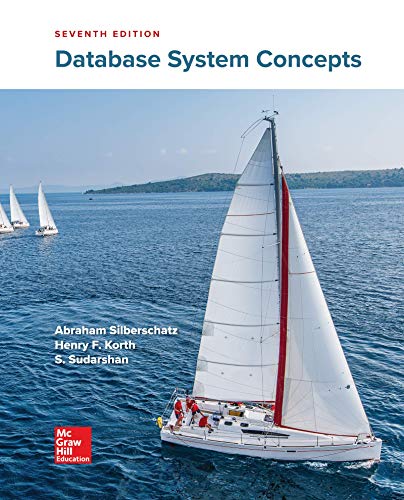
Write a program that reads a line of text, changes each uppercase letter to lowercase, and places each letter both in a queue and onto a stack. The program should then verify whether the line of text is a palindrome (a set of letters or numbers that is the same whether read forward or backward).
Cannot use <queue> Library, have to use queueADT class as shown in the below, "Queue Demo."
Thank you, will up vote.
#include <iostream>
#include <cassert>
using namespace std;
template <class Type>
class queueADT
{
public:
virtual bool isEmptyQueue() const = 0;
virtual bool isFullQueue() const = 0;
virtual void initializeQueue() = 0;
virtual Type front() const = 0; //Function to return the first element of the queue.
virtual Type back() const = 0; //Function to return the last element of the queue.
virtual void addQueue(const Type& queueElement) = 0; //Function to add queueElement to the queue.
virtual void deleteQueue() = 0; //Function to remove the first element of the queue.
};
template <class Type>
class queueType : public queueADT<Type>
{
private:
int maxQueueSize; //variable to store the maximum queue size
int count; //variable to store the number of elements in the queue
int queueFront; //variable to point to the first element of the queue
int queueRear; //variable to point to the last element of the queue
Type *list; //pointer to the array that holds the queue elements
public:
queueType(int queueSize = 100) //Constructor
{
if (queueSize <= 0)
{
cerr << "Size of the array to hold the queue must "
<< "be positive." << endl;
cerr << "Creating an array of size 100." << endl;
maxQueueSize = 100;
}
else
maxQueueSize = queueSize; //set maxQueueSize to queueSize
queueFront = 0; //initialize queueFront
queueRear = maxQueueSize - 1; //initialize queueRear
count = 0;
list = new Type[maxQueueSize]; //create the array to hold the queue elements
}
queueType(const queueType<Type>& otherQueue) //Copy constructor
{
maxQueueSize = otherQueue.maxQueueSize;
queueFront = otherQueue.queueFront;
queueRear = otherQueue.queueRear;
count = otherQueue.count;
list = new Type[maxQueueSize];
//copy other queue in this queue
for (int j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize)
list[j] = otherQueue.list[j];
} //end copy constructor
~queueType() //Destructor
{
delete[] list;
}
const queueType<Type>& operator=(const queueType<Type>& otherQueue) //Overload the assignment operator.
{
int j;
if (this != &otherQueue) //avoid self-copy
{
maxQueueSize = otherQueue.maxQueueSize;
queueFront = otherQueue.queueFront;
queueRear = otherQueue.queueRear;
count = otherQueue.count;
delete[] list;
list = new Type[maxQueueSize];
//copy other queue in this queue
if (count != 0)
for (j = queueFront; j <= queueRear; j = (j + 1) % maxQueueSize)
list[j] = otherQueue.list[j];
} //end if
return *this;
}
bool isEmptyQueue() const //Function to determine whether the queue is empty.
{
return (count == 0);
} //end isEmptyQueue
bool isFullQueue() const //Function to determine whether the queue is full.
{
return (count == maxQueueSize);
} //end isFullQueue
void initializeQueue() //Function to initialize the queue to an empty state.
{
queueFront = 0;
queueRear = maxQueueSize - 1;
count = 0;
}
Type front() const //Function to return the first element of the queue.
{
assert(!isEmptyQueue());
return list[queueFront];
}
Type back() const //Function to return the last element of the queue.
{
assert(!isEmptyQueue());
return list[queueRear];
} //end back
void addQueue(const Type& newElement) //Function to add queueElement to the queue.
{
if (!isFullQueue())
{
queueRear = (queueRear + 1) % maxQueueSize; //use mod
//operator to advance queueRear
//because the array is circular
count++;
list[queueRear] = newElement;
}
else
cerr << "Cannot add to a full queue." << endl;
}
void deleteQueue() //Function to remove the first element of the queue.
{
if (!isEmptyQueue())
{
count--;
Can't share the rest of the demo code because of the character limit.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a method to reverse the content of a stack. Inside the method, you may create exactly one temporary container -- either a stack or a queue. It is alright to create variables of primitive data types (such as, an integer), if required. You must use the following header. public void reverse(Stack s){arrow_forwardJava's LinkedList provides a method listlterator(int index) returning a Listlterator for a list. Declare a variable of LinkedList of integer numbers and write a fragment of Java code to compute and print the sum of the numbers on the list. Do not write code to insert elements to the list and assume that the list already has elements on it. You must only use an iterator obtained from the list (you can not use the get(int index) method) .arrow_forwardWe can declare a new array and copy the items of the old queue to new and by this, it will extend the size of the queue.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
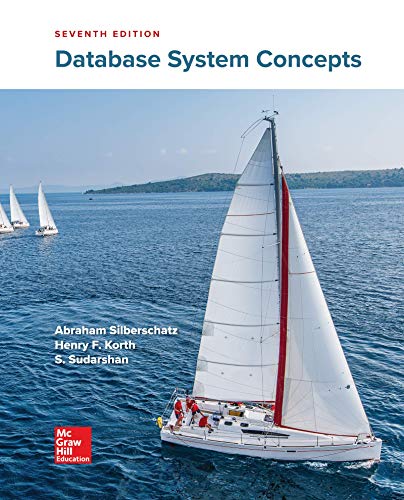
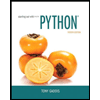
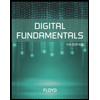
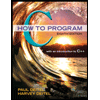
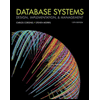
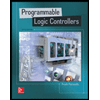