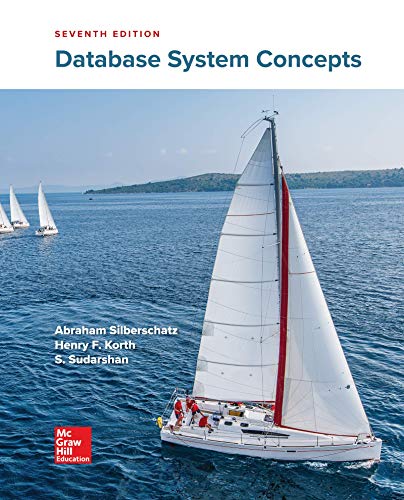
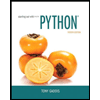
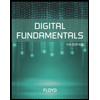
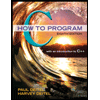
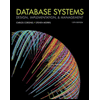
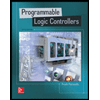