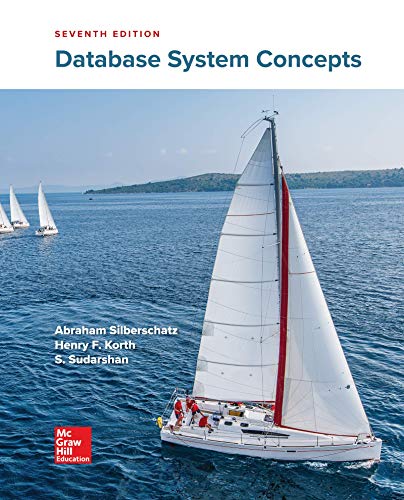
Write a
Sample Output

Note: Since no programming language is mentioned. I am attempting this in python. if you need it in any other language please repost the question and mention the language.
Required:
Write a program that uses a while loop to store goals and assists for Lightning players in a text file. The program should prompt the user for player names, goals, and assists as shown below. Enter data for at least five players. You can make up the names and data. Each datum should be stored on its own line in the file.
Step by stepSolved in 2 steps with 3 images

- How to fix my error? assignment question This program will have three functions, the main() create() and retrieve(). main() will call create() and then call retrieve() create() will prompt the user to enter any number of course name / grade pairs and write them to a file named grades.txt. Pressing Enter with no input value on the course name will exit the loop, close the file, print "File was created and closed" and return the bool value True to the main() retrieve() will open and read grades.txt. The function then will print the course names and scores as shown below. The average score should be calculated as well as a simple GPA and displayed to two decimal places and then return variable used for the GPA to the main() . See sample run below in which 4 course names were entered, but note that more or fewer courses could have been entered. Tip: Simple GPA, In its easiest form, an A=4, B=3, C=2, D=1, F=0. For each class you have, you assign the correct number to the letter grade,…arrow_forwardPYTHON without def function Problem Statement Suppose an input file (called "paragraph.txt") contains a paragraph of text. Read in the file and then count/print the number of times the word "book" is in the file. Hint: Punctuation should be ignored, i.e., "book?" and "book!" should increase the count. The program should also be case-insensitive. Sample Input (File paragraph.txt) I have a book. It's called Harry Potter. It's a pretty good book! Do you want to buy my BOOK? Sample Output The word 'book' appears 3 time(s).arrow_forwardSummary In this lab, you open a file and read input from that file in a prewritten Python program. The program should read and print the names of flowers and whether they are grown in shade or sun. The data is stored in the input file named flowers.dat. Instructions Open the source code file named Flowers.py Write a while loop to read the input until EOF is reached. In the body of the loop, print the name of each flower and where it can be grown (sun or shade). Execute the program. ----------------------------------------------------- flowers.dat (file information, lists flower and temp it needs to grow) AstilbeShadeMarigoldSunBegoniaSunPrimroseShadeCosmosSunDahliaSunGeraniumSunFoxgloveShadeTrilliumShadePansySunPetuniaSunDaisySunAsterSun -------------------------------------------- GIVEN CODE: # Flowers.py - This program reads names of flowers and whether they are grown in shade or sun from an input # file and prints the information to the user's screen. # Input: flowers.dat. #…arrow_forward
- Range for loop should be used even the block code needs to access index. Group of answer choices True Falsearrow_forwardim usually using python Write a program named filemaker.py that will be used to store the first name and age of some friends in a text file named friends.txt. The program must use a while loop that prompts the user to enter the first name and age of each friend. Each of these entries should be written to its own line in the text file (2 lines of data per friend). The while loop should repeat until the user presses Enter (Return on a Mac) for the name. Then, the file should be closed and a message should be displayed. See Sample Output.SAMPLE OUTPUT Enter first name of friend or Enter to quit DennyEnter age (integer) of this friend 24Enter first name of friend or Enter to quit PennyEnter age (integer) of this friend 28Enter first name of friend or Enter to quit LennyEnter age (integer) of this friend 20Enter first name of friend or Enter to quit JennyEnter age (integer) of this friend 24Enter first name of friend or Enter to quit File was createdarrow_forwardThis is a C++ program, to be done in a .cpp file Problem Statement: Write rules for the user on how to play the game in a text file. Then, create a program that will read in the text file and display it out to the user. LCR Game Rules: The Dice● There are three dice rolled each turn. Each die has the letters L, C, and R on it along with dots on the remaining three sides. These dice determine where the player’s chips will go.