Write two local classes named Pipe and Ellipsoid Container as described below. Then, write a main function that reads the flowSpeed and area of a Pipe object (p) both as double values. main will also read the axisA, the axisB, and the axisC of a Ellipsoid Container object (wingTank) all as double values. Finally, the inputPipe of wingTank will be the p Pipe object. Finally, the program should calculate the time it takes the wingTank to be completely filled with gas and prints it to the screen as a double value. Pipe Class • A private double field named "flowSpeed" representing the flow speed of the pipe. • A private double field named "area" representing the cross-section area (i.e., kesit alan) of the pipe. • A constructor that gets two double values and initializes the object. • An empty constructor. A public function named calculate Flow Rate that does not get any parameter, multiplies the flowSpeed and area of the pipe, and returns it as a double value. Ellipsoid Container Class • A private double field named "axisA" representing the A axis length of the ellipsoid. • A private double field named "axisB" representing the B axis length of the ellipsoid. • A private double field named "axisC" representing the C axis length of the ellipsoid. • A private Pipe field named "inputPipe" representing the pipe that provides water to this EllipsoidContainer. • A constructor that gets three double values, a Pipe value, and initializes the object. A public function named calculateVolume that does not get any parameter, calculates the volume of the pool using the ellipsoid volume formula given below and returns it as a double value: Volume = (4/3)*PI*axisA axisB * axisA PIL • A public function named calculateTimeToFill that does not get any parameter, calculates .* . ★ CH
Write two local classes named Pipe and Ellipsoid Container as described below. Then, write a main function that reads the flowSpeed and area of a Pipe object (p) both as double values. main will also read the axisA, the axisB, and the axisC of a Ellipsoid Container object (wingTank) all as double values. Finally, the inputPipe of wingTank will be the p Pipe object. Finally, the program should calculate the time it takes the wingTank to be completely filled with gas and prints it to the screen as a double value. Pipe Class • A private double field named "flowSpeed" representing the flow speed of the pipe. • A private double field named "area" representing the cross-section area (i.e., kesit alan) of the pipe. • A constructor that gets two double values and initializes the object. • An empty constructor. A public function named calculate Flow Rate that does not get any parameter, multiplies the flowSpeed and area of the pipe, and returns it as a double value. Ellipsoid Container Class • A private double field named "axisA" representing the A axis length of the ellipsoid. • A private double field named "axisB" representing the B axis length of the ellipsoid. • A private double field named "axisC" representing the C axis length of the ellipsoid. • A private Pipe field named "inputPipe" representing the pipe that provides water to this EllipsoidContainer. • A constructor that gets three double values, a Pipe value, and initializes the object. A public function named calculateVolume that does not get any parameter, calculates the volume of the pool using the ellipsoid volume formula given below and returns it as a double value: Volume = (4/3)*PI*axisA axisB * axisA PIL • A public function named calculateTimeToFill that does not get any parameter, calculates .* . ★ CH
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:e
Courses Groups Calendar Support BAU BAUGO
1
P1001 (1-3) Introduc...
Write two local classes named Pipe and Ellipsoid Container as described below. Then, write a main
function that reads the flowSpeed and area of a Pipe object (p) both as double values. main will also
read the axisA, the axisB, and the axisC of a Ellipsoid Container object (wingTank) all as double
values. Finally, the inputPipe of wingTank will be the p Pipe object. Finally, the program should
calculate the time it takes the wingTank to be completely filled with gas and prints it to the screen as a
double value.
Pipe Class
• A private double field named "flowSpeed" representing the flow speed of the pipe.
• A private double field named "area" representing the cross-section area (i.e., kesit alan)
of the pipe.
A constructor that gets two double values and initializes the object.
. An empty constructor.
• A public function named calculate Flow Rate that does not get any parameter, multiplies
the flowSpeed and area of the pipe, and returns it as a double value.
@
i
2
O
W
Overview
Ellipsoid Container Class
• A private double field named "axisA" representing the A axis length of the ellipsoid.
• A private double field named "axisB" representing the B axis length of the ellipsoid.
