Write a Program with Comments for your code to improve quick sort. The quick sort algorithm presented in the ClassNotes-Sorting, it selects the first element in the list as the pivot. Revise it by selecting the median among the first, middle, and last elements in the list. Explain your Outputs or Answers for the following: (C) Give a list of n items (for example, an array of 10 integers) representing the worst-case scenario. (D) Give a list of n items (for example, an array of 10 integers) representing the best-case scenario.
Language: C++
Write a Program with Comments for your code to improve quick sort. The quick sort
Explain your Outputs or Answers for the following:
- (C) Give a list of n items (for example, an array of 10 integers) representing the worst-case scenario.
-
(D) Give a list of n items (for example, an array of 10 integers) representing the best-case scenario.
Please compile your answers in a file including all source code with comments, Explain your running Outputs or Answers in (A) ~(D).
Quicksort that was mentioned in the ClassNotes:
#include <iostream>
using namespace std;
// Function prototypes
void quickSort(int list[], int arraySize);
void quickSort(int list[], int first, int last);
int partition(int list[], int first, int last);
void quickSort(int list[], int arraySize)
{
quickSort(list, 0, arraySize - 1);
}
void quickSort(int list[], int first, int last)
{
if (last > first)
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
// Partition the array list[first..last]
int partition(int list[], int first, int last)
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
int high = last; // Index for backward search
while (high > low)
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
// Search backward from right
while (low <= high && list[high] > pivot)
high--;
// Swap two elements in the list
if (high > low)
{
int temp = list[high];
list[high] = list[low];
list[low] = temp;
}
}
while (high > first && list[high] >= pivot)
high--;
// Swap pivot with list[high]
if (pivot > list[high])
{
list[first] = list[high];
list[high] = pivot;
return high;
}
else
{
return first;
}
}
int main()
{
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort(list, SIZE);
for (int i = 0; i < SIZE; i++)
cout << list[i] << " ";
return 0;
}
![1
#include <iostream>
using namespace std;
3
// Function prototypes
void quickSort(int list[], int arraysize);
void quickSort (int list[), int first, int last);
int partition(int list[], int first, int last);
4
6.
7
8.
void quickSort(int list[], int arraysize)
{
quickSort (list, 0, arraysize - 1);
9
10
11
12
13
void quickSort (int list[], int first, int last)
15
14
16
if (last > first)
17
{
18
int pivotIndex - partition(list, first, last);
quickSort (list, first, pivotIndex - 1);
quickSort (list, pivotIndex + 1, 1last);
19
20
21
22
23
// Partition the array list[first..last]
int partition (int list[U, int first, int last)
24
25
26
{
int pivot = list[first]; // Choose the first element as the pivot
int low = first + 1; // Index for forward search
27
28
29
int high - last; // Index for backward search
30
31
while (high > low)
32
{
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
33
34
35
36
// Search backward from right
while (low <- high && list[high] > pivot)
37
38
39
high--;
40
// Swap two elements in the list
if (high > low)
41
42
43
{
44
int temp = list[high];
list(high] = list[low];
list(low] - temp;
45
46
47
48
}
49
while (high > first && list[high] >= pivot)
high--;
50
51
52
// Swap pivot with list[high]
if (pivot > list[high])
53
54
55
{
list[first] - list[high];
list[high] = pivot;
return high;
56
57
58
59
60
else
61
{
62
return first;
63
}
64
65
int main()
67
66
const int SIZE = 9;
int list[] = {1, 7, 3, 4, 9, 3, 3, 1, 2};
quickSort (list, SIZE);
for (int i - 0; i < SIZE; i++)
cout <« list[i] <<
68
69
70
71
72
%3D
";
73
74
return 0;
75](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F04d72593-097f-4b69-938e-8eb3398dcc08%2Fce6fdb8f-c51a-46cf-9645-62daf2f3377b%2Foylfoi_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

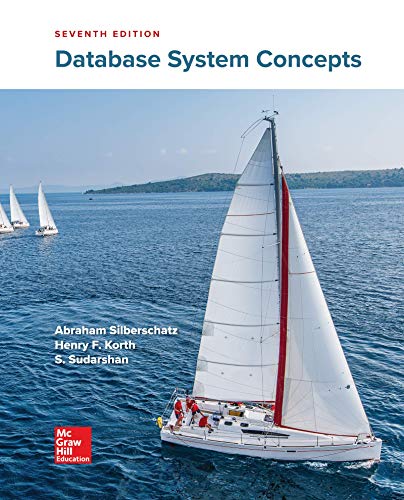
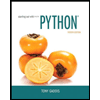
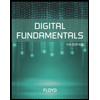
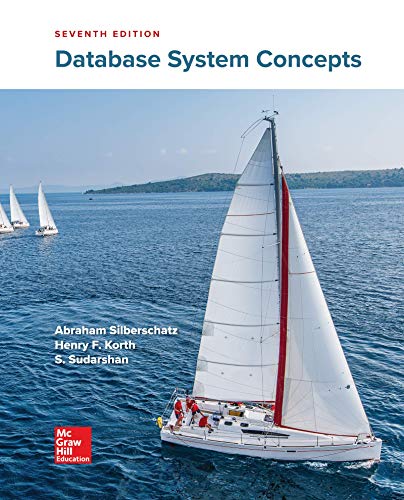
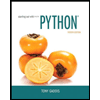
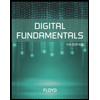
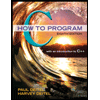
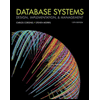
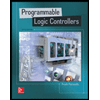