○ For each L, the player must pass one chip to the player sitting to the left.○ For each R, the player must pass one chip to the player sitting to the right.○ For each C, the player must place one chip into the center pot and those chips are now out of play.○ Dots are neutral and require no action to be taken for that die.The Chips● Each player will start with three chips.● If a player only has one chip, he/she rolls only one die. If a player has two chips left, he/she rolls two dice. Once a player is out of chips, he/she is still in the game (as he/she may get…arrow_forward
- PYTHON: I need to get the avg statement out of the loop so that the output prints correctly. Any suggestions. Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: Read the file name of the tsv file from the user. Open the tsv file and read the student information. Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: A: 90 =< x B: 80 =< x < 90 C: 70 =< x < 80 D: 60 =< x < 70 F: x < 60 Compute the average of each exam. Output the last names, first names, exam…arrow_forwardplease save number random and file and when print on monitor read from file and every num in single linearrow_forwardprogram6_1.pyBetterBuy is having a sale. Write a program that uses a while loop to store to a file the names, regular prices, and price reductions (as percents) for selected items in the promotion. The program should enable the user to enter this data from the keyboard as shown below . Each datum should be stored on its own line in the file. i already finished this program and came up with this code: f = open('data.txt', 'w+')while(True): name = input("Enter item name or Enter to quit ") if name == "" : break cost = input("Enter item's regular price ") reduction = input("Enter reduction percent for sale ") f.write(name + ' ' + cost + ' ' + reduction + ' \n')f.close()print("File was created") i need program6_2.pyarrow_forward
- C programarrow_forwardPython programming help I need help of question 1 to read a file from persons.txt Write a program that takes a person’s details (name, age and a city), and writes to a file (persons.txt) using a loop repeatedly. You should write three person’s to the file. The program terminates on entering any key by the user, except on (Y or y) key. Sample output of the first run of the program is shown below: Enter name: JohnEnter age: 20Enter city: SydneyData saved: John 20 SydneyPress (Y or y) to add another person, or any key to exit Question 1) Write a program that read a file (persons.txt) from previous question given on the top of this question, and shows all the records/lines from the file as a nicely formatted report with a header as shown: Name Age City +---------------------+--------------------+--------------arrow_forwardSummary In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. Instructions Make sure the file MovieGuide.py is selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 must be written with this code: """ MovieGuide.py This program allows each theater patron to enter a value from 0 to 4 indicating the number of stars that the patron awards to the Guide's featured movie of the week. The program executes…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
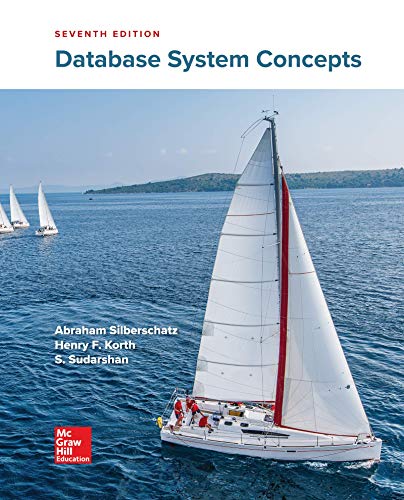
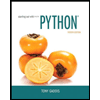
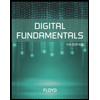
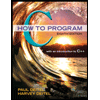
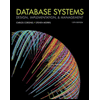
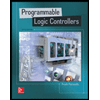