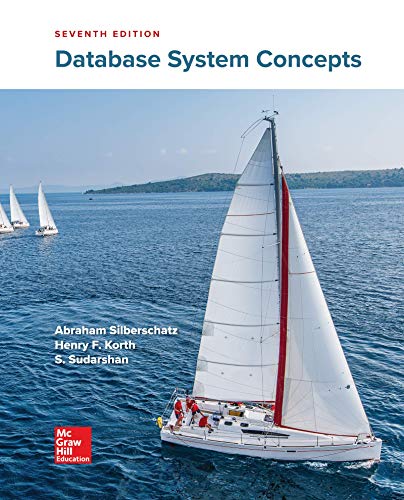
Write a program in C that carries out simple mathematical operations entered as a command-line strings named "calculator".
Implementation:
You will receive a single prefix arithmetic expression in text via argv[1]. You can assume the operands and operators will be space-separated. Test for possibility (i.e. no dividing by zero) and is correctly formatted (i.e. has exactly two operands).
Your operands are: Your operators are: Your errors are:
zero plus Error: not an operator: <text>
one minus Error: not an operand: <text>
two multiply Error: not enough operands
three divide Error: too many elements
four Error: divide by zero
five
six
seven
eight
nine
Common arithmetic expressions with the operator in betwene the operands are called "infix". Prefix notation puts the operand first, so "one plus two" is the infix version and "plus one two" is the prefix version. Be careful to apply context-sensitive operators (like minus and divide) in the correct direction, for example "2 - 1" in infix is equivalent to "minus two one" in prefix and not "minus one two"
You can presume you'll have a single simple expression. There will be no subexpressions, so every expression should have exactly three words: an operator followed by two operands.
Once you decode the expression, you should compute it and output the result in double precision (%2.2ld) and return 1.
If you find an error, you should print an error message and return -1.
e.g.
./calculator "plus four five"
9.00
./calculator "divide one four"
0.25
./calculator "five plus four"
Error: not an operator: two
./calculator "divide three zero"
Error: divide by zero
./calculator
Error: not an operator:
./calculator "plus two one six"
Error: too many elements

Step by stepSolved in 2 steps with 3 images

- Write a function in JAVA language that accepts three integer parameters and returns true if two or more of them (integers) have the same rightmost digit. The integers are non-negative. Inside the main function test the function for at least two test cases.arrow_forwardWrite a function to check three given integers and return their sum. However, If one of the values is the same as another of the values, then both the values are not counted in the sum. In Ruby language.arrow_forwardWrite code in C++, C# or Python to solve the following problem: Instead of a regular Fibonacci number, you are supposed to calculate a special one as follows: F(n) = F(n-1)+ 2 * F(n-2) + 3 * F(n-3) As an example, if F(0) = F(1) = F(2) = 1, then we have: F(3) = F(2) + 2 * F(1) + 3 * F(0) = 1 + 2 + 3 = 6 F(4) = F(3) + 2 * F(2) + 3 * F(1) = 6 + 2 + 3 = 11 Given F(0), F(1), F(2), and N, your job is to calculate F(N). Input Format First number is F(0), second number is F(1), third number is F(2), and last number is N. Example input: 1 1 1 4 Constraints NA Output Format Print the Nth special Fibonnaci number. Example output: 11 Sample Input 0 1 1 1 4 Sample Output 0 11arrow_forward
- Write a program in C programming language that takes two arguments at the command line, both strings. The program checks to see whether or not the second string is a substring of the first (without using the substr -- or any other library -- function). One caveat: any * in the second string can match zero or more characters in the first string, so if the input were abcd and the substring was a*c, then it would count as a substring. Also, include functionality to allow an asterisk to be taken literally if preceded by a \, and a \ is taken literally except when preceding an asterisk.arrow_forwardWrite a simple calculator program in C++. Your program should: read in the operator symbol (+, -, *, /) as a char read in two decimal numbers (type double) output the result of performing the requested operation on the given numbers format the output as in the given example HINT: Use a switch statement instead of conditionals. For example: Input Result + 5.5 2.01 5.5 + 2.01 = 7.51arrow_forwardWrite the following function in Lisp: a function that takes one parameter that you can assume to be a symbol named after a day of the week (no need to check it), such as 'monday, and returns the symbol for the next day. For example, (next-day 'tuesday) should return the symbol 'wednesday. Implement this function with a complex conditional.arrow_forward
- Write a C++ Program, using functions, that, given a number greater than zero (0), the user will choose to calculate one of the following values: the fibonacci series up to that number, where any number in the sequence is the sum of the previous two numbers: the sum of the numbers up to that number, given by the equation: the inverse square series up to that number, given by the equation: the Sum of alternating sign Squares up to that number, given by the equation the Sum of the factorial to the power of the number, given by the equation: Each option should have its own function. A function can call another function. Validate your input.Hint: Use loops and static local variables if you need them.arrow_forwardWrite in C++ please.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
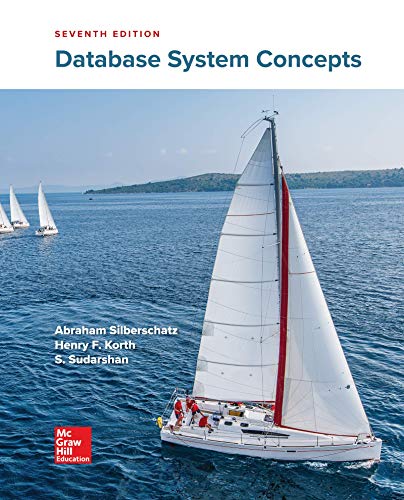
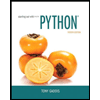
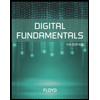
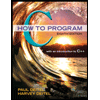
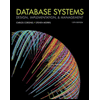
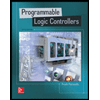