Write a program in C/C++ to that receives N number of integer values from a user, stores the inputted values in a binary search tree, and performs some basic operations on the tree. The program displays the elements of the binary search tree in in-order traversal. The program then prompts the user to provide an integer number (target value) to search in the tree. If the target value does not appear in the tree, the program inserts the value in an appropriate place according to the property of node insertion of binary search tree. If the target value appears in the tree, the program deletes the target value from the tree and preserves the property of binary search tree. In both cases, the program displays the modified binary search tree in in- order traversal. Your output format should be as shown in the test runs. Your program must contain the following user-defined functions: - insertNode – this function inserts the user’s input and creates a binary search tree. - inOrder – this function displays the entire tree in in-order traversal. - searchElement – this function receives the target value to search in the tree. - deleteNode – this function removes the target element from the tree. This function will only be executed if the target element appears in the tree. Example test runs Test Run 1: Enter the number of elements: 5 Enter 5 numbers: 30 50 40 10 20 Tree elements: 10 20 30 40 50 Enter the target number to search: 100 The target element 100 is not in the tree Inserting 100 in the tree Updated tree: 10 20 30 40 50 100 Test Run 2: Enter the number of elements: 7 Enter 7 numbers: 89 45 -1 40 8 7 50 Tree elements: -1 7 8 40 45 50 89 Enter the target number to search: -1 The target element -1 is in the tree. Deleting -1 from the tree. Updated tree: 7 8 40 45 50 89
Write a program in C/C++ to that receives N number of integer values from a user, stores the inputted values in a binary search tree, and performs some basic operations on the tree. The program displays the elements of the binary search tree in in-order traversal. The program then prompts the user to provide an integer number (target value) to search in the tree. If the target value does not appear in the tree, the program inserts the value in an appropriate place according to the property of node insertion of binary search tree. If the target value appears in the tree, the program deletes the target value from the tree and preserves the property of binary search tree. In both cases, the program displays the modified binary search tree in in- order traversal.
Your output format should be as shown in the test runs.
Your program must contain the following user-defined functions:
- insertNode – this function inserts the user’s input and creates a binary search tree.
- inOrder – this function displays the entire tree in in-order traversal.
- searchElement – this function receives the target value to search in the tree.
- deleteNode – this function removes the target element from the tree. This function will only be executed if the target element appears in the tree.
Example test runs
Test Run 1:
Enter the number of elements: 5
Enter 5 numbers: 30 50 40 10 20
Tree elements: 10 20 30 40 50
Enter the target number to search: 100 The target element 100 is not in the tree Inserting 100 in the tree
Updated tree: 10 20 30 40 50 100
Test Run 2:
Enter the number of elements: 7
Enter 7 numbers: 89 45 -1 40 8 7 50
Tree elements: -1 7 8 40 45 50 89
Enter the target number to search: -1
The target element -1 is in the tree.
Deleting -1 from the tree.
Updated tree: 7 8 40 45 50 89

Step by step
Solved in 2 steps with 2 images

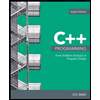
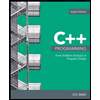