Write a static method that takes an array of a generic type as its only argument. The method should display the array and return the number of elements in the array. Test the method in main with at least three arrays of objects. SAMPLE OUTPUT Here is an Integer array 12 21 7 16 8 13 That array held 6 elements Here is a String array one two three four That array held 4 elements Here is a Double array 1.1 2.2 3.3 4.4 5.5 That array held 5 elements My code: package radosavljevic19part1; import java.util.ArrayList; import java.util.Arrays; public class CreatorOfArray { public static void main(String[]args) { //creating array in three different types Integer[]arr1= {12, 21, 7, 16, 8, 13}; String []arr2={"one", "two", "three", "four"}; Double[]arr3={1.1, 2.2, 3.3, 4.4, 5.5}; //display arrays System.out.println("Here is an integer array: "); printList(arr1); System.out.println("\nHere is a String array: "); printList(arr2); System.out.println("\nHere is a Double array: "); printList(arr3);
Write a static method that takes an array of a generic type as its only argument. The method should display the array and return the number of elements in the array. Test the method in main with at least three arrays of objects.
SAMPLE OUTPUT
package radosavljevic19part1;
import java.util.ArrayList;
import java.util.Arrays;
public class CreatorOfArray {
public static void main(String[]args) {
//creating array in three different types
Integer[]arr1= {12, 21, 7, 16, 8, 13};
String []arr2={"one", "two", "three", "four"};
Double[]arr3={1.1, 2.2, 3.3, 4.4, 5.5};
//display arrays
System.out.println("Here is an integer array: ");
printList(arr1);
System.out.println("\nHere is a String array: ");
printList(arr2);
System.out.println("\nHere is a Double array: ");
printList(arr3);
}
//generic method
public static <E> void printList (E[] list) {
for (int i=0;i<list.length;i++) {
System.out.println(list[i]);
System.out.println();
}
System.out.println("That array held "+list.length + " elements.");
}
}
Problem is does not print integers in the same line

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

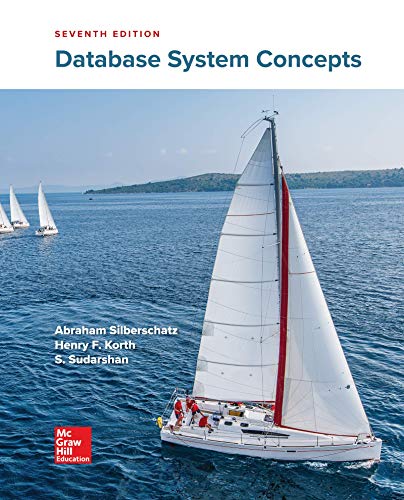
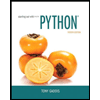
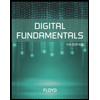
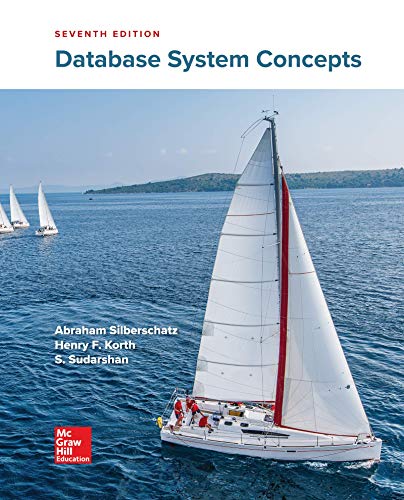
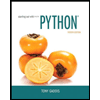
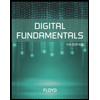
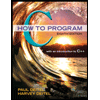
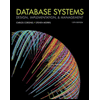
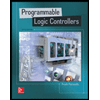