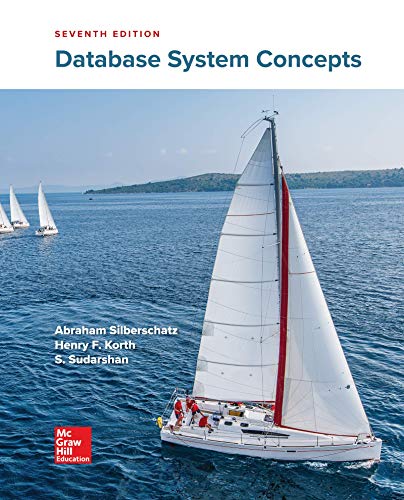
Use C++
Write a program that reads a list of integers, and outputs whether the list contains all even numbers, odd numbers, or neither. The input begins with an integer indicating the number of integers that follow.
Ex: If the input is:
5 2 4 6 8 10
the output is:
all even
Ex: If the input is:
5 1 -3 5 -7 9
the output is:
all odd
Ex: If the input is:
5 1 2 3 4 5
the output is:
not even or odd
Your program must define and call the following two functions. IsVectorEven returns true if all integers in the array are even and false otherwise. IsVectorOdd returns true if all integers in the array are odd and false otherwise.
bool IsVectorEven(
bool IsVectorOdd(vector<int> myVec)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Program31.java Write a program that prompts the user to enter an even multiple of 17 between 200 and 300. Use just ONE boolean expression to test the input and report the input as acceptable, or not. Program32.java Write a program that prompts the user for any day of the week. Report the entered day as either a weekend day, or not. Use a switch and "stack" cases that require the same report as shown on page 103. Your program should handle bad inputs, too. Program33.java Write a program that generates a random Fahrenheit oral temperature between 92 and 108, inclusive. Print the temperature, and then report whether the temperature indicates a fever or not using a conditional operator as on page 105. An oral temperature above 100F is feverish. Program34.java Write a program that prints the ordinal for a random integer from 0 to 100, inclusive. Example ordinals might be 31st, 62nd, 43rd, 55th.arrow_forwardC++arrow_forwardcode must be done in C++ no other language is acceptableWrite a program using nested loops that asks the user to enter a value for the number of rows to display. It should then display that many rows of * and $, with one * in the first row, two in the second row, and so on. However, the last in each row should be aligned (preceded by as many $ as necessary). A sample run would look like this: Enter number of rows: 5 Then the and $ should appear like this: * $ $ $ $ $ $ $ * * * $ $ $ * * *arrow_forward
- using loops in C++ Write a program that will predict the size of a population of organisms. The program should ask the user for the starting number of organisms, their average daily population increase (as a percentage, expressed as a fraction in decimal form: for example 0.052 would mean a 5.2% increase each day), and the number of days they will multiply. A loop should display the size of the population for each day.Prompts, Output Labels and Messages .The three input data should be prompted for with the following prompts: "Enter the starting number of organisms: ", "Enter the average daily population increase (as a percentage): , and "Enter the number of days they will multiply: " respectively. The population sizes displayed should appear on separate lines, each of the form "On day D the population size was P." where D is the day number (starting with 1) and P is the population you calculated for that day.Input Validation.Do not accept a number less than 2 for the starting size of…arrow_forwardQuestio Write a program that receives a series of characters from the user, the program stops if the user enters the same character two consecutive times, the program should display the number of entered characters at the end of the program (the last duplicated character is counted only once): Sample Run: Enter your characters: asbnmkrr we got 7 characters please wirte it in java using loops please answer it in java using netbeans.arrow_forwardWrite a C++ program using loops for a number guessing game. The program generates and stores a random number and prompts a user to guess the number. If user guess is less than the number, display “Sorry, guess is too low”, if user guess is greater than the number, display “Sorry, guess is too high”. If user guesses the right number, display “Congratulations, you have guessed the number”, and number of users tries to guess a number. The program should keep on taking a user input, until user guesses the number. Sample Output 1 (Number 50): Enter a number to guess: 50 “Congratulations, you have guessed the number” in first try. Sample Output 2 (Number 50): Enter a number to guess: 25 “Sorry, guess is too low” Enter a number to guess: 60 “Sorry, guess is too high” Enter a number to guess: 50 “Congratulations, you have guessed the number in 3 tries”, 2.12.0.0arrow_forward
- For C Programming This has to deal with the Fibonacci sequence. Complete a program so that it prints the nth Fibonacci number, where n is an integer value read as input by the program. For example, if the input 4, then the output should be: Fibonacci number 4 is 3. Another example if the input is 7 then the output should be: Fibonacci number 7 is 13. Use a loop for this program.arrow_forwardIn C++arrow_forwardin C programmingarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
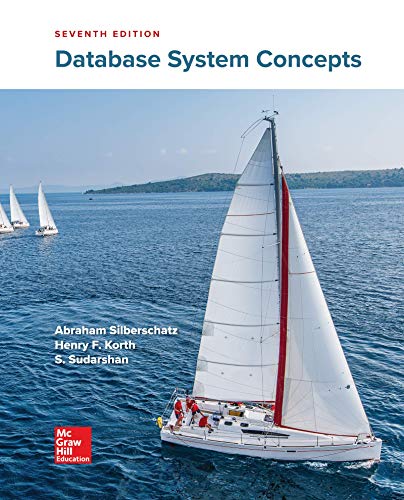
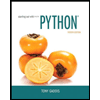
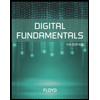
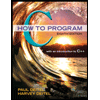
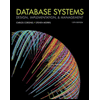
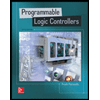