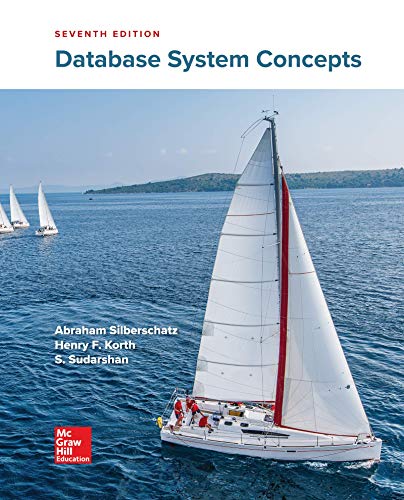
Write a java program in which
A class named Processor has
Two public attributes i.e. price and speed (float value like 2.8, 3.2 etc in MHz)
A parameterized constructor to initialize attributes with user-defined values
Class MainMemory consists of
Two public attributes i.e. size (4,8 etc in Gb) and price
A parameterized constructor to initialize attributes with user-defined values
Class MotherBoard has
a private data member named compName of type string
a no-argument or default constructor to initialize with default name intel
setter function setCompName() to set the name of the computer
getter function getCompName() to get the name of the computer
Class Monitor has
a private data member named size (17, 19 inches) of type Int that represents size of screen
A parameterized constructor to initialize attribute size.
setter function setMonitorSize() to set the value to size.
getter function getMointorSize() to get the size of screen.
public remove() method, that will change the size of screen to zero (0)
Design a class named Computer that includes
An array of type Processor. containing all the processors.
A data member named ram of type MainMemory
A data member named mboard of type MotherBoard
A data member named screen of type Mointor
A parameterized constructor that accept Three arguments of type string as computer Name,
Array of all the Processors and type MainMemory to initialize members of these types.
Moreover, within this constructor, instantiate object of Monitor class with default screen size of
15 inches.
A public setter function setMointor() that can change the size of monitor. if monitor size is set to
Zero i.e 0. it's means that monitor does not exist
A display() function that print complete computer specification. like
Computer: intel with monitor size 17 inches
CPU(s): 1.8, 3.2, MHz
RAM: 8 Gb
A Computer can't have more than 4 processors. in case of more than 4 processors, it should
display error message. and also we all know that Computer can exist without monitor.
Draw UML diagram for each class and show aggregation and composition relationship between these
classes. You are also required to show cardinality in UML diagram, submit your design in PDF format.
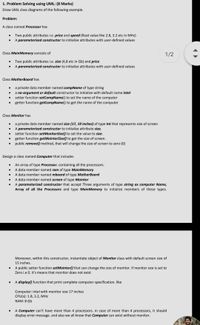

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Design a Java interface called Lockable that includes the following methods: setKey, lock, unlock, and locked. The setKey, lock, and unlock methods take an integer parameter that represents the key. The setKey method establishes the key. The lock and unlock methods lock and unlock the object, but only if the key passed in is correct. The locked method returns a boolean that indicates whether or not the object is locked. A Lockable object represents an object whose regular methods are protected: if the object is locked, the methods cannot be invoked; if it is unlocked, they can be invoked. Redesign and implement a version of the Coin class from Chapter 5 so that it is Lockable.[code]//********************************************************************// Coin.java Author: Lewis/Loftus//// Represents a coin with two sides that can be flipped.//********************************************************************public class Coin {private final int HEADS = 0;private final int TAILS =…arrow_forward1. Design a Java JFood class for a food which implements both cloneable and comparable interfaces The class should have the following private member variables: mId: an integer that holds the food ID mName: a string that holds the food name mQuantity: an integer that holds quantity of servings of food mCalory: a double that holds calory of one serving of food mIngredients: an array of String that holds the names of all ingredients and its size is three and the class should have the following public member functions: default constructor: initializes the product to some default one constructor #2: accepts id, name, quantity, calory and ingredients as arguments. The method should set these values to the appropriate instance variables setFood: accepts quantity, calory and ingredients as arguments. The method should set the arguments to the appropriate instance variables. Please note ingredients need be set as deep copy. Five respective get functions to retrieve the five instance…arrow_forwardThe internet, as defined by Wikipedia, "is a computer network that connects billions of computing devices worldwide." [Reference required] Please provide a brief description of the following three elements, as well as the part each of them plays in facilitating communication over the internet, using the "nuts and bolts" technique.arrow_forward
