Write a program in C++ that creates a file and writes data into that file column wise. The problem is that there is some data that I want to keep there permantely and I do not want to replace it every time. For example this format: TIME DAY MONTH YEAR 01:25 07 JUNE 2008 14:00 30 MARCH 2019 I have (TIME/ DAY/ MOTH/ YEAR) and I want to write that first and that any data that I add later is written under that and so on. If I want to add the row (05:05/ 10/ July/ 2000) following the pattern. Write code that checks if the file has data and write that data under the lines you already have.
Write a program in C++ that creates a file and writes data into that file column wise. The problem is that there is some data that I want to keep there permantely and I do not want to replace it every time. For example this format:
TIME DAY MONTH YEAR
01:25 07 JUNE 2008
14:00 30 MARCH 2019
I have (TIME/ DAY/ MOTH/ YEAR) and I want to write that first and that any data that I add later is written under that and so on.
If I want to add the row (05:05/ 10/ July/ 2000) following the pattern. Write code that checks if the file has data and write that data under the lines you already have.

The Algorithm of the code:-
1. Declare a string variable to store the name of the file to create and write to.
2. Create and open the file in append mode to add new data without deleting old data.
3. Check if the file is empty.
4. If the file is empty, write the headers for the columns.
5. Write the new row of data.
6. Close the file after writing.
Step by step
Solved in 4 steps with 3 images

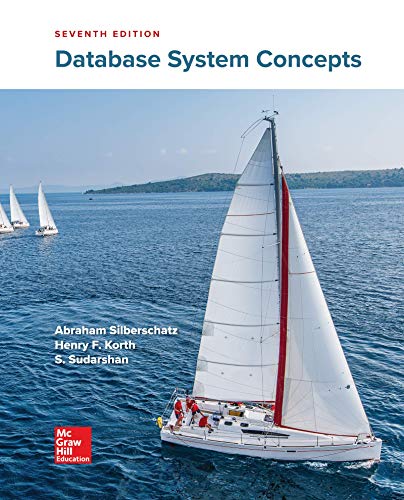
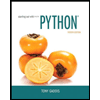
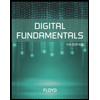
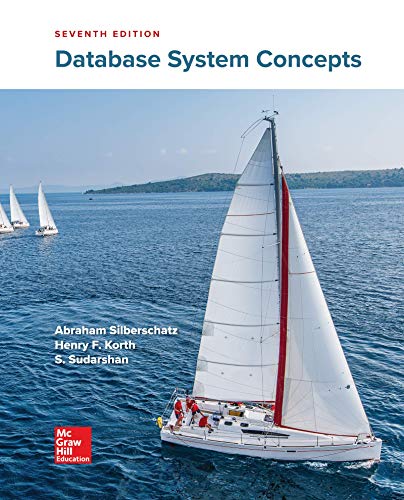
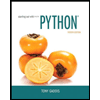
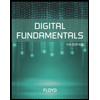
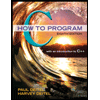
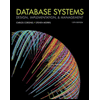
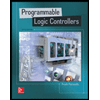