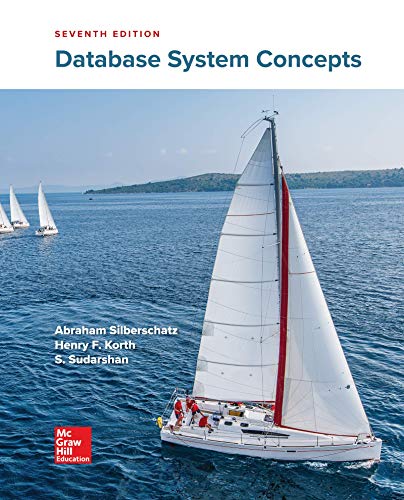
4. Write a java
a. Prompt the user to enter a password (a string, use Scanner class to read the password)
b. Check the validity of the password by calling a method, named passValidity()
c. Return an appropriate message (i.e. password is valid or invalid) to the main() method. The
main() method will print the message.
The passValidity() method will:
a. take the password as the input parameter,
b. check the validity as per the following rule:
• the password string must have at least eight characters
• the password must have at least one alphabet
• the password must contain at least one digit
• the password must contain at least one special character from @, #, $, and &
c. return an appropriate message to the main() method.
Sample output (assuming the input is ab459#de0):
The password is valid
Sample output (assuming the input is abcd#de&ui):
The password is invalid

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Write a Java method to check whether a string is a valid password. Password rules:A password must have at least ten characters.A password consists of only letters and digits.A password must contain at least two digits.arrow_forwardCreate a Java program that contains a String that holds your favorite inspirational quote. Pass the quote to the calculateSpaces method and return the number of spaces to be displayed in the main method. public class CountSpaces { public static void main(String[] args) { // write your code here } public static int calculateSpaces(String inString) { // write your code here } }arrow_forwardIn Java for zybooks ,Lab 3.27, Write a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first six letters of the first name, followed by the first letter of the last name, an underscore (_), and then the last digit of the number (use the % operator). If the first name has less than six letters, then use all letters of the first name.arrow_forward
- Write a java program called Sales considering the following directions and the sample run. Practice here first the write answer on the answer sheet. Also, make sure to distinguish high and low caps as Java is case - sensitive. - import and declare a Scanner class - declare three floating – point variables named price, discount, and discountPrice - ask the user for the price (print statement) and get the price (Scanner) from the user - calculate the discount if price is less than 100, then the discount is 10% of price, else discount is 14% of price - print the discounted prices for the item Sample Run Enter the price 90.0 The discount price is 81.0 Enter the price 200.0 The discount price is 172.0arrow_forwardIn Java please. Only aswer the code where it says "/* Your code goes here */". Write a method lastNameFirst that takes a string containing a name such as "Harry Smith" or "Mary Jane Lee", and that returns the string with the last name first, such as "Smith, Harry" or "Lee, Mary Jane". import java.util.Scanner; public class Names{ /** Changes a name so that the last name comes first. If name has no spaces, it is returned without change. @param name a name such as "Mary Jane Lee" @return the reversed name, such as "Lee, Mary Jane". */ public static String lastNameFirst(String name) { /* Your code goes here */ } public static void main(String[] args) { Scanner in = new Scanner(System.in); String name = in.nextLine(); System.out.println(lastNameFirst(name)); }}arrow_forwardBuild a java program that checks on a user who tries to change current password by asking some questions that user saved their answers while creating the account as shown below. The questions below use 5 different String class methods as follows: • What is your username? o Check if it's the same name saved regardless of capital/small letters • What is your password? o Check if it is written exactly the same as saved value • What is your favorite city? o Check if the city has o letter in middle • What is your brother age? o Compare the brother age to 20 • What is your luck number? o Check if the numeric value is > or <0 Note: customize the answers of ql & 2 as you prefer. Sample Runs (Suceess & Fail Scenarios):arrow_forward
- 3. Consider how a Java program reads input from the keyboard. O O Give three methods from the Scanner class that you can use to read input from the keyboard with Scanner sc = new Scanner(System.in); Which method is used to read an integer with the scanner sc in 3a? Can you use the Scanner class to read an integer twice from the keyboard with the method you identified in 3b? If so, give the code that will allow that to happen. If not, explain what your program should do to use that word multiple times. When mixing Scanner methods in a program (i.e., calling different methods consecutively), which Scanner method will appear to read nothing from the keyboard if following another Scanner method? (If you do not know, put a question mark and move on). Consider what happens when your Java program writes output to the monitor (console). Give two methods that you can use to write output to the monitor.arrow_forwardplease use java to answer the following questionarrow_forwardStart with the code below and complete the getInt method. The method should prompt the user to enter an integer. Scan the input the user types. If the input is not an int, throw an IOException; otherwise, return the int. In the main program, invoke the getInt method, use try-catch block to catch the IOException. import java.util.*; import java.io.*; public class ReadInteger{ public static void main() { // your code goes here } public static int getInt() throws IOException { // your code goes here } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
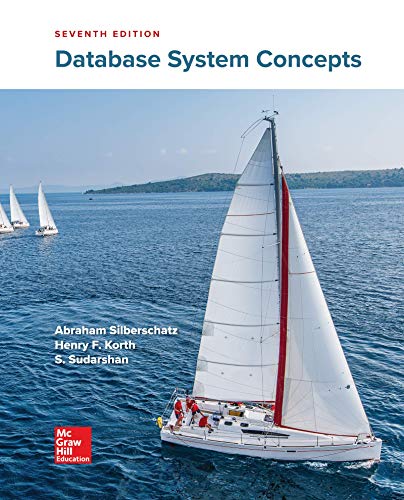
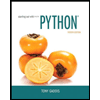
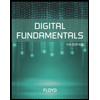
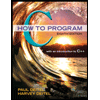
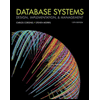
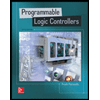