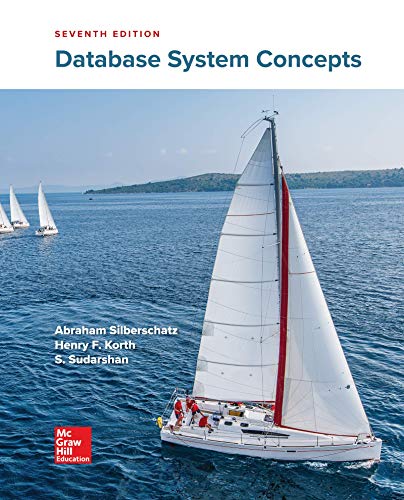
9.12: Element Shifter C++
Write a function that accepts an int array and the array’s size as arguments. The function should create a new array that is one element larger than the argument array. The first element of the new array should be set to 0. Element 0 of the argument array should be copied to element 1 of the new array, element 1 of the argument array should be copied to element 2 of the new array, and so forth. The function should return a pointer to the new array. Demonstrate the function by using it in a main
Prompts And Output Labels. There are no prompts for the integer and no labels for the reversed array that is printed out.
Input Validation. If the integer read in from standard input exceeds 50 or is less than 0 the program terminates silently.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

-
The function should create a new array that is twice the size of the argument array.
-
The function should copy the contents of the argument array to the new array, and initialize the unused elements of the second array with 0.
-
The function should return a pointer to the new array.
-
The function should create a new array that is twice the size of the argument array.
-
The function should copy the contents of the argument array to the new array, and initialize the unused elements of the second array with 0.
-
The function should return a pointer to the new array.
- I/ implement copyToVec such that: // 1) receives three parameters, an array, the array's size, and a vector // 2) copy elements from the array to the vector. Copy elements from 0 to size-1 // 3) using the RANGE-BASED for Loop ONLY, add 0.9 to every element in the vector /* implement main such that: 1) crate an array of size 100 and type float 2) prompt the user to enter 50 values 3) create a new instance of type vector that can store float values 4) invoke copyVec using this exact statement: copyToVec(arrFloat, 30, vecFloat); 5) Using the RANGE-BASED for Loop. print the values from the vector such that that the output is formatted as shown below. Use the following input test data: 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 vecFloat[0] vecFloat[1] = 1.290e+01 vecFloat[2] vecFloat[3] vecFloat[4] vecFloat[5] = 1.690e+01 vecFloat[6] = 1.790e+01 vecFloat[7] vecFloat[8] = 1.990e+01…arrow_forward[ ] [] power In the cell below, you are to write a function "power(list)" that takes in a list as its input, and then returns the power set of that list. You may assume that the input will not have any duplicates (i.e., it's a set). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (power ( ["A", "B"]), power ( ["A", "B", "C"])) + Code + Markdown This should return [[], ["A"], ["B"], ["A", "B"]] [[], ["A"], ["B"], ["C"], ["A", "B"], ["A", "C"], ["B", "C"], ["A", "B", "C"]] (the order in which the 'sets' appear does not matter, just that they are all there) Python Pythonarrow_forwardUsing C++ complete the following program 10.18: Phone Number ListWrite a program that has an array of at least 50 string objects that hold people’s names and phone numbers. The program then reads lines of text from a file named phonebook into the array. The program should ask the user to enter a name or partial name to search for in the array. All entries in the array that match the string entered should be displayed-- thus there may be more than one entry displayed. Prompts And Output Labels. The program prints the message "Enter a name or partial name to search for: " and then after the user enters a some input and hits return, the program skips a line, and prints the heading: "Here are the results of the search:", followed by each matched string in the array on a line by itself. Input Validation. None.arrow_forward
