Write implementation of the isFull() method for the well developed ArrayStackADT
Q: Implement a CircularArray class that supports an array-like data structure which can be efficiently…
A: A circular Array is a linear data structure that can efficiently route. The array is circular when…
Q: *24.3 (IMPLEMENT A DOUBLY LINKED LIST) The MyLinkedList class used in Listing 24.5 is a one-way…
A: Linked list The most popular data structure for processing elements of dynamic data is a linked…
Q: Implement a method called appendTerm: static void appendTerm (SinglyLinkedList polynomial, Double…
A: Implementation of the method appendtTerm is shown below:- CODE:- int main(){ List *p;…
Q: What are the two ways to remove duplicates from ArrayList?
A: 1. LinkedHashSet In this method, we convert arraylist to set that doesn't allow duplicates and…
Q: Write a short Java method that takes an array of int values and determines if there is a pair of…
A: The following Java method checks to see if an array contains any separate members whose product is…
Q: Write a program in Java using ArrayList to remove duplicates in the array {0,1,4,5,8,0,9,4}.
A:
Q: How can you tell whether the performance of an ArrayList is satisfactory?
A: Hello student Greetings ArrayList is a commonly used data structure in Java programming that…
Q: When would you need an array with more than two dimensions
A: A matrix has two dimensions, which are represented by rows and columns, respectively. There are two…
Q: In java, i want to use forkjoin platform to execute 5 ArrayList to make it load faster
A: import java.io.*;import java.util.Iterator;import java.util.concurrent.CopyOnWriteArrayList; class…
Q: A string containing text and possibly delimiters has been split into tokens and stored in String[]…
A: Below answer is coded in Java language.
Q: Write a generic method filter() that receives two arrays (in and out) from any type that implements…
A: We will write code in Java. We have 3 parameters in filter() function : array in array out e…
Q: I need help writing a method that merges two arrays and prints the new array. I have written the…
A: The implemented code is described below. Here to merge two arrays in java use the List library and…
Q: Design and implement a method to return the index of the smallest value in in arr. Example 1: Input:…
A: The Given Program is implemented in Java: SOURCE CODE: 1. import java.util.*; 2. public class Main…
Q: Measure the performance of addFirst, remove(Object), and removeLast for each of the three…
A: In general, We see three implementations of the List interface in Java are Arraylist, Linkedlist,…
Q: Provide a static method that reverses the elements of a generic array list, without modifying the…
A: import java.util.*;class ArrayMethods{ private int[] values; //constructor to initialize values…
Q: In this assignment, you will implement a class calledArrayAndArrayList. This class includes some…
A: For the above program, we need to add the below mentioned functions or methods in java: ●…
Q: a Java method that takes in a generic ArrayList of Integers. Have the function compute the average…
A: Let's break down the Java method filterAboveAverage step by step:1) Method Signature:public static…
Q: three implementations, evaluate the performance of addFirst, remove(Object), and removeLast (Vectors…
A: For each of the three implementations, evaluate the performance of addFirst, remove(Object), and…
Q: Hello i need help with this code. this is the problem: In Listing 11.10, MyStack is implemented…
A: UML diagram for class ArrayList
Q: Write a method SortTheEvens(), that first shifts all the even numbers of an input array to the left…
A: Solution:-- 1)Given in the question is to find or shift all the even numbers on left side.…
Q: What is the process of creating a one-dimensional array?
A: What is the process of creating a one-dimensional array below step
Q: java using sets r maps Given an array of distinct integers, write a method called elementRank()…
A: Programs: Programs are used mainly for problem-solving. Any kind of complex problems can be solved…
Q: how to use Java to remove the specified number (num) of elements from the internal ArrayList of…
A: using java programming remove specific number of elements from array list and inserts the elements…
Q: PLEASE SIMPLIFY PLEASE!!!! truth table, simplified equation, and logical diagram. Show your…
A: Please refer below for your reference:
Q: Write the method printTail() method that accepts an array of integers and an integer index as…
A: Answer in step2
Q: Write a method, calcMax(int[8] intArray) that accepts an eight-element integer array as a parameter…
A: Program code: //define a class Maximum class Maximum { //define the method calcMax() static int…
Q: Write a method called samePosition in a class called stackExt that receives two parameters st1 and…
A: ArrayStack is a class which holds collection of Integer objects <Integer> wrapper class used…
Q: Write a method public static boolean insertAfter (int value, int target, ArrayList data) 1. seraches…
A: In JAVA, the ArrayList class is used to create a dynamic array for storing the elements that can…
Q: Provide a static method that checks whether a generic array list is a pal values at index i and n -…
A: import java.util.*;public class TestGeneric{public static void main(String…
Q: Create a Java program that will store 10 student objects in an ArrayList, ArrayList. A student…
A: The provided Java program meets the requirements of storing 10 student objects in an ArrayList and…
Q: Implement a method public static double[] add(double[] a, double[] b) for the component-wise sum…
A: Use a loop to iterate for the indices of an array and keep on assigning sum for an element in new…
Q: Implement a version of shellsort that keeps the increment sequence in an array, rather than…
A: Sure! Shellsort is a popular sorting algorithm that works by comparing and swapping elements that…
Q: Differentiate between add() and set() methods of arraylist
A: add() is used to insert the element
Q: I need to write a method in java. in this method i have a paragraph (string) field. the first thing…
A: Below is the explanation regarding the implementation of the method setPiecesSize:
Q: I have an ArrayList and I want to sort it by increasing string length. The ArrayList class has a…
A: Note-Since question asked for implementation of implementation of comparator interface to sort…
Q: rivacy l
A: public MyClass{private double[] _dataArray = new double[100]; public void setDataArray(double[]…
Q: Implement a recursive version of the size method for SinglyLinkedLists. (Hint: A wrapper may be…
A: Implementation of the recursive version of the size method for SinglyLinkedLists in Python: class…
Q: Write a Java method that will take in a generic ArrayList with Doubles (reference type). Have the…
A: import java.util.ArrayList; import java.util.Scanner; public class Doubles2IntegersInArrayList {…
Q: Explain array method splice() with suitable examples in JavaScript.
