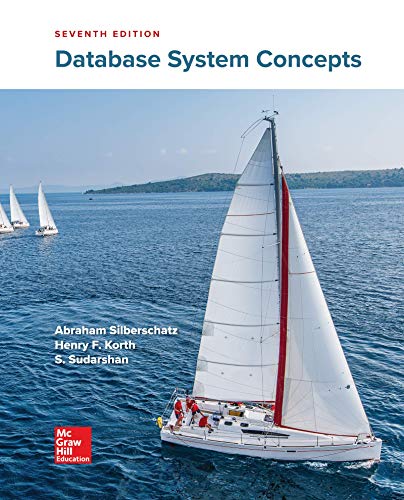
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
In C++
Write a class, named "TwoOrLess" with a header file (two_or_less.hpp) and an implementation file (two_or_less.cpp).
This is a class that acts much like a set, except it can hold 0, 1, or 2 duplicates of an int. You need to support the insert, count, and size methods with the same parameters and return types as the set<int> class (see test cases).
![### Code Sample
```cpp
#include "two_or_less.hpp"
TwoOrLess thing;
ASSERT_EQ(thing.size(), 0);
thing.insert(0);
ASSERT_EQ(thing.size(), 1);
```
### Your Code's Output
```plaintext
from /usr/include/gmock/internal/gmock-internal-utils.h:47,
from /usr/include/gmock/gmock-actions.h:51,
from /usr/include/gmock/gmock.h:59,
from cpp_unit_test:0:
cpp_unit_test:3:11: error: ‘thing’ was not declared in this scope
ASSERT_EQ(thing.size(), 0);
^~~~~
cpp_unit_test:3:11: note: suggested alternative: ‘this’
cpp_unit_test:4:1: error: ‘thing’ was not declared in this scope
thing.insert(0);
^~~~~
cpp_unit_test:4:1: note: suggested alternative: ‘this’
CMakeFiles/runTests.dir/build.make:62: recipe for target 'CMakeFiles/runTests.dir/tests.cpp.o' failed
make[2]: *** [CMakeFiles/runTests.dir/tests.cpp.o] Error 1
```
### Explanation
The code attempts to include and use a class or header called `TwoOrLess` from the `two_or_less.hpp` file. This snippet is supposed to perform unit tests using Google Mock, indicated by the `ASSERT_EQ` checks.
**Errors Identified:**
1. **Undeclared Variable:**
- The error message `‘thing’ was not declared in this scope` indicates a missing declaration or inclusion issue. The compiler suggests the alternative `‘this’`, which is not relevant in this context.
2. **Build Error:**
- The build is terminated with an error in the CMake process, implying that the compilation of `tests.cpp.o` failed due to the unresolved issues in the above code.
### Recommendations for Resolution:
- **Ensure `TwoOrLess` is Defined:**
- Verify that the `two_or_less.hpp` file is correctly included and that it defines the `TwoOrLess` class.
- **Check for Typos:**
- Confirm there are no syntax errors or typographical mistakes around the `TwoOrLess` and `thing` declarations.
- **Linking with Google Mock:**
- Ensure that Google Mock (GMock) is properly linked and installed in the environment since `ASSERT](https://content.bartleby.com/qna-images/question/3d01a12a-1ab5-4b5b-8ac9-ffcff6df37dc/a96e94b6-25b2-42c0-9bfe-535e060f7907/ag5n4do_thumbnail.png)
Transcribed Image Text:### Code Sample
```cpp
#include "two_or_less.hpp"
TwoOrLess thing;
ASSERT_EQ(thing.size(), 0);
thing.insert(0);
ASSERT_EQ(thing.size(), 1);
```
### Your Code's Output
```plaintext
from /usr/include/gmock/internal/gmock-internal-utils.h:47,
from /usr/include/gmock/gmock-actions.h:51,
from /usr/include/gmock/gmock.h:59,
from cpp_unit_test:0:
cpp_unit_test:3:11: error: ‘thing’ was not declared in this scope
ASSERT_EQ(thing.size(), 0);
^~~~~
cpp_unit_test:3:11: note: suggested alternative: ‘this’
cpp_unit_test:4:1: error: ‘thing’ was not declared in this scope
thing.insert(0);
^~~~~
cpp_unit_test:4:1: note: suggested alternative: ‘this’
CMakeFiles/runTests.dir/build.make:62: recipe for target 'CMakeFiles/runTests.dir/tests.cpp.o' failed
make[2]: *** [CMakeFiles/runTests.dir/tests.cpp.o] Error 1
```
### Explanation
The code attempts to include and use a class or header called `TwoOrLess` from the `two_or_less.hpp` file. This snippet is supposed to perform unit tests using Google Mock, indicated by the `ASSERT_EQ` checks.
**Errors Identified:**
1. **Undeclared Variable:**
- The error message `‘thing’ was not declared in this scope` indicates a missing declaration or inclusion issue. The compiler suggests the alternative `‘this’`, which is not relevant in this context.
2. **Build Error:**
- The build is terminated with an error in the CMake process, implying that the compilation of `tests.cpp.o` failed due to the unresolved issues in the above code.
### Recommendations for Resolution:
- **Ensure `TwoOrLess` is Defined:**
- Verify that the `two_or_less.hpp` file is correctly included and that it defines the `TwoOrLess` class.
