Write a function, ReadFile(), that reads the content of the provided text file “Message.txt” and stores it in an array. The function receives as parameter a pointer to a character array where the content of the text file should be stored.. The number of lines that was read from the text file must be returned to the calling statement. Write a function, ReverseMyString(), that converts the character array (which now contains the contents of the text file) by replacing the first character with the last character, the second character replaced with the second last character, etc, until the message is reversed as shown in the Figure 3. The function receives as parameters, a pointer to the character array and an integer value indicating the length of the array. The function returns a 1 or 0 to indicate success and failure respectively. NB: You must write your own function. The strrev()- function may not be used.
NB: Square bracket notation for array access is NOT allowed for Question 3, i.e. array[i]. Array access MUST be facilitated by the use of pointers.
Write a function, ReadFile(), that reads the content of the provided text file “Message.txt” and stores it in an array. The function receives as parameter a pointer to a character array where the content of the text file should be stored.. The number of lines that was read from the text file must be returned to the calling statement.
Write a function, ReverseMyString(), that converts the character array (which now contains the contents of the text file) by replacing the first character with the last character, the second character replaced with the second last character, etc, until the message is reversed as shown in the Figure 3. The function receives as parameters, a pointer to the character array and an integer value indicating the length of the array. The function returns a 1 or 0 to indicate success and failure respectively. NB: You must write your own function. The strrev()- function may not be used.
Write a function, WriteFile(), which stores information in a text file. The function receives as parameter a pointer to the character array that has to be written to the text file. Name the output file “Output.txt”. The function returns no value to the calling statement.
The above functions (ReadFile(), ReverseMyString(), and WriteFile()) must now be implemented in the main program (main()) in the following order with a fitting message as in Figure 3: 1. Create an array of type character and make provision for a maximum of 100 elements. 2. Read the contents of the text file “Message.txt” to the array with ReadFile(). 3. Call the ReverseMyString() function to reverse the content of the array. 4. Write the reversed character array to an output file with WriteFile().


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

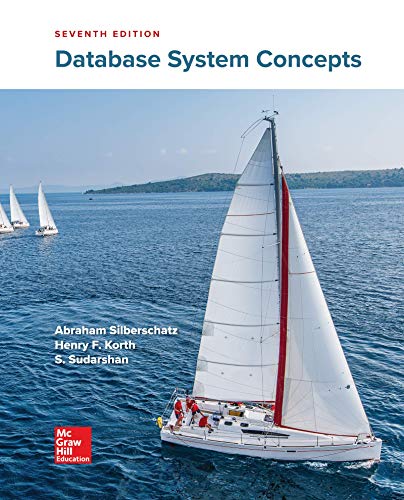
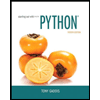
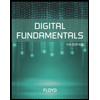
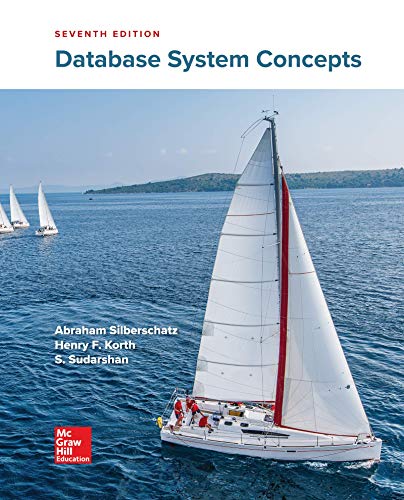
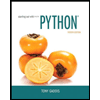
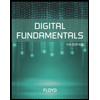
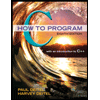
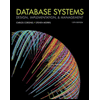
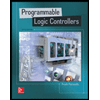