A: Introduction: For JavaScript Array objects, there is a built-in method called splice(). It allows…
Q: Main problem. Implement the class Array that imitates dynamic1 arrays of integers. Introduce the…
A: the class that i have created is Main.java and i have included all the methods with the output…
Q: What will be the value of element at index 2 of the alist after the following Java code is executed?…
A: PROGRAM APPROACH Importing necessary packages Declaring main class Declaring the main method…
Q: ment the complete stack functionality by using ArrayList which will contain Integer value
A: Below the java program which Implement the complete stack functionality by using ArrayList which…
Q: Design and implement a method to return the index of the smallest value in in arr. Example 1:…
A: required Method: public static int indexOfSmallest(int[] arr){ //if the array is empty…
Q: Write the same splice method for an ArrayList with member variables called data and size and methods…
A: The method splice is used to modify the contents of an arraylist , this has the following parameters…
Q: I need help with my RPN calculator. It keeps outputting null for every expression. Any help is…
A: The issue with the code is that the RPNStringTokenizer interface only has a static method, but it is…


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

- Calculate the performance of addFirst, remove(Object), and removeLast for each of the three implementations (Vectors may be included if desired). Which implementations do the best with short lists? Which implementations are the most efficient for huge lists?Hey, Please fill in the missing code needed for the three sum implementation in Java. Please provide code comments aswell. Thanks. Code required to fill in is in the do something area. import java.util.Arrays;import java.util.List;public class ThreeSum {private static final List<String> filePaths = new ArrayList<>();static {filePaths.add("resources/1Kints.txt");filePaths.add("resources/2Kints.txt");filePaths.add("resources/4Kints.txt");filePaths.add("resources/8Kints.txt");filePaths.add("resources/16Kints.txt");}public static void main(final String[] args) {for (final String path : filePaths) {// do something}}public static int threeSumA(final int[] array) {final int length = array.length;int count = 0;for (int i = 0; i < length; i++) {for (int j = i+1; j < length; j++) {for (int k = j + 1; k < length; k++) {if (array[i] + array[j] + array[k] == 0) {count++;}}}}return count;}public static int threeSumB(final int[] array) {final int length =…Develop classes STint and STdouble for maintaining ordered symbol tables where keys are primitive int and double types, respectively. (Convert genericsto primitive types in the code of RedBlackBST.) Test your solution with a version ofSparseVector as a client.
- Using java: Your task is to implement a class, IntegerSet, that contains only integers, given the specification below. public class IntegerSet { // Hint: probably best to use an array list. You will need to do a little research private List<Integer> set = new ArrayList<Integer>(); // Default Constructor public IntegerSet() { } // Clears the internal representation of the set public void clear() {…}; // Returns the length of the set public int length() {…}; // returns the length /* * Returns true if the 2 sets are equal, false otherwise; * Two sets are equal if they contain all of the same values in ANY order. */ public boolean equals(IntegerSet b) {…}; // Returns true if the set contains the value, otherwise false public boolean contains(int value) {…}; // Returns the largest item in the set; Throws a IntegerSetException if the set is empty public int largest()…Java Complete a method calledRedundantCharacterMatch(ArrayList<Character> YourFirstName): the parameter of this method is an ArrayList<Character> whose elements are the characters in your first name (they should be in the order appear in your first name, e.g., if your first name is bob, then the ArrayList<Char> includes ‘b’, ‘o’, ‘b’.). The method will check whether there exists duplicate characters in your name and return the index of those duplicate characters. For example, when using bob as first name, it will return b: 0, 2. 2. Create an ArrayList<Character> NameExample. All the characters of your first name will appear twice in this ArrayList. For example, if your first name is bob, then NameExample will include the following element {b,o,b,b,o,b}. Then, please use NameExample as parameter for the method RedundantCharacterMatch(). If your first name is bob, the results that print in the console will be b: 0, 2, 3, 5 o: 1, 4Compare and contrast the array and arrayList. Give at least one example that describe when to use arrayList compared to an array.
