Write a program with a loop that lets the user enter a series of positive integers. The user should enter -99 to signal the end of the series. Any negative number other than -99 must be rejected with a message “ERROR: no negative values other than -99 accepted!”. After all the numbers have been entered, the program should display the largest and the smallest numbers entered. If no numbers were entered (user entered -99 right away) a message “No numbers were entered” must appear. Test cases User input (1 number): -99 Produces output “No numbers were entered” Input 2 numbers: 1 , -99 Output: the largest number: 1 ; the smallest number: 1 Input 5 numbers :1 , 2 ,55, 0, -99 Output: the largest number: 55 ; the smallest number: 0
Write a
Test cases
- User input (1 number): -99
- Produces output “No numbers were entered”
- Input 2 numbers: 1 , -99
- Output: the largest number: 1 ; the smallest number: 1
- Input 5 numbers :1 , 2 ,55, 0, -99
- Output: the largest number: 55 ; the smallest number: 0
Write a program that generates 100 random integers in a given range and stores the integers in a file with a given name. When numbers are written into the file each integer is placed on a separate line.
Requirements
The program must:
- Ask user to provide a range of random integers to be generated.
- Use exceptions
mechanism to validate user input to be of correct type. If the user enters anything other than integer, the program must not crash but instead output an error message containing word “ERROR”, and ask user for input again.- See InputValidation.java to get example of how to do that.
- Use two validation loops to make the user provide two integers.
- Both numbers of the range provided by the user must be positive integers. If the user provides negative value, a message containing word “ERROR” must be displayed, and the user must be asked to provide an integer again.
- Continue asking user for input until the numbers fit both requirements.
- See sample interaction with a user below.
- Use exceptions
- Ask user for a file name where the numbers will be written to. If the file does not exist it must be created. If it does exist, the contents of it must be replaced with the new set of random numbers.
- The user may give the numbers of the range in incorrect order. Your program must not break when that happens. Make sure that lower limit is smaller than upper limit. If it is not the case – swap the numbers and continue with random number generation.
- Open the file with the given name, generate random numbers and write them in the file, placing each new number on a new line.
- Use try/catch block to handle possible IOExceptions.
- IMPORTANT: Your main() must not throw any exceptions.
Sample user interaction - all input is correct
Enter the minimum value as a positive integer: 1
Enter the maximum value as a positive integer: 5
Enter the filename to write into: abc.txt
Data written to the file.
Sample user interaction - incorrect input handled
Enter the minimum value as a positive integer: gdfgfd
Input ERROR. Number entered was not an integer.
Enter the minimum value as a positive integer: -5
Input ERROR. Number entered was not positive.
Enter the minimum value as a positive integer: 8
Enter the maximum value as a positive integer: ewfw
Input ERROR. Number entered was not an integer.
Enter the maximum value as a positive integer: 9.7
Input ERROR. Number entered was not an integer.
Enter the maximum value as a positive integer: 3
Enter the filename to write into: someFile.txt
Data written to the file.

There are two programs and they are not interlinked. So as per our guidelines we only answer the first one.
- The program will take positive integer from user.
- First program will ask the user to input number of element they want in an array.
- Then system will ask input element for the array.
- If the user input value -99 then the system will popup message saying “No input number”.
- The system will find the minimum and maximum number from the array.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

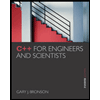
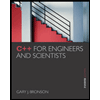