write a program named MarshallsRevenue that prompts a user for the number of interior and exterior murals scheduled to be painted during the next month. Compute the expected revenue for each type of mural. Interior murals cost $500 each, and exterior murals cost $750 each. Also, display the total expected revenue and a statement that indicates whether more interior murals are scheduled than exterior ones.
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
In Chapter 1, you created two programs to display the motto for Marshall’s Murals. Now write a



Trending now
This is a popular solution!
Step by step
Solved in 4 steps

this is error message I get
![## Educational Webpage: Understanding a Simple C# Program for Calculating Mural Revenues
### Overview
This C# program calculates expected revenues from interior and exterior murals. It also compares the revenues to determine which type yields more financial return.
### Code Explanation
#### Libraries and Namespace
```csharp
using System;
using static System.Console;
using System.Globalization;
```
These lines import essential libraries and namespaces for input, output, and globalization features.
#### Define Class and Main Method
```csharp
class MarshallsRevenue
{
static void Main(string[] args)
{
```
The class `MarshallsRevenue` contains the `Main` method where program execution begins.
#### Variables Declaration
```csharp
int int_num, ext_num, r;
double int_rev, ext_rev, tot_rev = 0;
```
This declares integer variables for the number of interior and exterior murals and double variables for their respective revenues and total revenue.
#### Input Handling
```csharp
Console.WriteLine("Enter a Number of interior murals : ");
int_num = int.Parse(Console.ReadLine());
Console.WriteLine("Enter a Number of exterior murals : ");
ext_num = int.Parse(Console.ReadLine());
```
This section prompts the user to input the number of interior and exterior murals and parses the input into integers.
#### Revenue Calculation
```csharp
int_rev = int_num * 500;
ext_rev = ext_num * 750;
tot_rev = int_rev + ext_rev;
```
Here, the program calculates revenues: $500 per interior mural and $750 per exterior mural. It then calculates the total expected revenue.
#### Output Generated Revenue
```csharp
Console.WriteLine(int_num + " interior murals are scheduled for a total of " + int_rev);
Console.WriteLine(ext_num + " exterior murals are scheduled for a total of " + ext_rev);
Console.WriteLine("Total revenue expected: " + tot_rev);
```
This outputs the calculated revenues for each type of mural and the total revenue.
#### Revenue Comparison
```csharp
if (int_rev > ext_rev)
{
Console.WriteLine("The cost of interior murals is more than exterior murals");
}
else if (ext_rev > int_rev)
{
Console.WriteLine("The cost of exterior murals is more than interior murals");
}
```
This logic compares interior and exterior mural revenues and outputs which type is more lucrative.
#### Code Details
- **Warning**: There](https://content.bartleby.com/qna-images/question/5ac771d0-d256-4ee4-b7ac-1580c40cec9b/a251368c-a358-4e9b-8adf-b574514bb10c/avzcxam_thumbnail.png)
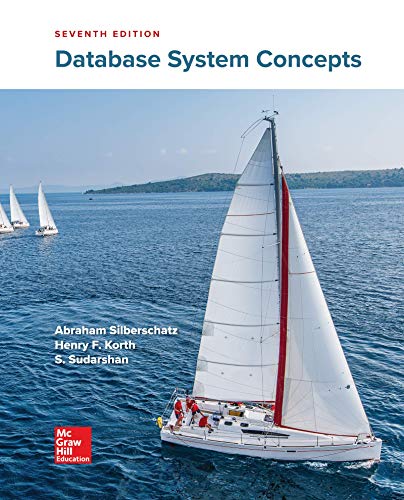
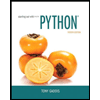
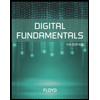
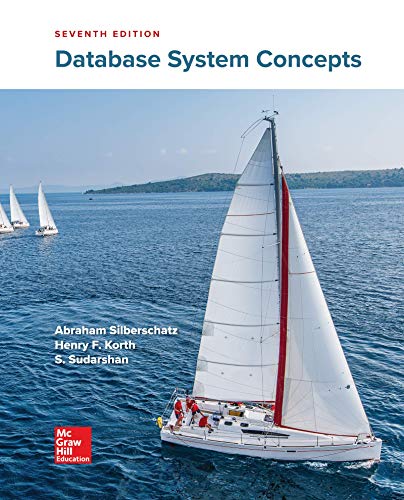
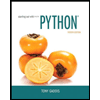
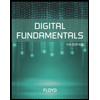
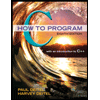
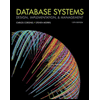
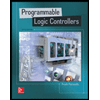