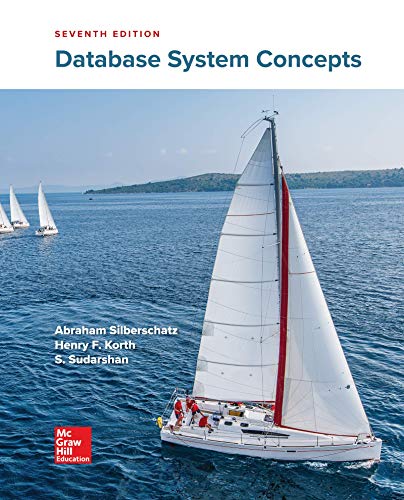
Write a recursive function that, given a sequence of comparable values, returns the count of elements where the current element is less than the following ( next ) element in the given sequence. See the examples given below.
def count_ordered ( seq ) :
"""
Input : A sequence of comparable elements
Output : The number of elements that are less than the following element in the sequence
Example :
>>> count_ordered ( [ 1 , 2 , 3 , 4 , 5 , 6 ] )
5
>>> count_ordered ( ( 1 , 12, 7.3 , -2,4 ) )
2
>>> count_ordered ( 'Python' )
2
>>> count_ordered ( [ 6 ] )
0
>>> count_ordered ( [ ] )
0
"""
In the first example above , count_ordered ( [ 1,2,3,4,5,6 ] )
the returned answer is 5 because for all the first 5 numbers the current number is less than the next number.
In the second example above, count_ordered ( ( 1,12,7.3 , -2,4 ) )
the returned answer is 2 because there are two cases where the current value is less than the next value, i.e. 1 is less than 12 and -2 is less than 4.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Write a function print_matching_indexes (items, target) that prints the indexes of all the occurrences of the target in the list items. Check the test cases for how the function should work. Note: Your answer must use a for loop. You are not allowed to use a while loop. For example: Test Result nums = [10, 20, 30] 1 print_matching_indexes (nums, 20) pets = ['dog', 'cat', 'fish', 'cat', 'dog', 'iguana'] 1 print_matching_indexes (pets, 'cat') 3 empty = [] print_matching_indexes (empty, 42)arrow_forwardPython. Please solve quickly!!arrow_forwardModify the recursive Fibonacci function to employ the memoization technique discussed in this chapter. The function creates a dictionary and then defines a nested recursive helper function named memoizedFib. You will need to create a dictionary to cache the sum of the fib function. The base case of the fib function is the same as before. However, before making any recursive calls, the helper function looks up the value for the function’s current argument in the dictionary (use the method get, with None as the default value). If the value exists, the function returns it and skips any recursive calls. Otherwise, after the helper function adds the results of its two recursive calls, it saves the sum in the dictionary with the current argument of the function as the key. Note: The program should output in the following format: n fib(n) 2 1 4 3 8 21 16 987 32 2178309 ---------------------------------------------------------------------------------- def…arrow_forward
- Write a recursive function that accepts a number and returns its factorial. b. Write a recursive function that accepts an array, its size and the index of the initial element as arguments. The function fills the array with the elements of the following sequence: n1 = 3, nk+1 = nk+35 c. Write an iterative and a recursive versions of the binary search. In C++ codingarrow_forwardPlease solve using C language The function get_tokens gets a string str, and a char delim, and returns the array with the tokens in the correct order. The length of the array should be the number of tokens, computed in count_tokens. char** get_tokens(const char* str, char delim); For example: ● get_tokens("abc-EFG--", '-') needs to return ["abc","EFG"] ● get_tokens("++a+b+c", '+') needs to return ["a","b","c"]. ● get_tokens("***", '*') needs to return either NULL or an empty array. Note that the returned array and the strings in it must all be dynamically allocated.arrow_forward1. The sorted values array contains 16 integers 5, 7, 10, 13, 13, 20, 21, 25, 30,32, 40, 45, 50, 52, 57, 60. Indicate the sequence of recursive calls that are made tobinaraySearch, given an initial invocation of binarySearch(32, 0, 15).show only the recursive calls. For example, initial invocation is binarySearch(45,0,15)where the target is 45, first is 0 and last is 15.arrow_forward
- please solve in python and give code. thankzzarrow_forwardC++arrow_forwardThe function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forward
- Write the in_order() function, which has a list of integers as a parameter, and returns True if the integers are sorted (in order from low to high) or False otherwise. The program outputs "In order" if the list is sorted, or "Not in order" if the list is not sorted. Ex: If the list passed to the in_order() function is [5, 6, 7, 8, 3], then the function returns False and the program outputs: Not in order Ex: If the list passed to the in_order() function is [5, 6, 7, 8, 10], then the function returns True and the program outputs: In order Note: Use a for loop. DO NOT use sorted() or sort(). python pleasearrow_forward///////1/////. Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Let me know answer. //. .arrow_forwardWrite a function little_sum (number_list, limit) that returns the sum of only the numbers in number_list that are less than or equal to the given limit. In this question you must use a for loop and you are not allowed to use the sum function. For example: Test Result numbers = [1, 2, 3, 4, 5, 6] total = little_sum(numbers, 3) print(total) values = [6, 3, 4, 1, 5, 2] 10 total = little_sum(values, 4) print(total)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
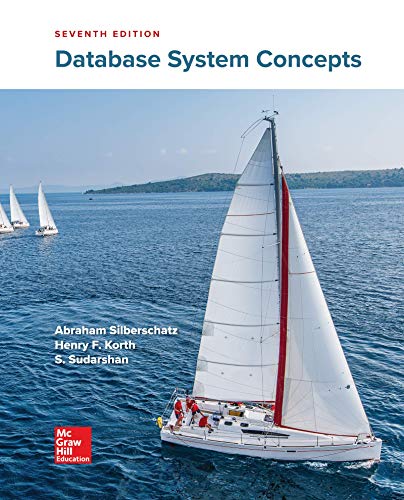
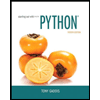
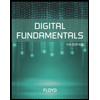
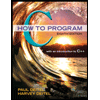
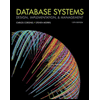
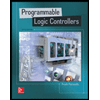