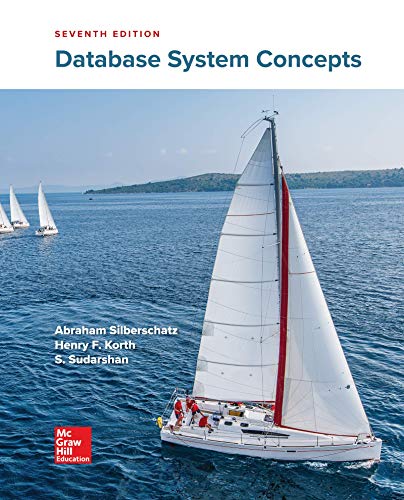
Write a loop-based menu driven complete C++ application (that will run without errors or warnings!) that uses an input file to process the external file, "BankAccounts.txt". The application will continue to run until the loop termination condition is selected. Prior to entering the loop, the application must read all records from the file. Within the loop the application must display a menu that gives the user the option to call one of the “Display...” functions or exit the loop. After the loop, the application will output the total balance of all the accounts (i.e., total for all savings, checking and money market accounts).The external file BankAccounts.txt includes a record for each account and contains the following information (each field separated by colon) Customer ID First Name Last Name Street Address Check Account Balance Money Market Balance Saving Account Balance The application must read the file of records and store the information in an array of structures (maximum size of the array will be 100 entries). Therefore, the application must be designed to support a file that contains a maximum of 100 accounts. The number of accounts must be specified as a constant declaration. This constant must be used in the code everywhere the size of the array is required. The application must have functions that perform the following. DisplayAccounts – displays all the account records, sorted by Customer ID. This is a void function with 2 formal parameters (i.e., array of structures & number of array elements) DisplaySavingsAccounts – displays all the account records with a savings account balance greater than 0, sorted by Customer ID. This is a void function with 2 formal parameters (i.e., array of structures & number of array elements) DisplayCheckingAccounts – displays all the account records with a checking account balance greater than 0, sorted by Customer ID. This is a void function with 2 formal parameters (i.e., array of structures & number of array elements) DisplayMoneyMktAccounts – displays all the account records with a money market account balance greater than 0, sorted by Customer ID. This is a void function with 2 formal parameters (i.e., array of structures & number of array elements) TotalBalanceAccounts – calculates the total of all account balances for all customer savings, checking and money market. The function declaration is below. double TotalBalanceAccounts (double arr[], int accts); Note: Initialize all member variables. 1. The application must read all records of the file by using the technique of testing for the end-of-file (i.e., cannot prompt user for the number of records in the file). In addition, the application must be designed to handle strings that contain spaces. 2. The “Display...” functions are only required to display the pertinent money values (e.g., “DisplaySavingsAccounts” does not display money market or checking amounts).

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- In c++ write a code that tells a user to enter a filename and read that text file line by line.arrow_forwardTraceback (most recent call last): File "C:/app.py", line 20, in main() File "C:/app.py", line 17, in main print_report() File "C:/app.py", line 12, in print_report print_id() File "C:/app.py", line 6, in print_id message = "Your id is:" + id num TypeError: must be str, not int Process finished with exit code 1 Given this stack trace above, on what line was the exception raised? O Line 17 O Line 12 O Line 6 O Line 20arrow_forwardC Sharp Random Number File writer and Reader Create an application that writes a series of random numbers to a file. Each random number should be in the range of 1 through 100. The application should let the user specify how many randome numbers the file will hold and should use a SaveFileDialog control to let the user specify the file's name and Location. Create another application that uses an OpenFileDialog control to let the user select the file that was created above. This application should read the numbers from the file, display the numbers in a ListBox control and display: the total of the numbers and the number of random numbers read from the file.arrow_forward
