You are given a file which has an Australian state name and a university name in each line. You can view the file as follows. DO NOT CHANGE the content of the uni.txt (otherwise you will have to press the triple dot button at the top-right and then "Reset to Scaffold"). sorter.py uni.txt + uni.txt 1 NSW USYD 2 NSW UNSW 3 ACT ANU 4 WA UWA 5 QLD UQ 6 VIC UMEL 7 VIC MONASH_U 8 SA ADELAIDE_U Write a program sorter.py that 1) reads a command line argument containing the file to open, 2) opens and reads the file, 3) extracts each Australian state (not the university), i.e. the first word token in each line, and 4) sorts the states in alphabetical order. You may assume that each state is one word long. Furthermore, make sure to only record the names of unique states - so do not record any state twice. HINT: use the in keyword. E.g. ▸ Run 1 ls = [1, 2 if 4 in ls: 3 4 elif 4 not in ls: 2, 3] print("4 is in list") 5 print("4 is NOT in list") PYTHON You MUST use open(), read(), split(), sort(), print() methods, and for loops. Make sure you handle the case where the file cannot be found (use a try ... except block)! *In order to sort the list, you can use list.sort() method. For example, the list items can be sorted by using the following codes: ► Run 1 names = ['caren', 'annie', 'henry'] 2 #listname.sort() 3 names.sort() 4 print(names) PYTHON Your program should output the following. 1) When the file (e.g. uni.txt) is found: [user@sahara ~]$ python3 sorter.py uni.txt ['ACT', 'NSW', 'QLD', 'SA', 'VIC', 'WA'] 2) When the file (e.g. does_not_exist.txt) is NOT found: [user@sahara ~]$ python3 sorter.py does_not_exist.txt There is no such file
You are given a file which has an Australian state name and a university name in each line. You can view the file as follows. DO NOT CHANGE the content of the uni.txt (otherwise you will have to press the triple dot button at the top-right and then "Reset to Scaffold"). sorter.py uni.txt + uni.txt 1 NSW USYD 2 NSW UNSW 3 ACT ANU 4 WA UWA 5 QLD UQ 6 VIC UMEL 7 VIC MONASH_U 8 SA ADELAIDE_U Write a program sorter.py that 1) reads a command line argument containing the file to open, 2) opens and reads the file, 3) extracts each Australian state (not the university), i.e. the first word token in each line, and 4) sorts the states in alphabetical order. You may assume that each state is one word long. Furthermore, make sure to only record the names of unique states - so do not record any state twice. HINT: use the in keyword. E.g. ▸ Run 1 ls = [1, 2 if 4 in ls: 3 4 elif 4 not in ls: 2, 3] print("4 is in list") 5 print("4 is NOT in list") PYTHON You MUST use open(), read(), split(), sort(), print() methods, and for loops. Make sure you handle the case where the file cannot be found (use a try ... except block)! *In order to sort the list, you can use list.sort() method. For example, the list items can be sorted by using the following codes: ► Run 1 names = ['caren', 'annie', 'henry'] 2 #listname.sort() 3 names.sort() 4 print(names) PYTHON Your program should output the following. 1) When the file (e.g. uni.txt) is found: [user@sahara ~]$ python3 sorter.py uni.txt ['ACT', 'NSW', 'QLD', 'SA', 'VIC', 'WA'] 2) When the file (e.g. does_not_exist.txt) is NOT found: [user@sahara ~]$ python3 sorter.py does_not_exist.txt There is no such file
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 1CP
Related questions
Question
100%
Please give correct code. Thanks
![You are given a file which has an Australian state name and a university name in each line.
