Write a program that reads a list of words. Then, the program outputs those words and their frequencies (case insensitive). Ex: If the input is: hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use lower() to set each word to lowercase before comparing. My code: my_list = input() my_list_lower = my_list.lower() list = my_list_lower.split() for word in list: freq = list.count(word) print(word, freq) the program wants the lower case number count AND for the list of words to remain upper case if they were upper case. how do i make it do that?
Write a program that reads a list of words. Then, the program outputs those words and their frequencies (case insensitive). Ex: If the input is: hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use lower() to set each word to lowercase before comparing. My code: my_list = input() my_list_lower = my_list.lower() list = my_list_lower.split() for word in list: freq = list.count(word) print(word, freq) the program wants the lower case number count AND for the list of words to remain upper case if they were upper case. how do i make it do that?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a program that reads a list of words. Then, the program outputs those words and their frequencies (case insensitive).
Ex: If the input is:
hey Hi Mark hi markthe output is:
hey 1 Hi 2 Mark 2 hi 2 mark 2Hint: Use lower() to set each word to lowercase before comparing.
My code:
my_list = input()
my_list_lower = my_list.lower()
list = my_list_lower.split()
for word in list:
freq = list.count(word)
print(word, freq)
the program wants the lower case number count AND for the list of words to remain upper case if they were upper case. how do i make it do that?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
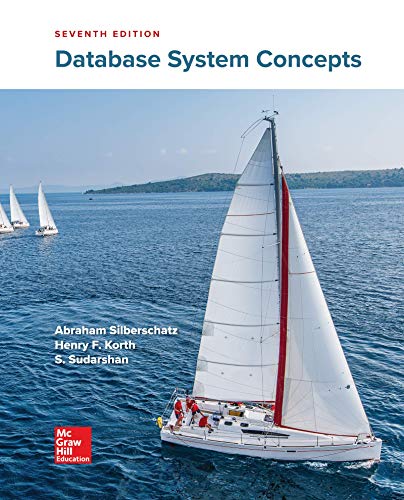
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
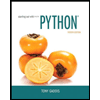
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
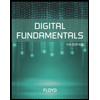
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
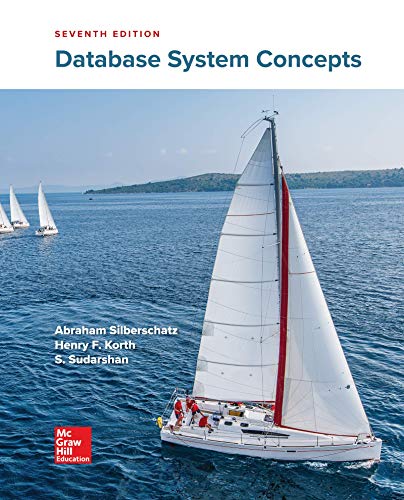
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
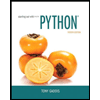
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
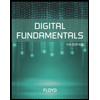
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
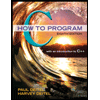
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
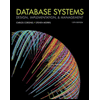
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
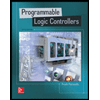
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education