Write a program using C++ that has two functions with the same name (function overloading) to calculate the area of different shapes. The first function takes the radius of a circle as input and returns the area of the circle. The second function takes the length and width of a rectangle as input and returns the area of the rectangle. Your main function should declare three variables, one for the radius of a circle and two for the length and width of a rectangle, respectively. Initialize the variables with the following values: double radius = 5.0; double length = 10.0; double width = 8.0; Your program should call both functions with the respective input values and print the results to the console in the following format: The area of the circle with radius [radius] is: [area] The area of the rectangle with length [length] and width [width] is: [area] You can assume that the value of radius is non-negative and the values of length and width are positive. // Function to calculate the area of a circle given its radiusdouble calculateArea(double radius); // Function to calculate the area of a rectangle given its length and widthdouble calculateArea(double length, double width); SAMPLE OUTPUT: The area of the circle with radius 5 is: 78.5398 The area of the rectangle with length 10 and width 8 is: 80
Write a program using C++ that has two functions with the same name (function overloading) to calculate the area of different shapes.
- The first function takes the radius of a circle as input and returns the area of the circle.
- The second function takes the length and width of a rectangle as input and returns the area of the rectangle.
Your main function should declare three variables, one for the radius of a circle and two for the length and width of a rectangle, respectively. Initialize the variables with the following values:
double radius = 5.0;
double length = 10.0;
double width = 8.0;
Your program should call both functions with the respective input values and print the results to the console in the following format:
The area of the circle with radius [radius] is: [area]
The area of the rectangle with length [length] and width [width] is: [area]
You can assume that the value of radius is non-negative and the values of length and width are positive.
// Function to calculate the area of a circle given its radiusdouble calculateArea(double radius);
// Function to calculate the area of a rectangle given its length and widthdouble calculateArea(double length, double width);
SAMPLE OUTPUT:
The area of the circle with radius 5 is: 78.5398
The area of the rectangle with length 10 and width 8 is: 80

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

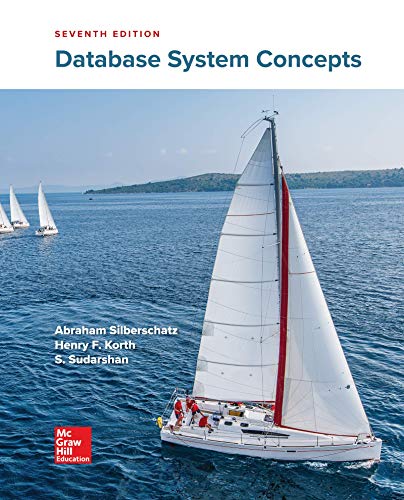
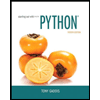
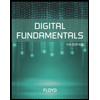
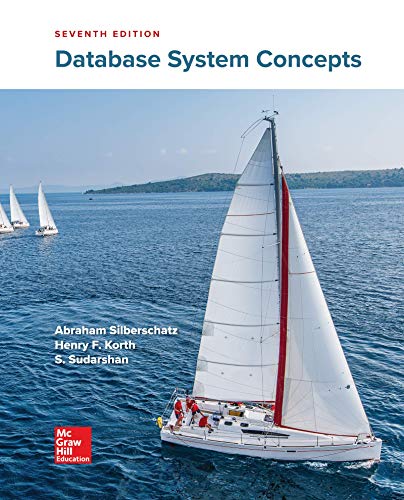
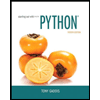
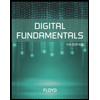
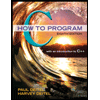
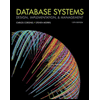
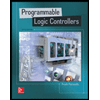