- C++ Please help!arrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward9. Write function getMoveRow to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the row portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. 2 is the row iii. 2 is index 1 in the board array e. Return the row array index that corresponds to the player’s move f. Return a -1 if the row is not valid (i.e. INVALID) 10. Write function getMoveCol to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the column portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. b is the column iii. b is index 1 in the board array e. Return the column array index that corresponds to the player’s move f. Return a -1 if the column is not valid (i.e. INVALID) I am getting an error when entering the code at the very end. please fix it. This is the code in C int…arrow_forward
- 5.12 LAB: User-Defined Functions: Adjust list by normalizing CORAL LANGUAGE ONLY PLEASE When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Define a function named getMinimumInt that takes an integer array as a parameter and returns the smallest value. Then write a main program that reads five integers as input, stores the integers in an array, and calls getMinimumInt() with the array as an argument. The main program then outputs the normalized array by subtracting the returned smallest value from all the values in the array. Ex: If the input is: 30 50 10 70 65 function getMinimumInt() returns 10, and the program output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program should define and use a function:Function getMinimumInt(integer array(?) userVals) returns integer minInt…arrow_forward13. Write function updateBoard to do the following a. Return type void b. Parameter list i. 1-d character array (i.e. move), size 3 ii. 2-d character array (i.e. board), size 8 rows and 8 cols iii. Structure Player (i.e. player) as a pointer c. Declare local variable rowInt, data type integer, set equal to function call getMoveRow, passing parameter move as an argument d. Declare local variable colInt, data type integer, set equal to function call getMoveCol, passing parameter move as an argument e. If calling function checkHorizontal (i.e. see argument list in step f.i.) is greater than ZERO, call function updateHorizontal, passing as arguments i. rowInt ii. colInt iii. board iv. player f. If calling function checkVertical (i.e. see argument list in step f.ii.) is greater than ZERO, call function updateVertical, passing as arguments i. rowInt ii. colInt iii. board iv. player g. If calling function checkDiagonal (i.e. see argument list in step f.iii.) is greater than ZERO, call…arrow_forwardComplete the function below. You must not change the fuction signatures, names return types and parametersmust remain as given. Please the code must be in c+arrow_forward
- A1: File Handling with struct C++ This program is to read a given file and display the information about employees according to the type of employee. Specifically, the requirements are as follows. Read the given file of the information of employees. Store the information in an array or arrays. Display all the salaried employees first and then the hourly employees Prompt the user to enter an SSN and find the corresponding employee and display the information of that employee. NOTE : S- salaryemployee , H- Hourly employee S 135-25-1234 Smith Sophia DevOps Developer 70000H 135-67-5462 Johnaon Jacob SecOps Pentester 30 60.50S 252-34-6728 William Emma DevOps DBExpert 100000S 237-12-1289 Miller Mason DevOps CloudArchitect 80000S 581-23-4536 Jones Jayden SecOps Pentester 250000S 501-56-9724 Rogers Mia DevOps Auditor 90000H 408-67-8234 Cook Chloe DevOps QAEngineer 40 45.10S 516-34-6524 Morris Daniel DevOps ProductOwner 300000H 526-47-2435 Smith Natalie DevOps…arrow_forwardcode in Carrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
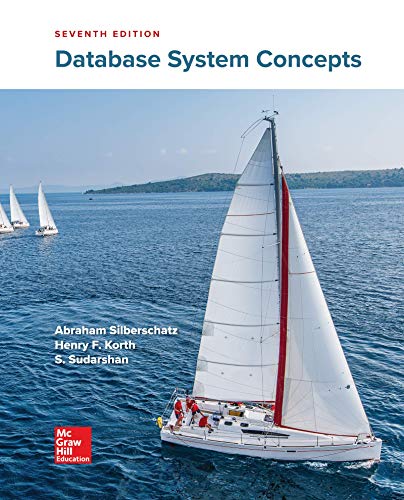
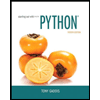
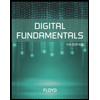
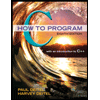
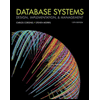
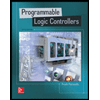