Write a program that takes in three integers as inputs and outputs the largest value. Use a try block to perform all the statements. Use a catch block to catch any NoSuchElementException caused by missing inputs. Then output the number of inputs read and the largest value, or output "No max" if no inputs are read. Note: Because inputs are pre-entered when running a program in the zyLabs environment, the system throws the NoSuch ElementException when inputs are missing. Test the program by running the program in the Develop mode. Hint: Use a counter to keep track of the number of inputs read and compare the inputs accordingly in the catch block when exception is caught. Ex: If the input is: 375 the output is: 7 Ex: If the input is: 3 the system throws the NoSuchElementException and outputs: 1 input (s) read: Max is 3 Ex: If no inputs are entered: the system throws the NoSuchElementException and outputs: 0 input (s) read: No max
Write a program that takes in three integers as inputs and outputs the largest value. Use a try block to perform all the statements. Use a catch block to catch any NoSuchElementException caused by missing inputs. Then output the number of inputs read and the largest value, or output "No max" if no inputs are read. Note: Because inputs are pre-entered when running a program in the zyLabs environment, the system throws the NoSuch ElementException when inputs are missing. Test the program by running the program in the Develop mode. Hint: Use a counter to keep track of the number of inputs read and compare the inputs accordingly in the catch block when exception is caught. Ex: If the input is: 375 the output is: 7 Ex: If the input is: 3 the system throws the NoSuchElementException and outputs: 1 input (s) read: Max is 3 Ex: If no inputs are entered: the system throws the NoSuchElementException and outputs: 0 input (s) read: No max
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter14: Exception Handling
Section: Chapter Questions
Problem 2PE
Related questions
Question
import java.util.Scanner;
import java.util.NoSuchElementException;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int val1;
int val2;
int val3;
int max;
val1 = 0;
val2 = 0;
val3 = 0;
/* Type your code here. */
}
}

Transcribed Image Text:Write a program that takes in three integers as inputs and outputs the largest value. Use a try block to perform all the statements. Use a
catch block to catch any NoSuchElementException caused by missing inputs. Then output the number of inputs read and the largest value,
or output "No max" if no inputs are read.
Note: Because inputs are pre-entered when running a program in the zyLabs environment, the system throws the NoSuch ElementException
when inputs are missing. Test the program by running the program in the Develop mode.
Hint: Use a counter to keep track of the number of inputs read and compare the inputs accordingly in the catch block when an exception is
caught.
Ex: If the input is:
3 7 5
the output is:
7
Ex: If the input is:
3
the system throws the NoSuchElementException and outputs:
1 input (s) read:
Max is 3
Ex: If no inputs are entered:
the system throws the NoSuchElementException and outputs:
0 input (s) read:
No max
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
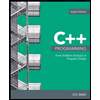
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
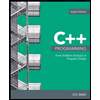
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning