Write a function getSSANum() that takes no parameters, but prompts the user to enter a Social Security number, and returns a string representing a formatted version of the first valid number entered by the user. A valid Social Security Number has precisely nine digits and does not contain any other characters. The formatted version that is returned by the function should look like: xxx-xx-xxxx where the x’s represent the digits entered by the user. The user should be repeatedly re-prompted by the function until they enter a string consisting of precisely nine valid digits. Hint: Assign the information from the user as a string and use string functions (len(str) and string method str.isnumeric(str) to determine if the user input is all numeric) to help you determine if what the user entered has the right format. Note the correct solution to this function will involve a while loop (so check the lecture notes 8-2 to refresh on how while loops operate), because the user is entering a string rather than a numeric value. Also do not use a try-except block in the while loop is not helpful. Don’t forget to include the docstring and comments. Copy and paste or screen shot the code and the eight test cases shown below in your submission. result of code: >>> getSSANum() Please enter a nine-digit Social Security number: ********* That is not a valid number. Please try again. Please enter a nine-digit Social Security number: 888&()280 That is not a valid number. Please try again. Please enter a nine-digit Social Security number: 12345678 That is not a valid number. Please try again. Please enter a nine-digit Social Security number: testtest! That is not a valid number. Please try again. Please enter a nine-digit Social Security number: 787878789 '787-87-8789' >>> getSSANum() Please enter a nine-digit Social Security number: 3.1415926 That is not a valid number. Please try again. Please enter a nine-digit Social Security number: 314159265 '314-15-9265' >>> getSSANum() Please enter a nine-digit Social Security number: 012345678 '012-34-5678'
Write a function getSSANum() that takes no parameters, but prompts the user to enter a Social Security number, and returns a string representing a formatted version of the first valid number entered by the user. A valid Social Security Number has precisely nine digits and does not contain any other characters. The formatted version that is returned by the function should look like: xxx-xx-xxxx where the x’s represent the digits entered by the user. The user should be repeatedly re-prompted by the function until they enter a string consisting of precisely nine valid digits. Hint: Assign the information from the user as a string and use string functions (len(str) and string method str.isnumeric(str) to determine if the user input is all numeric) to help you determine if what the user entered has the right format. Note the correct solution to this function will involve a while loop (so check the lecture notes 8-2 to refresh on how while loops operate), because the user is entering a string rather than a numeric value. Also do not use a try-except block in the while loop is not helpful. Don’t forget to include the docstring and comments. Copy and paste or screen shot the code and the eight test cases shown below in your submission.
result of code:
>>> getSSANum()
Please enter a nine-digit Social Security number: *********
That is not a valid number. Please try again.
Please enter a nine-digit Social Security number: 888&()280
That is not a valid number. Please try again.
Please enter a nine-digit Social Security number: 12345678
That is not a valid number. Please try again.
Please enter a nine-digit Social Security number: testtest!
That is not a valid number. Please try again.
Please enter a nine-digit Social Security number: 787878789
'787-87-8789'
>>> getSSANum()
Please enter a nine-digit Social Security number: 3.1415926
That is not a valid number. Please try again.
Please enter a nine-digit Social Security number: 314159265
'314-15-9265'
>>> getSSANum()
Please enter a nine-digit Social Security number: 012345678
'012-34-5678'

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

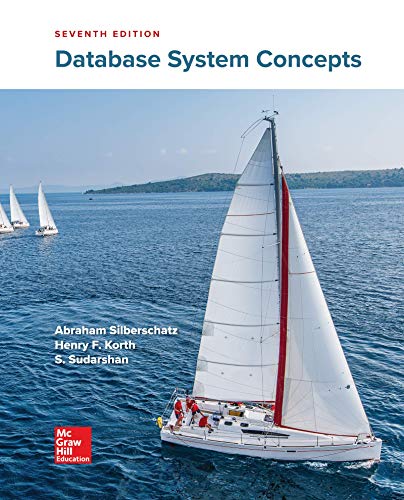
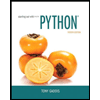
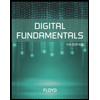
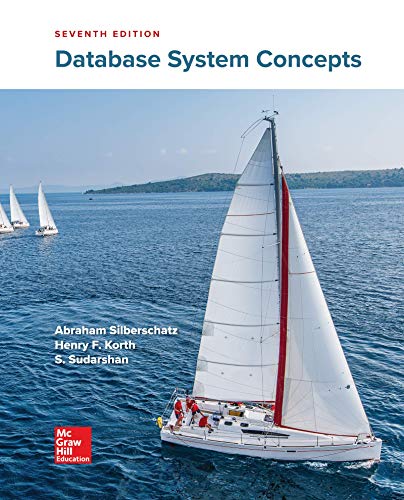
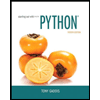
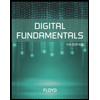
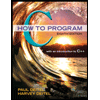
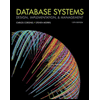
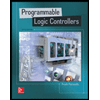