• A private double field named "axisC" representing the C axis length of the ellipsoid.
• A private Pipe field named "inputPipe" representing the pipe that provides water to this
EllipsoidContainer.
• A constructor that gets three double values, a Pipe value, and initializes the object.
• A public function named calculateVolume that does not get any parameter, calculates
the volume of the pool using the ellipsoid volume formula given below and returns it as a
double value:
^
PALL
3 #
• A public function named calculateTimeToFill that does not get any parameter, calculates
...
1
PA
**
E
Plans
Volume = (4/3)*PI*axisA axisB * axisA
437
++
4
Resources
CH
R
*
%
5
buel.itslearning.com
BAU Library
T
Arama yapın veya web sitesini girin
ܢܚ
Follow-up and reports
&
6
MacBook Pro
Y
1
7
U
8

Transcribed Image Text:its Home Courses Groups Calendar Support BAU BAUGO
CMP1001 (1-3) Introduc...
Overview
Plans
Resources
Follow-up and reports
• A private Pipe field named "inputPipe" representing the pipe that provides water to this
Ellipsoid Container.
• A constructor that gets three double values, a Pipe value, and initializes the object.
• A public function named calculateVolume that does not get any parameter, calculates
the volume of the pool using the ellipsoid volume formula given below and returns it as a
double value:
Volume (4/3)*PI*axisA * axisB * axisA
• A public function named calculateTimeToFill that does not get any parameter, calculates
the time it takes for the EllipsoidContainer to be filled with gas from the pipe by dividing
the volume of the Ellipsoid Container by the flow rate of the pipe and returns it as a
double value.
The main function will,
1. Instantiate one Pipe object called p and instantiate an EllipsoidContainer object called
wingTank as explained above.
2. Use the calculateTimeToFill method of the Ellipsoid Container class to print out the time it
takes to fill the Ellipsoid Container and print it out.
BAU Library
NOTE: flowSpeed and area will always be given as positive values.
NOTE: All the functions MUST only do the tasks described above, nothing else.
NOTE: Both classes MUST be written as described.
NOTE: To calculate Pl, you MUST use the M_PI constant from the cmath library.
Input
Output
1536128
53.6165
928710
130.318
351512
16.7552
MacBook Pro
Participants
DE
1.02.2
Closed
BAS:
Max. s
>>> Colla
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
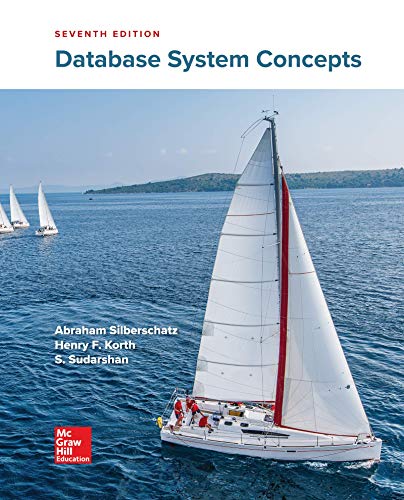
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
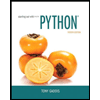
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
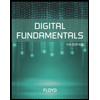
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
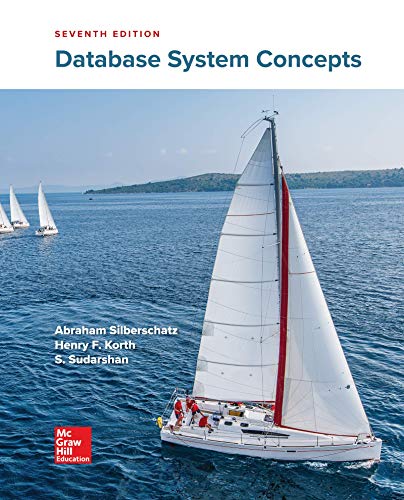
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
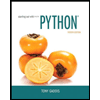
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
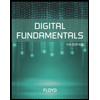
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
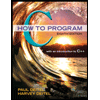
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
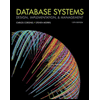
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
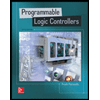
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education