Write a complete C++ program that takes 10 integers from the user and stores them in an array by uing a for loop. The program should find the sum of the integers at even indexes (including index 0), and then multiply each integer in the array with the sum. The program should then print the new values in the array. Sample run: Enter values: 2 517 346 98 10 Sum of the numbers at even indexes is 20 New values in the array: 40 100 20 140 60 80 120 180 160 200
Q: To be more explicit, in what ways are the BIOS and UEFI passwords on certain PCs able to be…
A: Introduction: Basic Input-Output System, or BIOS, is what it stands for. It speaks about the…
Q: ould be the config of a computer (A support a 32-bit word computer sys
A:
Q: How is it necessary to protect the "building blocks" of data communication networks, such as…
A: Solution: It is very necessary to protect the "building blocks " of data communications networks,…
Q: What belongs in the XXX and YYY in the statement below in order to generate a random number between…
A: Random generator = new Random(); generator.nextInt(XXX) - YYY; nextInt() method in Random class will…
Q: JAVA Programming Write a function that returns true if you can partition an array into one element…
A: Algorithm - Take one string as input. Now use the below logic if(count==1) return false;…
Q: on why it's so important that you comprehend compressed z
A: Introduction: As we know The subject of this article is compressed zip files and folders. as it. A…
Q: e a comparison table comparing HTTP and WebSocket difference
A: It is defined as : HTTP : The Hypertext Transfer Protocol (HTTP) is an application-level protocol…
Q: Plotting linear and quadratic equations Write a program graph.py that prompts a user for three…
A: import matplotlib.pyplot as plt import numpy as np x = np.linspace(-5,5,100) y = 2*x+1 plt.plot(x,…
Q: Question 8: The following statement is a right way to link to an e-mail address: Say HELLO!!!. A.…
A: We need to find the correct option regarding html email tag.
Q: what use Linux may have in any element of rocket technology.
A: ANY ASPECTS OF ROCKET TECHNOLOGY COULD USE LINUX - Like NASA, the new waves are necessary. We need…
Q: Give an explanation of how buffer overflow attacks are carried out and what the results of such an…
A: A Buffer Overflow Attack is a kind of attack that exploits the "buffer overflow" vulnerability. A…
Q: Assume the variable myWord references a string. Write a statement that uses a slicing expression…
A: Python is an interactive programming language that is used to make our program easy and used to make…
Q: List the justifications for why a company could want firewalls for physical security measures.
A: Introduction: Physical security refers to protecting an organization's physical assets, such as its…
Q: Assume that after installing a motherboard, someone forgets to connect the wires from the casing to…
A: Numerous wires protrude from the front panel of the computer casing and link to the motherboard's…
Q: Create a function that takes two "sorted" arrays of numbers and returns an array of numbers which…
A: In this problem we need to design the Java script program. Input - that takes two "sorted" arrays…
Q: What is the SDLC?
A: SDLC stands for "Software Development Life Cycle" SDLC is the collection of processes which needs…
Q: Many commands in Linux work exactly as they do in Linux, but why and how does this happen?
A: A Linux command is a program or utility that runs on the command line.
Q: Perform the following operations using the 2's complement method. a. 1100001010111001 b.…
A:
Q: Elaborate on any five advantages of alpha testing.
A: alpha testing Alpha testing is the earliest process of evaluating whether a new product will…
Q: Provide an overview of the built-in antiviral programme for Windows, Windows Defender
A: Microsoft Defender Antivirus Windows Security is built-in to Windows and includes an antivirus…
Q: Assume you need to store book data in a structured manner. Either more books should be added, or…
A: The answer is given in the below step
Q: A computer consists of a processor and an I/O device D connected to main memory M via a shared bus…
A: Given that, on an average, six cycles are required by the instruction.
Q: Consider a fixed partitioning scheme with equal-size partitions of 2¹6 bytes and a total main memory…
A: Fixed partitioning is therefore defined as the system of dividing memory into non-overlapping sizes…
Q: Take into consideration the wide area network, which is your preferred form of wireless…
A: A communication network that connects numerous unique geographic locations utilizing a single…
Q: Trace each pass of selection, insertion, bubble and quick sort for the list of values below. For…
A: The answer is written in step 2
Q: virtual machine (VM) and how does it work? Which benefits may virtual machines provide?
A: Introduction Virtual Machine:- A Virtual Machine, typically shortened to simply VM, is not any…
Q: What is a virtual machine (VM) defined as? Is there a reason I would want to use a virtual machine?…
A: A Virtual Machine, also shortened as VM, is the same as any other physical computer, such as a…
Q: What are the benefits of using a layered architectural approach?
