(You are only allowed to use the following functions/methods: print(), range(), len(), sum(), max(), min(), and .append()) You will be adjusting the Fibonacci Sequence code down below. You will be creating a Fibonacci Sequence where the numbers that are present in the sequence are generated using the rnd() function, including the first two digits in the sequence. For simplicity, we will assume your Fibonacci Sequence function will always produce a sequence with 10 digits. You will create a new function that takes all adjacent values from the sequence and finds their average value. The average values are then inserted into a list which will be the output of this code. For example, if we have the following sequence: [0, 1, 2, 3, 4, 5], the output of your averaging function will be [0.5, 1.5, 2.5, 3.5, 4.5]. def fibonacci_sequence(num_terms): # Initialize the first two terms of the sequence fib_seq = [0, 1] # Compute and append the next term for each term up to num_terms for i in range(2, num_terms): next_term = fib_seq[i-1] + fib_seq[i-2] fib_seq.append(next_term) # Display the resulting sequence print(fib_seq) # Test the function by computing and displaying the first 10 terms of the sequence fibonacci_sequence(10)
(You are only allowed to use the following functions/methods: print(),
range(), len(), sum(), max(), min(), and .append())
You will be adjusting the Fibonacci Sequence code down below. You will be creating a Fibonacci Sequence where the numbers that are present in the sequence are generated using the rnd() function, including the
first two digits in the sequence. For simplicity, we will assume your Fibonacci
Sequence function will always produce a sequence with 10 digits.
You will create a new function that takes all adjacent values from the sequence and
finds their average value. The average values are then inserted into a list which will
be the output of this code. For example, if we have the following sequence:
[0, 1, 2, 3, 4, 5], the output of your averaging function will be
[0.5, 1.5, 2.5, 3.5, 4.5].
def fibonacci_sequence(num_terms):
# Initialize the first two terms of the sequence
fib_seq = [0, 1]
# Compute and append the next term for each term up to num_terms
for i in range(2, num_terms):
next_term = fib_seq[i-1] + fib_seq[i-2]
fib_seq.append(next_term)
# Display the resulting sequence
print(fib_seq)
# Test the function by computing and displaying the first 10 terms of the sequence
fibonacci_sequence(10)

Step by step
Solved in 4 steps with 2 images

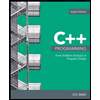
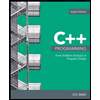