- Define a Java class named Printer that has the following 1. Four properly encapsulated variables: model of type String, speed of type double, price of type double, and year of type int. 2. A no-arg constructor that sets model to "Co 1", speed to 10.5, price to 310.7, and year to 2021. 3. A constructor that takes four parameters and sets the four instance variables accordingly. 4. A getter and a setter method for the instance variable price. 5. An instance method named toString that does not take any parameter and returns a value of type String. The returned value contains the four instance variables of this object in a format of your choice. Paragraph BIU- ...arrow_forwardIn Java programming. Write the code to create an object of each type of class. The names of the objects created in this part will be used for further requirements in this phase. Class Name: Customer Class Name: Inventory Class Name: OrderHeader Class Name: OrderDetail Class Name: Invoice Class Name: Shipment Four lines of code, one for each class defined above.arrow_forwardJAVA:arrow_forward
- Write a Java program A2p1.java with a public class A2p1 and no named packages to read from stdin information for several singers and dancers. You should design a superclass Person with appropriate constructor(s) to initialize member variables such as name (of type String), sex (of type String) and age (of type int). You should design two additional subclasses Singer and Dancer of the Person class with appropriate constructor(s) to initialize additional member variables such as favorite (of type String). You can add the toString method to these three classes to provide a suitable string representation for objects of these classes. Put all four classes in the same file. A sample run can look like the following (you can also try running it with standard input redirection): [~/temp] $ java A2p1 [kwang@computer] How many persons do you want to input? 3 Enter information for person 1: Name: Tom Sex ('F' or 'M') : M Age: 18 Singer or Dancer ('S' or 'D'): S Favorite song: songl Enter…arrow_forwardExplain through each question about Classes and Objects in Java. What is encapsulations and why is it useful? What is an instance method and how does it differ from the static methods we learned previously? What is a mutator method? What is an accessor method? What is a constructor in a Java class? What is an implicit parameter? Please provide an entire coding example to illustrate the answer.arrow_forward1.Abstract classes, 2.abstract methods 3.Interfaces are three important OOP concepts. Give an example where each would be appropriate to usearrow_forward
- in java language... Draw the UML diagram of the following class: public class Employee { String name; double salary; public Employee() { } public double getSalary() { return this.salary; } public String getName() { return this.name; } }arrow_forwardwrite a program in JAVA 4. Design and write a class to represent a bank account that includes the following members: a. Data members Owner name Account number Balance amount in the account b. Methods members To assign initial values To deposit an amount To withdraw an amount after checking balance To display the owner name and balancearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
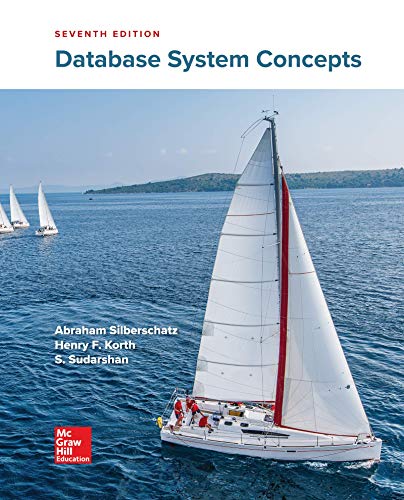
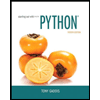
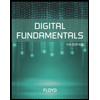
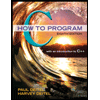
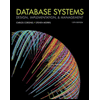
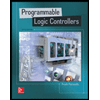