write a program (its name should be StudentPoll.java) that first reads an integer, N, for the number of responses from students and then creates an array of N elements of type int to store the responses. Use SecureRandom to generate N random integers of values between 3 and 10 inclusive as the response values (i.e., the survey values) for the array. The program should print out the distribution of the survey like the following outputs. public class StudentPoll { public static void main(String[] args) { // student response array (more typically, input at runtime) int[] responses = {1, 2, 5, 4, 3, 5, 2, 1, 3, 3, 1, 4, 3, 3, 3, 2, 3, 3, 2, 14}; int[] frequency = new int[6]; // array of frequency counters // for each answer, select responses element and use that value // as frequency index to determine element to increment for (int answer = 0; answer < responses.length; answer++) { try { ++frequency[responses[answer]]; } catch (ArrayIndexOutOfBoundsException e) { System.out.println(e); // invokes toString method System.out.printf(" responses[%d] = %d%n%n", answer, responses[answer]); } } System.out.printf("%s%10s%n", "Rating", "Frequency"); // output each array element's value for (int rating = 1; rating < frequency.length; rating++) { System.out.printf("%6d%10d%n", rating, frequency[rating]); } } }
write a
public class StudentPoll {
public static void main(String[] args) {
// student response array (more typically, input at runtime)
int[] responses =
{1, 2, 5, 4, 3, 5, 2, 1, 3, 3, 1, 4, 3, 3, 3, 2, 3, 3, 2, 14};
int[] frequency = new int[6]; // array of frequency counters
// for each answer, select responses element and use that value
// as frequency index to determine element to increment
for (int answer = 0; answer < responses.length; answer++) {
try {
++frequency[responses[answer]];
}
catch (ArrayIndexOutOfBoundsException e) {
System.out.println(e); // invokes toString method
System.out.printf(" responses[%d] = %d%n%n",
answer, responses[answer]);
}
}
System.out.printf("%s%10s%n", "Rating", "Frequency");
// output each array element's value
for (int rating = 1; rating < frequency.length; rating++) {
System.out.printf("%6d%10d%n", rating, frequency[rating]);
}
}
}

![public class BarChart {
public static void main(String[] args) {
int[] array = {0, 0, 0, 0, 0, 0, 1, 2, 4, 2, 1};
System.out.println("Grade distribution:");
// for each array element, output a bar of the chart
for (int counter = 0; counter < array.length; counter++)
// output bar label (" ")
if (counter
10) {
==
System.out.printf("%5d: ", 10);
}
else {
System.out.printf("%02d:
counter * 1);
}
// print bar of asterisks
for (int stars
0; stars < array[counter]; stars++)
System.out.print("*");
}
System.out.println();
}
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F36b85914-2744-42f7-b8bd-af21a659e2ca%2Fbf1b37f7-f281-40dd-b928-679374abbbbc%2Fupzk54n_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

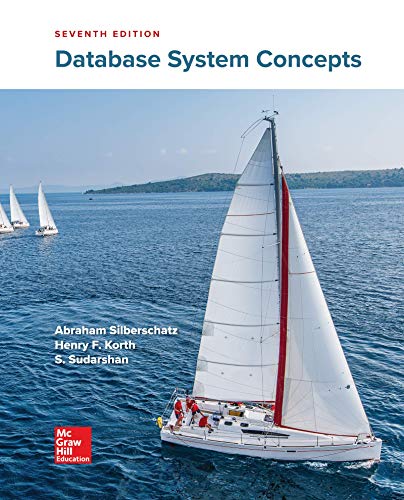
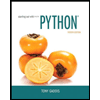
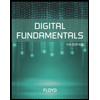
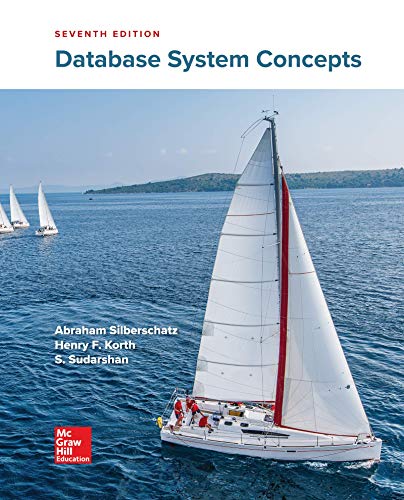
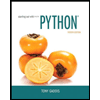
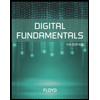
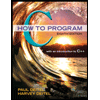
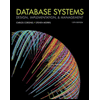
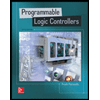