- In c++, using STL containers, iterators, or algorithms use the movies.txt file attached to get the total for the customer to watch their movie. Console Movies program COMMAND MENU command: total cout << "What movie would you like to watch?"; cin>>Bob and the Horses cout << "Do you want to add a drink ? Y/N"; cin>> N; cout << "Do you want to add popcorn?Y/N"; cin>>Y; cout << " Your price will be" << total << "for everything"; The total should be $17.50 for the Popcorn and movie. ----------------------------------------------------------------------------------------- movies.txt file provided: The Adventures of a Babysitter $10 Barney $25 Bob and the Horses $12 Larry the Car Man $15 Dora and the missing map $15 Ceasar and the Lunch Lady $10 Suzie and the Bad Kids $13.95 Popcorn $5.50 Drink $3.75arrow_forwardThis is a C++ program, to be done in a .cpp file Problem Statement: Write rules for the user on how to play the game in a text file. Then, create a program that will read in the text file and display it out to the user. LCR Game Rules: The Dice● There are three dice rolled each turn. Each die has the letters L, C, and R on it along with dots on the remaining three sides. These dice determine where the player’s chips will go.○ For each L, the player must pass one chip to the player sitting to the left.○ For each R, the player must pass one chip to the player sitting to the right.○ For each C, the player must place one chip into the center pot and those chips are now out of play.○ Dots are neutral and require no action to be taken for that die.The Chips● Each player will start with three chips.● If a player only has one chip, he/she rolls only one die. If a player has two chips left, he/she rolls two dice. Once a player is out of chips, he/she is still in the game (as he/she may get…arrow_forwardin c# i need to Write the program named DirectoryInformation that allows a user to continually enter directory names until the user types end. If the directory name exists, display a list of the files in it; otherwise, display the following message, Directory foo does not exist, to indicate the directory does not exist (foo would be the name of the directory to be checked) . Create as many test directories and files as necessary to test your program. An example of the program is shown below: Enter a directory >> lorem lorem contains the following files lorem/ipsum.txt Enter another directory or type end to quit >> foo Directory foo does not existarrow_forward
- In C++ I am opening a file through a command argument and reading through it. How do you write to the file that would be opened?arrow_forwardThe general form of a Java while loop is : while(___?___) { statements to execute } _____ expression goes in the blank with the "?" mark beside the Java keyword while.arrow_forwardThe header record of a batch file contains totals of items in the file. Each time the file is processed, the totals are also updated. Nightly, after the batch processes, the relevant data fields are summed and compared with the totals. Unbalanced conditions are reported and corrected. This example describes: a) Segregation of duties b) Application output control c) Application edit check d) Applicaiton input controlarrow_forward
- File redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forwardSummary In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. Instructions Make sure the file MovieGuide.py is selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 must be written with this code: """ MovieGuide.py This program allows each theater patron to enter a value from 0 to 4 indicating the number of stars that the patron awards to the Guide's featured movie of the week. The program executes…arrow_forward#function read file and display reportdef displayAverage(fileName):#open the fileinfile = open(fileName, "r")#variable declaration and initializationtotal = 0count = 0#read filefor num in infile:total = total + float(num)count = count + 1#close the fileinfile.close()#calculate averageaverage = total / count#display the resultprint("Average {:.1f}".format(average)) #method callingdisplayAverage("numbers.txt")arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
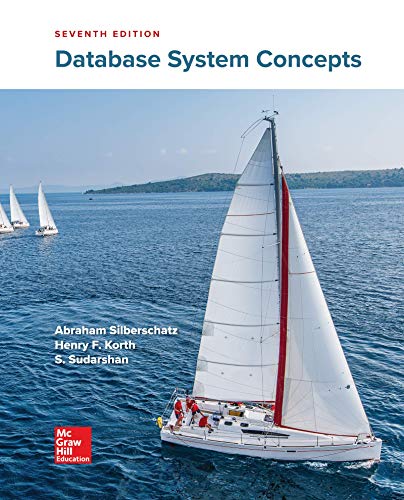
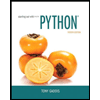
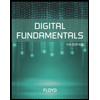
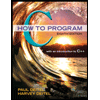
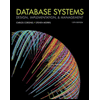
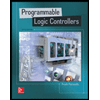