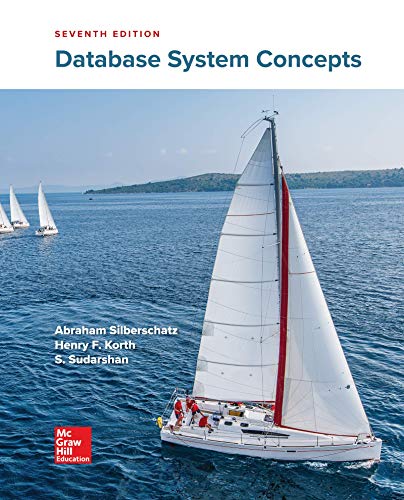
Write a program that determines an integer value the user had in mind. Your program first asks the user to enter the lower and upper bounds of the range that the integer belongs to, and then presents a series of questions “Is the number greater than x?” with yes/no answers to the user in order to determine the integer value that the user had in mind.
For instance, if the integer in mind is 5 and the interaction between the user and your program is:
Please enter the lower bound: -10
Please enter the upper bound: 50
Is the number greater than 20 (Y/N)? n
Is the number greater than 4 (Y/N)? y
Is the number greater than 12 (Y/N)? n
Is the number greater than 8 (Y/N)? n
Is the number greater than 6 (Y/N)? n
Is the number greater than 5 (Y/N)? n
Your program should then print:
The number you had in mind is 5
Your program should ask roughly log2(upper_bound − lower_bound) questions. Your program A3_1.py should include a main() function and may include other functions that you prefer. The execution section of your program should only include one instruction that calls main().

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Write the pseudocode for Aunt Joan’s egg ranch problem. Aunt Joan has a large number of chicken coops. Each day she gathers eggs from a different number of chicken coops and wants to track how many dozens and excess eggs she gathers from each individual coop. She will enter a series of numbers representing the number of eggs gathered from each chicken coop. The program will calculate and output the number of dozens as well as the number of excess eggs for that coop before entering the input for the next coop. The program will continue this process until a negative number is entered.arrow_forwardWrite a program that asks the user to enter a number of seconds and works as follows: There are 60 seconds in a minute. If the number of seconds entered by the user is greater than or equal to 60, the program should convert the number of seconds to minutes and seconds. There are 3,600 seconds in an hour. If the number of seconds entered by the user is greater than or equal to 3,600, the program should convert the number of seconds to hours, minutes, and seconds. There are 86,400 seconds in a day. If the number of seconds entered by the user is greater than or equal to 86,400, the program should convert the number of seconds to days, hours, minutes, and seconds.arrow_forwardTransient PopulationPopulations are affected by the birth and death rate, as well as the number of people who move in and out each year. The birth rate is the percentage increase of the population due to births and the death rate is the percentage decrease of the population due to deaths. Write a program that displays the size of a population for any number of years. The program should ask for the following data: The starting size of a population P The annual birth rate (as a percentage of the population expressed as a fraction in decimal form)B The annual death rate (as a percentage of the population expressed as a fraction in decimal form)D The average annual number of people who have arrived A The average annual number of people who have moved away M The number of years to display nYears Write a function that calculates the size of the population after a year. To calculate the new population after one year, this function should use the formulaN = P + BP - DP + A - Mwhere N is the…arrow_forward
- In various applications, you are often asked to compute the mean and standard deviation of data. The mean is simply the average of the numbers. The standard deviation is a statistic that tells you how tightly all the various data are clustered around the mean in a set of data. For example, what is the average age of the students in a class? How close are the ages? If all the students are the same age, the deviation is 0. Write a program that prompts the user to enter any number of values into a double array, and then calculates and displays the mean and standard deviations of these numbers using the following formulas: (z - mean) z+z3+ *** +zn deviation = mean = n-1 Required Methods You must write your program so that the following methods are defined/implemented and used (called): • /* Compute the deviation of double values */ public static double deviation (double[] x) • /* Compute the mean of an array of double values */ public static double mean (double[] x) Sample Run (user input…arrow_forwardWrite a console program, that will calculate the pocket money saved over a period, with one cent at the start of account setup, will double every day. For example, if the opening balance is one cent the first day, it will be two cents the second day, and continues to double each day. The console program you will write, should ask the user for the number of days, and display a table showing what the saving was for each day, and then show the total savings at the end of the period. The output should be displayed in a dollar amount, not the number of cents.arrow_forwardFor this question, you will write a program that prompts the user to enter an integer value between 1 and 1000 (exclusive). Your program should then print out a sequence of numbers between the given value and 1 (inclusive) following the pattern below:For a given number ai, If ai is even, then ai+1 = floor(ai / 2) If ai is odd, then ai+1 = 3ai + 1 Your program should stop printing numbers once the sequence reaches the value of 1. If the user enters a number that is not between 1 and 1000 (exclusive), print "Invalid input." and prompt the user for a number again. This sequence is referred to as the Collatz sequence. The Collatz Conjecture states that this sequence will eventually reach the value of 1 for any starting number, a fact that has not yet been proven by modern mathematics! Note: floor(x) means that x should be rounded down to the nearest integer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
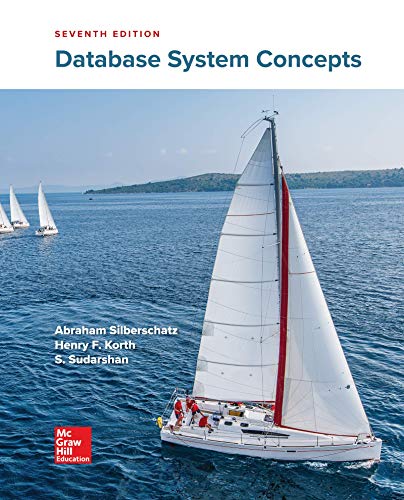
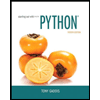
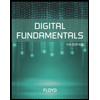
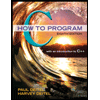
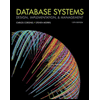
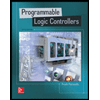