- Write a method called samePosition in a class called StackExt that receives two parameters stl and st2 of type ArrayStack and a third parameter pos of type int. The method returns true, if stack stl and st2 contains the same value in position pos. Otherwise, the method returns false. Assume that both stacks stl and st2 contain same number of elements. Assume that the position of the top element is O and increases by 1 for each subsequent element. The elements of both stacks stl and st2 must remain in the original relative positions in the stacks at the end of the method. Use common stack operations only such as push, pop, peek, isEmpty, constructor and copy constructor. You can create temporary objects of type ArrayStack in your method. Example: top 4 5 3 10 8 stl: stl: 5 9 4 10 1 If the pos is 3, then both stacks contain 10 in position 3. The method returns true. If the pos is 1, then st1 Contains 5 and st2 contains 9 in position 1. The method returns false. public class StackExt {…In java Write a method public static ArrayList<Integer> merge(ArrayList<Integer> a, ArrayList<Integer> b) that merges one array list with another. Ex: a = 1 2 3 4 5, b = 6 7 8 9 10 then merge returns 1 6 2 7 3 8 4 9 5 10Write a complete java program including filerNegative method that filters out all of the negative values from ArrayList. Then write a method minMax to return the value and the index for the minimum and the maximum values from the rest of numbers values. Suppose that you have the following list of integer values to be inserted to your ArrayList: (-1, 2, -4, -3, 0, 5, 9, 6, -7, 8).
- Question in java Arraylist Please help fastImplement substringList() that will produce the collection of words from the 1D array B that has substrings equivalent to the string key. The resulting collection should be stored in array C and this should contain only the unique set of words. The function returns the resulting total number of elements in array C. For your reference, a substring is a contiguous sequence of characters within a string. As an example, the strings “app”, “ppl”, “apple”, and “e” are among the substrings from the string “apple”. But, strings “ale” are “elppa” are not substrings from string “apple”. key = "or" array B = {"it's", "today", "now", "or", "forever", "today", "ACT"} array C should be {"or", "forever"} Rules: You can only use strcmp, strcat, and strcpy Maximize the use of loops (e.g., for) you can create local variables that can aid youThe "dynamic array" data structure is used as the basis of Java's ArrayList library class. Describe this data structure and explain how it achieves (amortized) constant-time adding of elements, while also giving constant-time random access of elements. As part of your answer, make sure to explain what "amortized" means for the data structure.
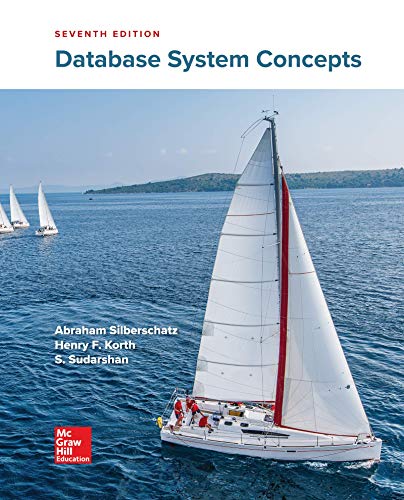
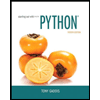
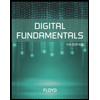
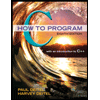
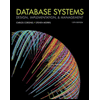
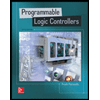
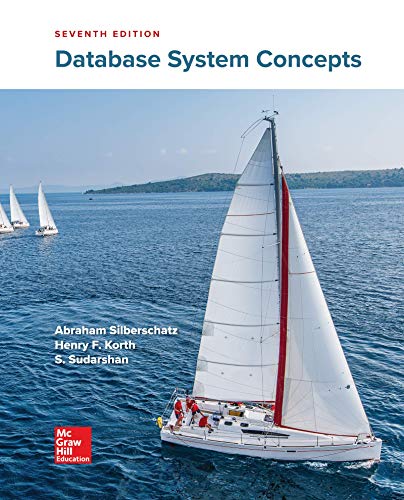
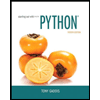
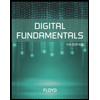
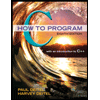
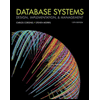
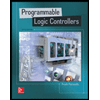