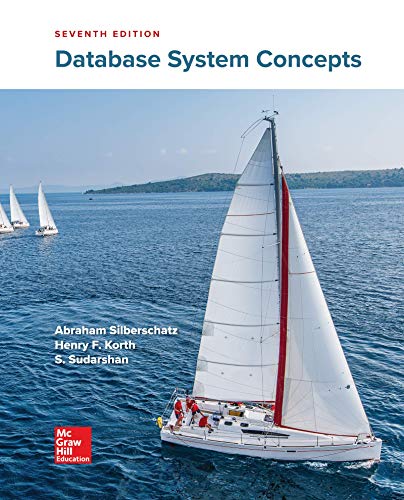
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Java- Please don't change anything like method, don't use scanner. Do exacty the following way:
Write a method named getSumOfRange that accepts two integer parameters min and max and returns the sum of the integers from min through max inclusive. For example, the call of sumOfRange(3, 7) returns 25, which is 3 + 4 + 5 + 6 + 7. If max is less than min, return 0.
Note that getStartOfRange is declared public so that the unit test code can call it
import acm.program.*;
public class SumOfRange extends CommandLineProgram {
public void run()
{
// You may put code here to help with your debugging,
// but it isn't tested.
}
public int getSumOfRange(int start, int end)
{
// Your code here.
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Need help with following Java method: import java.util.Scanner; import java.lang.Math; public class CosineFromScratch { /* The following code demonstrates how to estimate the cosine of a number. Your assignment is to migrate the calculation of the cosine into a new static method named cosine. The method should take one double input and return one double output. Make sure to call the method from main and set the variable estimate equal to the returned value. */ public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("\nGive me a number: "); double x = input.nextDouble(); //Trying to find cosine of this number double correct_cos = Math.cos(x); //START HERE: The following code goes into //the method. //Source for the following: //https://austinhenley.com/blog/cosine.html double num_terms = 10; int div = (int)(x / Math.PI); x = x - (div * Math.PI); int sign = -1; double estimate = 1.0;…arrow_forwardWrite a method on elicpse, computeFutureValue, which receives the investment amount, annual interest rate and number of months as parameters and does the following: Prints the interest amount earned each month and the new value of the investment (hint: use a loop). Sample Program runningEnter the investment amount10000Enter the annual interest rate5.75Enter the number of months18Monthly Interest and Investment valueMonth Interest Value===== ======== =====1 $47.92 $10,047.922 $48.15 $10,096.06 3 $48.38 $10,144.444 $48.61 $10,193.055 $48.84 $10,241.896 $49.08 $10,290.977 $49.31 $10,340.288 $49.55 $10,389.829 $49.78 $10,439.6110 $50.02 $10,489.6311 $50.26 $10,539.8912 $50.50 $10,590.4013 $50.75 $10,641.1414 $50.99 $10,692.1315 $51.23 $10,743.3716 $51.48 $10,794.8417 $51.73 $10,846.5718 $51.97 $10,898.54The total interest earned in 12 months is $898.54arrow_forwardWrite a method (with a parameter n), n: integer type that prints numbers from 1 to n. The print out should be 5 numbers per line. All the output numbers should be aligned well. Call the method with n=13 and n=10 in the main()arrow_forward
- We will rewrite the Sales Bar Chart question to use method for printing out the bar chart. The part where the asterisks are printed out should be done with a method. Use this method for each bar. The original question statement is here: Write a program that asks the user to enter today’s sales for five stores. The program should display a bar chart comparing each store’s sales. Create each bar in the bar chart by displaying a row of asterisks. Each asterisk should represent $100 of sales. Here is an example of the program’s output: Enter today's sales for store 1: 1000 [Enter] Enter today's sales for store 2: 1200 [Enter] Enter today's sales for store 3: 1800 [Enter] Enter today's sales for store 4: 800 [Enter] Enter today's sales for store 5: 1900 [Enter] SALES BAR CHART Store 1: ********** Store 2: ************ Store 3: ****************** Store 4: ******** Store 5: *******************arrow_forwardThe method convertVolume() has an integer parameter. Define a second convertVolume() method that has two integer parameters. The first parameter is the number of gallons and the second parameter is the number of pints. The method should return the total number of pints. Ex: If the input is 9 2, then the output is: 9 gallons yields 72 pints. 9 gallons and 2 pints yields 74 pints. Note: The total number of pints can be found using (gallons * 8) + pints. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class VolumeMethods { publicstaticintconvertVolume(intgallons) { returngallons*8; } /* Your code goes here */ publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intgallonsUsed; intpintsUsed; inttotalPints1; inttotalPints2; gallonsUsed=scnr.nextInt(); pintsUsed=scnr.nextInt(); totalPints1=convertVolume(gallonsUsed);arrow_forwardWrtie TWO methods pass number of birds and print number of birds in California (method1) and birds in Texas (Method 1) Print the total of birds. You can use void method.arrow_forward
- Write a program in Eclipse called LoopMethod (main method) that contains a method called printNums (method name) that prints the numbers from a specified start to end value.You need to use if method.arrow_forwardJava Programarrow_forwardDefine a method printDate, with int parameters year and day, and String parameter month, that prints using Gregorian "Month DD, YYYY" format. For example, printDate (2020, 21, "October") prints October 21, 2020. Write the code of the method in the box below.arrow_forward
- please give me code and output with following direction. thanks!arrow_forwardJava Code with comments and output screenshot is must for an Upvote. Thank you!!!arrow_forwardCreate a method that takes a value and indicate whether it is positive or negative by a return aBoolean value.Declared as: boolean is Positive (float a) in javaarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
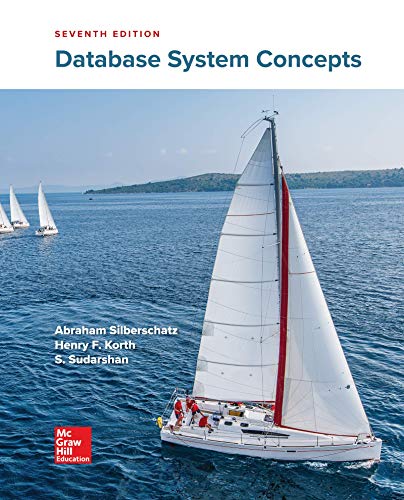
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
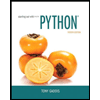
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
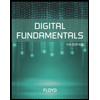
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
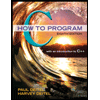
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
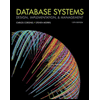
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
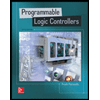
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education