A: Layered architecture is the processing of data that occurs at several levels. Basically, multitiered…
Q: JAVA Problem Create a function that determines whether elements in an array can be re-arranged to…
A: Algorithm - Take one string as input. Now use the below logic return (arr[arr.length - 1] -…
Q: It is said that IPsec may not work with Network Address Translation (NAT) (RFC 1631). However,…
A:
Q: Give specific examples of each method for preventing OS intrusions.
A: Intrusion: An incursion is any activity that breaches network data security to obtain unauthorized…
Q: What other administration strategies for a firewall are you acquainted with? Explain.
A: Here we have given administration strategies for a firewall. You can find the solution in step 2.
Q: What is JVM and is it platform independent?
A: JVM is also called as Java Virtual Machine.
Q: How does the separation between kernel mode and user mode work as a basic security (or protection)…
A: 1) In kernel mode, the program has immediate and unlimited admittance to framework assets. In user…
Q: How should someone continue if they have forgotten both the administrator password and the system's…
A: Introduction: In this question, we are asked to give the solutions if some forgot the password for…
Q: uss the ways in which the benefits of enterprise information architecture contribute to the…
A: As we know Enterprise design promotes organisational effect by increasing efficiency, mobility,…
Q: What is the method that DMA uses to increase the concurrency of the system? In what ways does it…
A: The answer of the question is given below:
Q: There are many problems that wireless networks run into as a result of their inherent…
A: Here are the explanation regarding the wireless network:
Q: JAVA Script | A Happy Year is the year with only distinct digits. Create a function that takes an…
A: Algorithm - Take one integer as input. Now use the below logic if(count==1) return false;…
Q: Define the characteristics that are required for test-driven development. In the event that you…
A: INTRODUCTION: A software development approach called test-driven development keeps track of all…
Q: Consider the context-free grammar (CFG) G = with production P S do S while S | while S do S | & and…
A: ANSWER:-
Q: Think about how cloud computing encourages open innovation.
A: Cloud computing enables a variety of services.This includes consumer services such as Gmail or the…
Q: Build a function that creates histograms. Every bar needs to be on a new line and its length…
A: 1. created the method with 2 arguments,1 array, and a special character 2. create the result string…
Q: What does the presence of hashtags in an Excel column mean?
A: Introduction: There are few reasons are there for the presence of hashtag in Excel spreadsheet. We…
Q: A memory system consists of a cache and a main memory. It takes 25ns to access the cache, and 100ns…
A: Answer the above question are as follows
Q: 1 Turing Machines Produce a Turing Machine state diagram that decides the following language. You do…
A: Here we have given solution to produce a Turing Machine state diagram that decides the following…
Q: What is open source in computer science?
A: In computer science we write codes to make a software program. From simple computer to advanced…
Q: A microprocessor provides an instruction capable of moving a string of bytes from one area of memory…
A:
Q: Explain what is meant by the term "caching" when referring to shared memory
A: The cache is a temporary storage area which can retrieve data easily by a computer processor.…
Q: What does it mean to be "busy waiting"? What other sorts of waiting are there in an operating…
A: What does it mean to be "busy waiting"? What other sorts of waiting are there in an operating…


Step by step
Solved in 4 steps with 2 images

- Write a complete C++ program that takes 10 integers from the user and stores them in an array by using a for loop. The program should find the sum of the integers at even indexes (including index 0), and then multiply each integer in the array with the sum. The program should then print the new values in the array. Sample run: Enter values: 2 5 1 734698 10 Sum of the numbers at even indexes is 20 New values in the array: 40 100 20 140 60 80 120 180 160 200Programming in C language. AskForNumbers Declare an integer array lacally with the size of 200. Create a program that asks the user how many numbers the have. Use your getChoice() function from before. Make sure it does not exceed 200 as the locally declared array has the size of 200. Use a for loop and ask the user to enter each value that must be stored in the array. Use a second loop to display each number, and also determine the average of all values in the array. After the for loop, display the average of all numbers. This program will let you enter a list of numbers into an array. It will then display all of the numbers, and finally display the average of all numbers. How many numbers would you like to enter?5 Please enter a number:22 Please enter a number:33 Please enter a number:44 Please enter a number:55 Please enter a number:66 Number Number 2 is 33 Number 3 is 44 Number 4 is 55 Number 5 is 66 The average is 44 is 22Programming in C language. RandomNumbers Declare an integer array locally with the size of 200. Create a program that asks the user how many numbers the have. Use your getChoicel) function from before. Make sure it does not exceed 200 as the locally declared array has the size of 200. Use a loop to assign random numbers from 1 to 10000 to the array elements. (Remember to use srand() to seed the random number generator). Use a second loop to display each number, and also determine the average of all values in the array. You must also find the largest and the smallest numbers. After the for loop, display the average of all numbers. This program will let you enter a list of numbers into an array. It will then display all of the numbers, and finally display the average of all numbers. How many numbers would you like to enter?10 Number 1 is 42 Number 2 is 8468 Number 3 is 6335 Number 4 is 6501 umber 5 is 9170 Number 6 is 5725 Number 7 is 1479 Number 8 is 9359 Number 9 is 6963 Number 10 is…
