Write the following three functions: a) The function gets an array A of length n of ints, and a boolean predicate pred. It returns the smallest index i such that pred(A[i])==true. If no such element is not found, the function returns -1. int find(int* A, int n, bool (*pred)(int)); b) The function gets an array A of length n of ints, and a function f. It applies f to each element of A. void map(int* A, int n, int (*f)(int)); c) The function gets an array A of length n of ints, and a function f. The function f gets 2 ints and works as follows: 1. Start with accumulator = A[0] 2. For i=1...length-1 compute accumulator=f(accumulator, A[i]) 3. Return accumulator For example, if f computes the sum of the two inputs, then reduce() will compute the sum of the entire array. int reduce(int* A, int n, int (*f)(int,int)); Test for the functions: // used for test Q3-find bool is_even(int x) { return x%2 == 0; } bool is_positive(int x) { return x>0; } void test_q3_find() { intA[6] = {-1,3,-6,5,2,7}; if (find(A, 6, is_even)==2 && find(A, 6, is_positive)==1) printf("Q3-find ok\n"); else printf("Q3-find ERROR\n"); } // used for test Q3-map int mult4(int x) { return x*4;} void test_q3_map() { intA[6] = {-1,3,-6,5,2,7}; map(A, 6, mult4); if (A[0]==-4 && A[3]==20) printf("Q3-map ok\n"); else printf("Q3-map ERROR\n"); } // used for test Q3-reduce int sum(int x, int y) {return x+y;} int last(int x, int y) {return y;} void test_q3_reduce() { intA[6] = {-1,3,-6,5,2,7}; if (reduce(A, 6, sum)==10 && reduce(A, 6, last)==7) printf("Q3-reduce ok\n"); else printf("Q3-reduce ERROR\n"); }
Write the following three functions:
a) The function gets an array A of length n of ints, and a boolean predicate pred.
It returns the smallest index i such that pred(A[i])==true.
If no such element is not found, the function returns -1.
int find(int* A, int n, bool (*pred)(int));
b) The function gets an array A of length n of ints, and a function f.
It applies f to each element of A.
void map(int* A, int n, int (*f)(int));
c) The function gets an array A of length n of ints, and a function f. The function f gets 2 ints
and works as follows:
1. Start with accumulator = A[0]
2. For i=1...length-1 compute accumulator=f(accumulator, A[i])
3. Return accumulator
For example, if f computes the sum of the two inputs, then reduce() will compute the sum of the
entire array.
int reduce(int* A, int n, int (*f)(int,int));
Test for the functions:

Step by step
Solved in 3 steps with 3 images

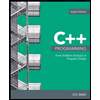
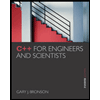
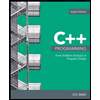
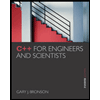