Write down a program to accept user’s First and Last Name. Print full name of the user. Print the length of the full name of the user excluding the white spaces. Print the length of the First Name and Last Name respectively.
Problem 1:
- Write down a
program to accept user’s First and Last Name. Print full name of the user. - Print the length of the full name of the user excluding the white spaces.
- Print the length of the First Name and Last Name respectively.
Problem 2:
Write down the program that displays your mailing address as it is generally printed in your area where you live.
You do not require to take any input from the user. Mailing address can be any hypothetical address.
Problem 3:
Accept the length and width of the room in floating-point and compute the area of the room. Print area of the room including the units e.g. inch, meters, cms, feet, etc.
Problem 4:
Write a program to print the sum of n positive integers (1 to n), where n is input by the user.
You can compute summation using following formula:
Problem 5:
Create a program that reads two integers, a and b, from the user. Your program should compute and display:
- The sum of a and b
- The difference when b is subtracted from a
- The product of a and b
- The division when a is divided by b
Problem 6:
Accept any string input from the user and return every 3rd character of the string from 2nd index.
Problem 7:
Define a variable with value as following string:
“How Giving Up Refined Sugar Changed My Brain”
Print 13 characters from 17th index in reversed order of the above reversed string. Your program should not contain more than 1 variable and string value of the variable should remain intact.

Step by step
Solved in 3 steps with 1 images

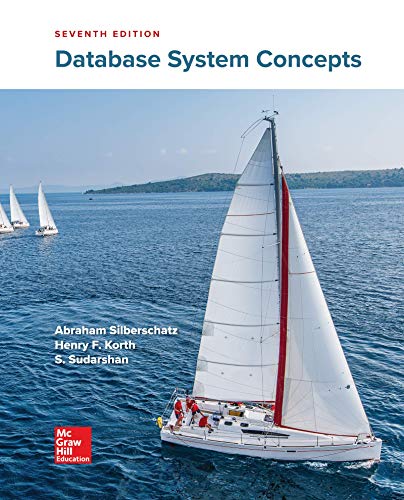
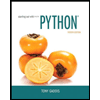
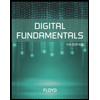
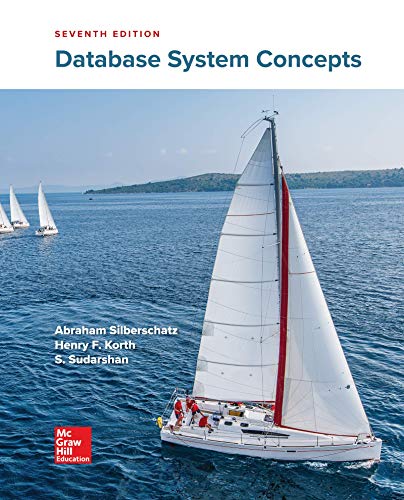
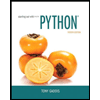
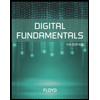
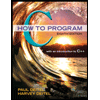
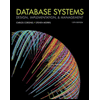
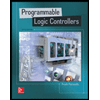