You can view the file as follows. DO NOT CHANGE the content of the uni.txt (otherwise you
will have to press the triple dot button at the top-right and then "Reset to Scaffold").
sorter.py
uni.txt
+
uni.txt
1 NSW USYD
2 NSW UNSW
3 ACT ANU
4 WA UWA
5 QLD UQ
6 VIC UMEL
7 VIC MONASH_U
8 SA ADELAIDE_U
Write a program sorter.py that 1) reads a command line argument containing the file to
open, 2) opens and reads the file, 3) extracts each Australian state (not the university), i.e. the
first word token in each line, and 4) sorts the states in alphabetical order. You may assume
that each state is one word long. Furthermore, make sure to only record the names of unique
states - so do not record any state twice.
HINT: use the in keyword. E.g.
▸ Run
1 ls = [1,
2 if 4 in ls:
3
4 elif 4 not in ls:
2, 3]
print("4 is in list")
5
print("4 is NOT in list")
PYTHON
You MUST use open(), read(), split(), sort(), print() methods, and for loops.
Make sure you handle the case where the file cannot be found (use a try ... except block)!
*In order to sort the list, you can use list.sort() method. For example, the list items can be
sorted by using the following codes:
► Run
1 names = ['caren', 'annie', 'henry']
2 #listname.sort()
3 names.sort()
4 print(names)
PYTHON
Your program should output the following.
1) When the file (e.g. uni.txt) is found:
[user@sahara ~]$ python3 sorter.py uni.txt
['ACT', 'NSW', 'QLD', 'SA', 'VIC', 'WA']
2) When the file (e.g. does_not_exist.txt) is NOT found:
[user@sahara ~]$ python3 sorter.py does_not_exist.txt
There is no such file](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb4d9d51c-cee3-4855-8494-55e8cc009ab5%2Fc288032f-67c6-4929-916c-467040fd6e52%2Ftrmgwus_processed.png&w=3840&q=75)
Transcribed Image Text:You are given a file which has an Australian state name and a university name in each line.
You can view the file as follows. DO NOT CHANGE the content of the uni.txt (otherwise you
will have to press the triple dot button at the top-right and then "Reset to Scaffold").
sorter.py
uni.txt
+
uni.txt
1 NSW USYD
2 NSW UNSW
3 ACT ANU
4 WA UWA
5 QLD UQ
6 VIC UMEL
7 VIC MONASH_U
8 SA ADELAIDE_U
Write a program sorter.py that 1) reads a command line argument containing the file to
open, 2) opens and reads the file, 3) extracts each Australian state (not the university), i.e. the
first word token in each line, and 4) sorts the states in alphabetical order. You may assume
that each state is one word long. Furthermore, make sure to only record the names of unique
states - so do not record any state twice.
HINT: use the in keyword. E.g.
▸ Run
1 ls = [1,
2 if 4 in ls:
3
4 elif 4 not in ls:
2, 3]
print("4 is in list")
5
print("4 is NOT in list")
PYTHON
You MUST use open(), read(), split(), sort(), print() methods, and for loops.
Make sure you handle the case where the file cannot be found (use a try ... except block)!
*In order to sort the list, you can use list.sort() method. For example, the list items can be
sorted by using the following codes:
► Run
1 names = ['caren', 'annie', 'henry']
2 #listname.sort()
3 names.sort()
4 print(names)
PYTHON
Your program should output the following.
1) When the file (e.g. uni.txt) is found:
[user@sahara ~]$ python3 sorter.py uni.txt
['ACT', 'NSW', 'QLD', 'SA', 'VIC', 'WA']
2) When the file (e.g. does_not_exist.txt) is NOT found:
[user@sahara ~]$ python3 sorter.py does_not_exist.txt
There is no such file
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 1 steps with 1 images

Recommended textbooks for you
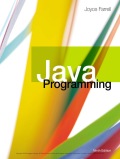
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
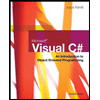
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
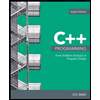
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
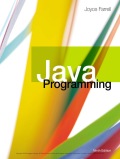
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
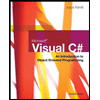
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
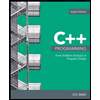
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning