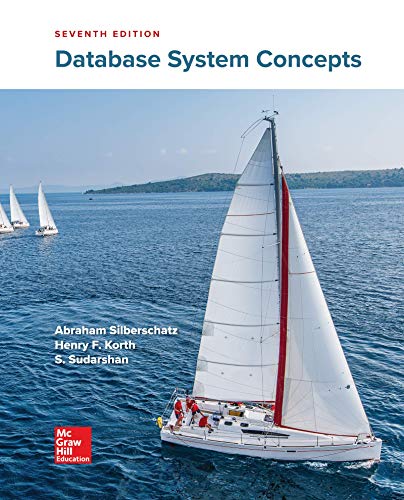
Write a program to solve the H problem for general values of n > 0 and k ≥ 0. Your program must read the values of n and k from cin and write the winners for to cout. Each problem will be on its own input line containing values for n and k (in that order). For each input problem, your program should output a single line containing only the number of the survivor (so you’ll know in which position to stand). Your program may assume that both n and k can be held by int variables
OUTPUT (Note I have numbered my data from 0 to n-1, but final answer is adjusted by 1 for actual position)
Please enter number of people and step :5 2
finding live 0
incrementing to 1
killed 1
finding live 2
incrementing to 3
killed 3
finding live 4
incrementing to 0
killed 0
finding live 2
incrementing to 4
killed 4
survivor is #3
You may write your code in C++, Java or Python an submit either a replit link or a zipped file with documented code AND submit a word doc with a screenshot of your ouput. Failure to do this will result in loss of credit

Step by stepSolved in 4 steps with 1 images

- Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The function integer_to_reverse_binary() should return a string of 1's and 0's representing the integer in binary (in reverse). The function reverse_string() should return a string representing the input string in reverse.def integer_to_reverse_binary(integer_value)def reverse_string(input_string) Note: This is a lab from a previous chapter that now requires the use of a function. def integer_to_reverse_binary(integer_value): if number > 0: number % 2 number = number // 2 return number def…arrow_forwardUse the cubicSpline module to write programs that interpolate between a given data point with a cubic spline. The program must be able to evaluate the interpolant for more than one x value. As a test, use the data points specified in the previous Example and calculate the interpolants at x = 1.5 and x = 4.5 (due to symmetry, these values must be the same) import numpy as np from cubicSpline import * xData = np.array([1,2,3,4,5], float) yData = np.array([0,1,0,1,0], float) k = curvatures (xData, yData) while True: try: x = eval(input("\nx ==> ")) except SyntaxError: break print("y=", evalSpline (xData, yData, k, x)) input ("Done. Press return to exit") Exercise Fix the Cubic Spline Program There is an error in the Cubic Spline program, fix it and evaluate the values of x 1/2 = 1.5 and x = 4.5, where the results of both values must be the same.arrow_forwardWrite a program that will find the longest common substring from a series of two to many words given to you by the user. For example, your program will ask the user how many words he/she wants to compare, with two being the minimum input. The longest common substring of the strings “QWERTY”, “TEWERU”, “YWERQT” is WER. Your algorithm should work for any number of inputs (words), if there is no match of common substrings, indicate to the user with a message. A common algorithm to follow when making this program is first to prompt the user for how many words he/she wants to compare (check for valid input of a number 2 to ‘n’) and then prompt the user for the strings (words) and following this return the common substring if one is present.arrow_forward
- Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The IntegerToReverseBinary() function should return a string of 1's and 0's representing the integer in binary (in reverse). The ReverseString() function should return a string representing the input string in reverse. string IntegerToReverseBinary(int integerValue)string ReverseString(string userString) #include <iostream>using namespace std; /* Define your functions here */ int main() {/* Type your code here. Your code must call the functions. */ return 0;} Please help me with this problem using…arrow_forwardI need to write a program for a Lion Hunt. The program should calculate the number of hours it will take a group of hunters to find the lion in the worst case scenario. We asumme that The lion has b b Hiding spots where 2 ≤ b ≤ 10 , 000 2 ≤ b ≤ 10 , 000 . We assume that there are s s Hunters where 1 ≤ s ≤ 1 , 000 1 ≤ s ≤ 1 , 000 . We assume that there are g g number of hunters, who need to be in a group to travel safely where 1 ≤ g ≤ s 1 ≤ g ≤ s . We assume that it takes a group 1 hour to search any of The lions hiding spots The input consists of a single line containing three integers ?, ? and ?, where 2≤?≤1000 is the number of hiding spots in the jungle. 1≤ e. Sample input/output is shown in attached photoarrow_forwardYour team was asked to program a self-driving car that reaches its destination with minimum travel time. Write an algorithm for this car to choose from two possible road trips. You will calculate the travel time of each trip based on the car current speed and the distance to the target destination. Assume that both distances and car speed are givenarrow_forward
- Coding in Pythonarrow_forwardWrite a program that takes an integer N and uses the function “np.random.randint(low = 0, high = N)” to generate a random sequence of integers between 0 and N-1. Run experiments to validate the hypothesis that the number of integers generated before the first repeated value is found is “~sqrt(pi * N / 2)”. please help with last part Show that as n increases (e.g. with a doubling experiment), from n = 2 to n = 1,000, the value of “day_sim(n)” approaches “sqrt(pi * n / 2)”.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
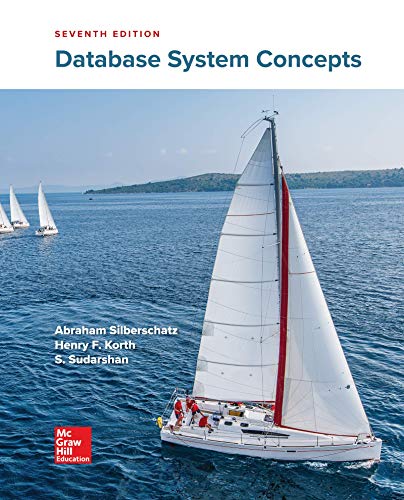
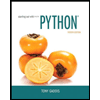
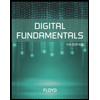
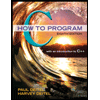
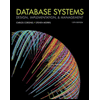
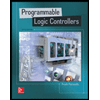