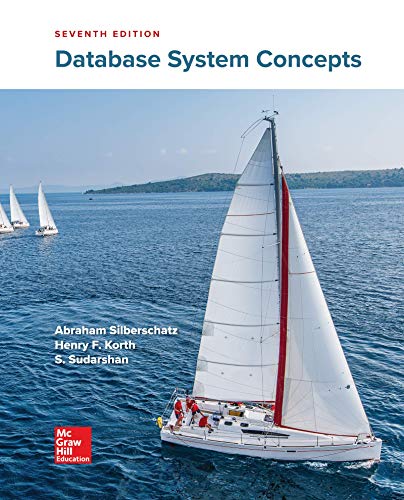
Write a Python
Specifications
• Call your program wordReverse.py
• Write a function called printText. This function is going to accept a list strings and print the string. This function will:
– Loop through the list one sentence at a time.
– For each sentence, reverse each word in the sentence.
– Once all the processing is done, print the text.
• In the main function
– Note that you will read a maximum of 25 rows and each row could contain 200 characters.
– Read in the text from the user. Each “value” of the list is a newline terminated string. Stop when the user enters a blank line.
– Call the printText function.
• Please comment your code appropriately.
• The text entered may contain stray whitespace.
Sample Run
Enter the text:
Life is but a day;
A fragile dew-drop on its perilous way
From a tree’s summit.
The reversed worded text is
efiL si tub a ;yad
A eligarf wed-pord no sti suolirep yaw
morF a s’eert .timmuy

Step by stepSolved in 4 steps with 1 images

- please code in pythonWrite a function that takes in a number and returns the sum of all primes less than or equal to that number. You'll need your answer to the previous problem to answer this.Example: sum_primes(11) == 2 + 3 + 5 + 7 + 11 == 28Bonus: Can you do this in one line with a list comprehension?arrow_forwardPython Write a function that takes as a parameter a list of strings and returns a list containing the lengths of each of the strings. That is, if the input parameter is ["apple pie", "brownies","chocolate","dulce de leche","eclairs"], your function should return [9, 8, 9, 14, 7]. The file you submit should include a main() function that demonstrates that your function works. The list that is the input argument can be a hard-coded one like the one shown above or you could ask the user to input a string and split on the appropriate delimiter, creating a list. Hint: This problem can be approached as an "accumulator", where we accumulate lists:arrow_forwardWrite a function that accepts two arguments, a list and a number n. The list contains numbers. The function return a list of all the numbers from the input list that are greater than number n. greater_than_n(list, n)arrow_forward
- please use your own code and just a picturearrow_forwardIn pythonarrow_forwarddo these coe in python: Suppose you have the following animal names in a list: cat, dog,mouse, hamster. Write a code that prints the first letter, the last letter, andthe length of each animal’s name. Print out all the animal names that havea length greater than 3. after that Write a program that counts the number of aletter that appears in the list (i.e. the frequency of the letter).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
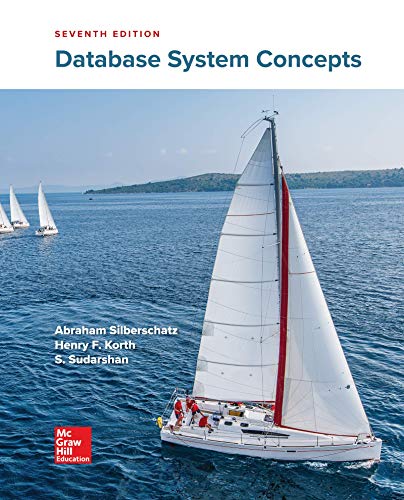
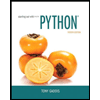
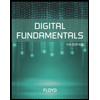
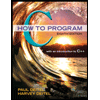
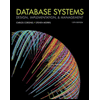
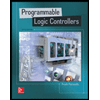