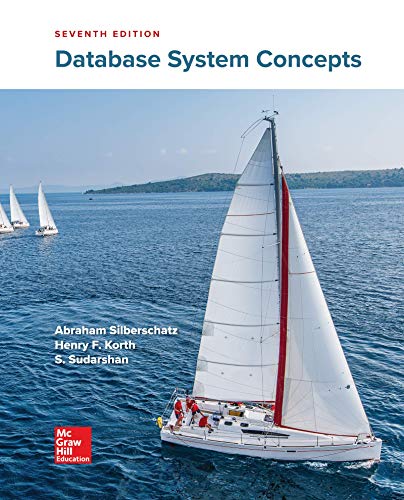
Write a
allocated arrays.
DataFile.txt contains the information of poker cards.
- C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest)
- 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A
- No Joker cards
- Any C cards are lower than any D cards.
DataFile Content(You can write the file specification into your program.):
H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ
,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10
H4, D5, HK, D2
H4, HK, SK
C9,C10
For examples, DJ means J of Diamonds;H7 means 7 of hearts.
Your job
- Create a list by dynamic allocated array and set the size to 20
- Read the first 20 cards in the first line of the file, the put them one by one into the list by implementing and using putItem(). The list must be kept sorted in ascending order. Then print out all the cards in the list in one line separating by commas.
- Then delete the cards indicated in the second line of the file by using deleteItem()
- Then print out all the cards in the list in one line separating by commas.
- Then put the items in the third line in to the list. Must use putItem()
Then print out all the cards in the listin one line separating by commas.
- Search the current list for the elements in the list.
Then output the result as the follows. Yes or No depends on whether the card exists in
the current list. Must implement and use getItem()
C9 NO, C10 YES
- A printAll() function should be defined and called in order to output all the contents in
the list.
- A compareTo() function must be defined and used to compare which card is greater
Use C++ and do not use Standard Library Template. Show detailed comments to help understand please!
Copy following to test with your own .txt file!
H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10
H4, D5, HK, D2
H4, HK, SK
C9,C10

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

I am going over the code and the output seems to be missing steps as it doesn't show in output such as :
- Then delete the cards indicated in the second line of the file by using deleteItem()
- Then print out all the cards in the list in one line separating by commas.
- Then put the items in the third line in to the list. Must use putItem()
It seems to show that you are only reading two lines of the data and not all to fully show the output?
I am going over the code and the output seems to be missing steps as it doesn't show in output such as :
- Then delete the cards indicated in the second line of the file by using deleteItem()
- Then print out all the cards in the list in one line separating by commas.
- Then put the items in the third line in to the list. Must use putItem()
It seems to show that you are only reading two lines of the data and not all to fully show the output?
- Integer m and string my_name are read from input. Then, four strings are read from input and stored in the list names_data. Delete the first element in names_data, and then replace the element at index m with my_name.arrow_forwardQuestion 44 Computer Science A list of elements has a size of 100. Choose the operations where an ArrayList would be faster than a LinkedList. (Select all that apply) Question 5 options: removing from index 99 inserting at index 1 removing from index 4 inserting at index 4 Full explain this question and text typing work onlyarrow_forwardDebug the program debug_me.py. The program should test each of the users in the provided list. · If the list is empty, it should print “There are no users.” · If the user is “Admin,” the program should print “Hello all powerful one.” · Otherwise, for normal users, it should print “You are a normal user.” Test the program with an empty list to confirm correct operation for that case.arrow_forward
- Complete the doctring.def average_daily_temp(high_temps: List[int], low_temps: List[int]) -> List[float]: """high_temps and low_temps are daily high and low temperatures for a series of days. Return a new list of temperatures where each item is the daily average. Precondition: len(high_temps) == len(low_temps) >>> average_daily_temp([26, 27, 27, 28, 27, 26], [20, 20, 20, 20, 21, 21]) [23.0, 23.5, 23.5, 24.0, 24.0, 23.5] """arrow_forwardImplement the following: Given: names = ['ann', 'bill', 'calvin', 'david']ids = [12, 34, 56, 78] 1) Create a dictionary, stuInfo by using the two given lists above.2) Use a loop to print all keys and values in the dictionary stulfno. 3) Print the dictionary, stuInfo. Example Output12 ANN34 BILL 56 CALVIN78 DAVID{'ann': 12, 'bill': 34, 'calvin': 56, 'david': 78} NEED HELP WITH PYTHONarrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- Write a python program that takes two lists, merges thetwo lists, sorts the resulting list, and then finds the median of theelements in the two lists. You cannot use python build-in sort() function #todo#You can use math functions like math.floor to help the calculationimport math #todo#You can use math functions like math.floor to help the calculation import math def task7(list_in_1, list_in_2): # YOUR CODE HERE return median please use these variables no print function pleasearrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardPOEM_LINE = str POEM = List[POEM_LINE] PHONEMES = Tuple[str] PRONUNCIATION_DICT = Dict[str, PHONEMES] def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int], word_to_phonemes: PRONUNCIATION_DICT) -> bool: """Return True if and only if the lines from poem_lines with index in lines_to_check all rhyme, according to word_to_phonemes. Precondition: lines_to_check != [] >>> poem_lines = ['The mouse', 'in my house', 'electric.'] >>> lines_to_check = [0, 1] >>> word_to_phonemes = {'THE': ('DH', 'AH0'), ... 'MOUSE': ('M', 'AW1', 'S'), ... 'IN': ('IH0', 'N'), ... 'MY': ('M', 'AY1'), ... 'HOUSE': ('HH', 'AW1', 'S'), ... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K', ... 'T', 'R', 'IH0', 'K')} >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True…arrow_forward
- Having trouble with creating the InsertAtEnd function in the ItemNode.h file below. " // TODO: Define InsertAtEnd() function that inserts a node // to the end of the linked list" Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts ------------------------------------------------------- main.cpp -------------------------------------------------------- #include "ItemNode.h" int main() { ItemNode *headNode; // Create intNode objects ItemNode *currNode; ItemNode *lastNode; string item; int i; int input; // Front of nodes list headNode = new ItemNode(); lastNode = headNode; cin >> input; for (i = 0; i < input; i++) { cin >> item;…arrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forwardIn Python Write code that creates a LIST (data type) of only the first names of the students who are juniors. call it juniorRoster= Given the folowing data set: studentDataScores =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
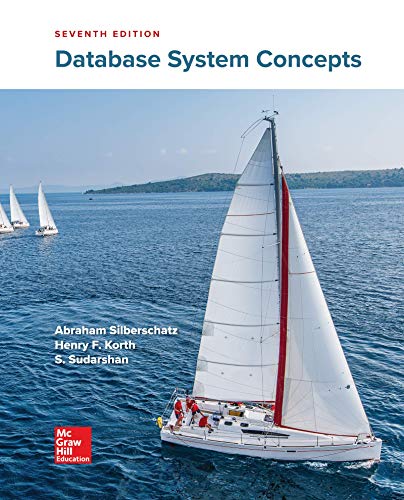
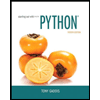
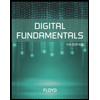
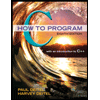
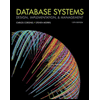
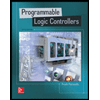