Write another member function (only) in the previously developed class of SpecialTime in question1, as timeMachine() that takes 02 integer parameters, direction and duration. The direction can have value of -1 or 1 representing going back in time or into the future, respectively. The duration parameter should contain number of minutes to travel in past or future. Add validation to enforce the value of direction as -1 or 1, and value of duration as positive value (number of minutes not exceeding 24 hours). The timeMachine function should return the time your land in (past or future) by adding the received duration into the hour, minute and seconds. Please note that your time machine can only travel upto 24 hours either to the past or to the future. so far my code for the question one is #include # include class time { int hr,min,sec; public: //don't forget it ! time() { hr=min=sec=0; cout<<" Time reset to 00:00:00"; } time(int h,int m,int s) { hr =h; min=m; sec=s; cout<<" Time set to specified value."; } void display() { cout<<" Time set is # "< 59) {sec -=60; min++;} min += t1.min +t2.min; //Note Operator Precedence if(min>59) {min-=60; hr++;} hr += t1.hr + t2.hr; // Excluding the possibility of hr being greater than // 24. We do not want digression...! } }; void main() { clrscr(); time a(12,11,33); time b(10,34,50); time c; a.display(); b.display(); c.add(a,b); c.display(); getch(); }
Write another member function (only) in the previously developed class of SpecialTime in question1, as timeMachine() that takes 02 integer parameters, direction and duration. The direction can have value of -1 or 1 representing going back in time or into the future, respectively. The duration parameter should contain number of minutes to travel in past or future. Add validation to enforce the value of direction as -1 or 1, and value of duration as positive value (number of minutes not exceeding 24 hours). The timeMachine function should return the time your land in (past or future) by adding the received duration into the hour, minute and seconds.
Please note that your time machine can only travel upto 24 hours either to the past or to the future.
so far my code for the question one is
#include<iostream.h>
# include<conio.h>
class time
{
int hr,min,sec;
public: //don't forget it !
time()
{
hr=min=sec=0;
cout<<"
Time reset to 00:00:00";
}
time(int h,int m,int s)
{
hr =h;
min=m;
sec=s;
cout<<"
Time set to specified value.";
}
void display()
{
cout<<"
Time set is # "<<hr<<":"<<min<<":"<<sec;
}
void add(time t1,time t2)
{
sec = t1.sec + t2.sec;
if (sec > 59)
{sec -=60; min++;}
min += t1.min +t2.min; //Note Operator Precedence
if(min>59)
{min-=60; hr++;}
hr += t1.hr + t2.hr;
// Excluding the possibility of hr being greater than
// 24. We do not want digression...!
}
};
void main()
{
clrscr();
time a(12,11,33);
time b(10,34,50);
time c;
a.display();
b.display();
c.add(a,b);
c.display();
getch();
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

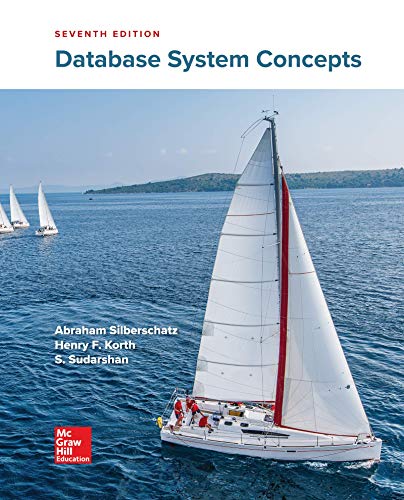
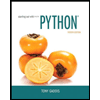
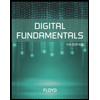
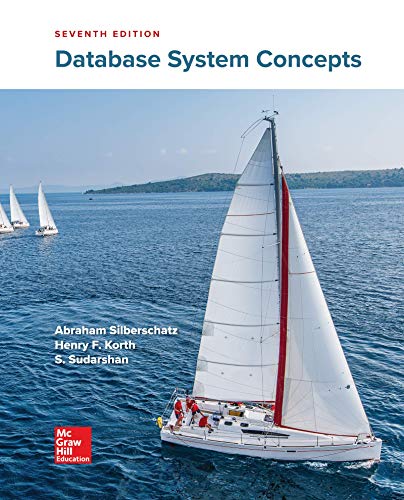
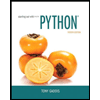
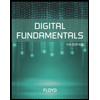
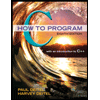
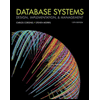
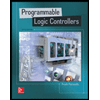