- Programing in C Write a program that calculates the average grade of a class. 1. You should ask the user how many students you have (you will use this number to set up the length of your array of decimals). 2. Once you have your array, you will have to ask the user for all the grades (there should only be one grade per student). Using a while loop. 3. While you are scanning the grades, you should check if the inputted grade is in the range 0.0 to 10.0 (inclusive). If the grade is not in this range, you should display a message to the user and assign 0.0 to the grade. 4. Once your array is populated with all student's grades, print out the values of the array, so that the user knows these values were saved correctly (use a while loop as well). 5. After this calculate the average (add up all grades and divide by the number of students). Hint: You will need a loop to do so; please use a while loop as well. Example: How many students are in your class? 8 Please input the grades: 8.5 9.8…Write a C++ program that asks a user to input 10 numbers. The numbers are stored in the array if it is not Odd and not already available. After creating the array, it asks the user to enter a number and deletes it from the arrayin c++ InstructionsWrite a program that prompts the user to enter 50 integers and stores them in an array. The program then determines and outputs which numbers in the array are sum of two other array elements. If an array element is the sum of two other array elements, then for this array element, the program should output all such pairs separated by a ';'. example: list[0] = 15 is the sum of: ----------------------list[1] = 25 is the sum of: list[5], list[14]; list[13], list[47]; list[46], list[47]; ----------------------list[2] = 23 is the sum of: list[0], list[33]; ----------------------list[3] = 65 is the sum of: list[4], list[32]; list[13], list[20]; list[20], list[46]; list[21], list[34]; list[32], list[38]; ----------------------list[4] = 34 is the sum of: list[0], list[14]; list[6], list[48]; ----------------------list[5] = 6 is the sum of: ... If no two elements can equal the value of an element, the line can remain empty, as shown above for list[0] and list[5].
- Answer in C++ Programming Language. C++, Array Write a program that will ask the user to enter a set of numbers and outputs all the subsets of that set. The size of the array is 5. Ask the user if he wants to repeat the program by pressing y/Y.a C++ program that creates a two-dimensional integer array (4x3) initialized with user given data. The program should have the following functions: • Print the sum of all values in the array. • Print the average of all the values in the array. • Take row number from user and print the sum of the values in that specified row.(use loop and no if statement) • Take column number from user and print the sum of the values in that specified column.(use loop and no if statement)In C++ please Write a program to simulate a pick-5 lottery game. Your program should generate and store 5 distinct numbers between 1 and 9 (inclusive) into an array. The program prompts the user to enter five distinct between 1 and 9 and stores the number into another array. The program then compares and determines whether the two arrays are identical. If the two arrays are identical, then the user wins the game; otherwise the program outputs the number of matching digits and their position in the array. Your program must contain a function that randomly generates the pick-5 lottery numbers. Also, in your program, include the function sequential search to determine if a lottery number generated has already been generated.
- For C++: Design an application that has an array of at least 20 integers. It should call a module that uses the sequential search algorithm to locate one of the values. The module should keep a count of the number of comparisons it makes until it finds the value. Then the program should call another module that uses the binary search algorithm to locate the same value. It should also keep a count of the number of comparisons it makes. Display these values on the screen.in c++ vs2019 You should write a program to sort an array. For this purpose you will implement two sorting algorithms: Insertion Sort and Merge Sort. For each algorithm, you are supposed to have a counter and count how many times a comparison is made to sort the array. Once this logic is implemented, create integer arrays of 1000 and 10000 elements with random values inside (you can implement a random number generator for this purpose and randomize their elements). Then go ahead and run your application. The output should show us how many comparisons it made for each algorithm for 1000 and 10000 elements. Do it seperate files. 1 cpp file 1 header file 1 main.cpp fileWrite a C++ program that asks the user to enter 10 integer values in an array. After entering 10elements arrange in ascending order and display it on output screen.Sample Input:Please enter elements for array:10 8 5 9 14 7 12 10 1 2
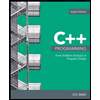
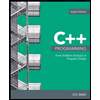