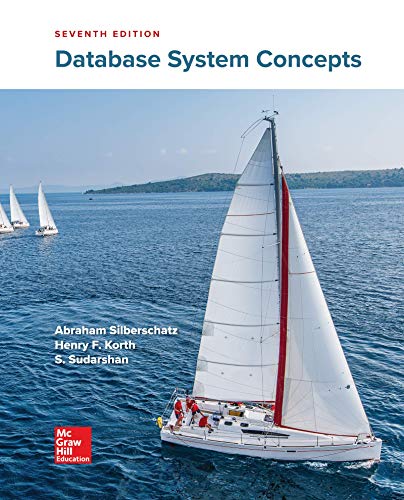
Problem 3 In HW6, you wrote a C program that performs state-space search for the 15-Puzzle similar in principle to those used in game engines. This search consists of a main loop that operates on two major lists open and
closed and a small list, successors. Write a C program that performs A* search using open, closed, succ, expand(node), filter(succ, list), merge(lst1,lst2). Write the main loop and merge(). DO NOT write filter() and expand(). Again, use the same
structure used in the homework. Assume the values used for node selection are computed and insertion functions listed
below are available:
struct node *insert_by_h(node,lst),*insert_by_g(node,lst),*insert_by_f(node,lst); /* insert a node to list */
struct node {
int loc[N+1][N]; /* the last row holds the three values used for node selection */
struct node *next;
} *start,*goal;
struct node *initialize(),*expand(),*merge(),*filter();
int main(int argc,char **argv) {
struct node *tsucc,*csucc,*copen,*topen,*open,*closed,*succ;
open=closed=succ=NULL;
start=initialize(argc,argv); /* init initial and goal states */
open=start;
code here
}
/* you may use any and all of the insert functons listed above */
struct node *merge(struct node *succ,struct node *open,int flag) {
struct node *csucc,*copen;
code here
return open;
}

Step by stepSolved in 3 steps

- Using c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardFor the given question use C language (DO NOT USE C++ OR JAVA). Write a complete C program to build an unordered Linked List with exactly 4 nodes, which contains randomly generated integer data. Program should also display all the data in the linked list by looping. Example : Start 15 8 24 17arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
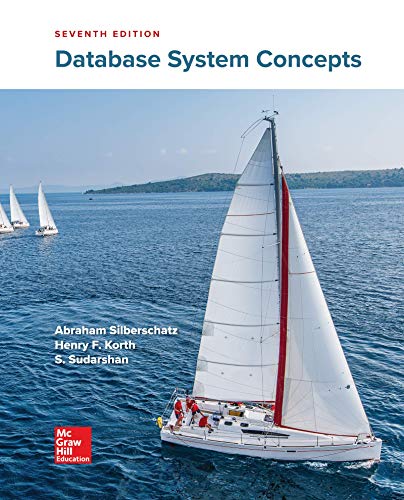
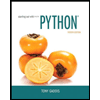
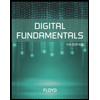
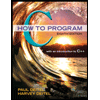
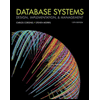
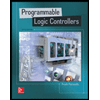