- **Check for Typos:**
- Confirm there are no syntax errors or typographical mistakes around the `TwoOrLess` and `thing` declarations.
- **Linking with Google Mock:**
- Ensure that Google Mock (GMock) is properly linked and installed in the environment since `ASSERT
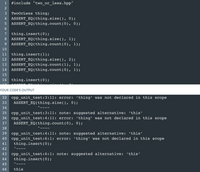
Transcribed Image Text:### C++ Code Example: "TwoOrLess" Class Test
**Code:**
```cpp
#include "two_or_less.hpp"
TwoOrLess thing;
ASSERT_EQ(thing.size(), 0);
ASSERT_EQ(thing.count(0), 0);
thing.insert(0);
ASSERT_EQ(thing.size(), 1);
ASSERT_EQ(thing.count(0), 1);
thing.insert(1);
ASSERT_EQ(thing.size(), 2);
ASSERT_EQ(thing.count(1), 1);
ASSERT_EQ(thing.count(0), 1);
thing.insert(0);
ASSERT_EQ(thing.size(), 2);
```
**Output:**
```
cpp_unit_test:3:11: error: ‘thing’ was not declared in this scope
ASSERT_EQ(thing.size(), 0);
^~~~
cpp_unit_test:3:11: note: suggested alternative: ‘this’
cpp_unit_test:4:11: error: ‘thing’ was not declared in this scope
ASSERT_EQ(thing.count(0), 0);
^~~~
cpp_unit_test:4:11: note: suggested alternative: ‘this’
cpp_unit_test:6:1: error: ‘thing’ was not declared in this scope
thing.insert(0);
^~~~
cpp_unit_test:6:1: note: suggested alternative: ‘this’
```
### Explanation
This code attempts to utilize a class or struct named `TwoOrLess`, performing several operations to test its functionality:
1. **Initialization and Assertion:**
- The `TwoOrLess` object, `thing`, is created but not defined in the scope, leading to compilation errors.
- `ASSERT_EQ` is used to check if the `size()` and `count()` functions return expected values.
2. **Insertion and Verification:**
- The code attempts to insert elements into `thing` and verify its `size` and `count` after each operation.
3. **Errors Encountered:**
- The main issue is the undeclared identifier `thing`, suggesting a lack of appropriate definition or inclusion of necessary header files.
- The error messages suggest replacing `thing` with `this`, which is inappropriate in this context but hints at scope issues.
### Troubleshooting Steps
To resolve these errors, consider the following steps:
- **Include Proper Headers:** Ensure that the `two_or_less.hpp` file is correctly included and
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Using C++ Without Using linked lists: Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a main function that…arrow_forwardIn C++Write the header file (.h file) of a class Counter containing: A data member counter of type int. A data member named counterID of type int. A static int data member named nCounters. A constructor that takes an int argument. A function called increment that accepts no parameters and returns no value. A function called decrement that accepts no parameters and returns no value. A function called getValue that accepts no parameters and returns an int. A function named getCounterID that accepts no parameters and returns an int.arrow_forwardin C++ Define a new “Word” class that must inherit from the given Name class. This class manages a dictionary word info: name (string) and its definition (string). It must prevent the creation of a Word object with either an empty or all-blank word or definition. This class must at least complete the methods defined in the Name class: toString method returns a string representation of the Word object in the format of “WORD( <word> ) DEFINITION( <definition> ) such as WORD(school) DEFINITION(an educational institution) contains method that accepts a search string and returns true if either word or definition contains the search string as a substring. It returns false otherwise. isTheSame method that compares two Word objects and returns true only if both Word objects have the exact same word and definition and false otherwise. Please note that if the given object in the parameter is not a Word object, it will return false.arrow_forward
- Submit code in C++ CODE with comments and output screenshot is must to get Upvote. Thanksarrow_forwardWrite a simple trivia quiz game using c++ Start by creating a Trivia class that contains information about a single trivia question. The class should contain a string for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should be worth more). Add appropriate constructor and accessor functions. In your main function create either an array or a vector of type Trivia and hard-code at least five trivia questions of your choice. Your program should then ask each question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. If so, award the player the dollar amount for that question. If the player enters the wrong answer your program should display the correct answer. When all questions have been asked display the total amount that the player has won.arrow_forwardClasses, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
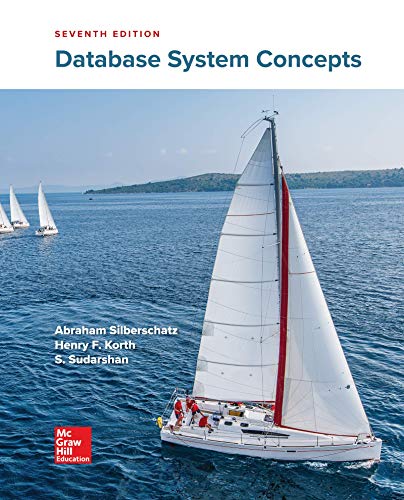
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
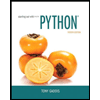
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
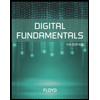
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
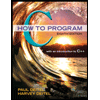
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
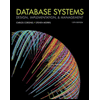
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
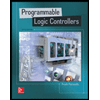
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education