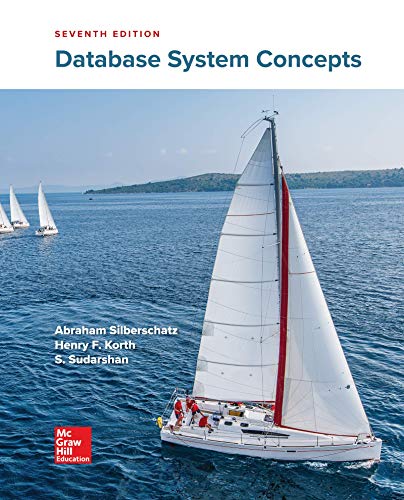
Concept explainers
Write a C++ program with three different files. Each time that the program guesses a number the user responds by telling the program either the correct answer is higher or lower, or that the program’s guess is correct.
A sample run of the program is printed below. In the example the user picks the value 72 for the first game, and then 25 for the second game. The user's input is in bold. Here is how the output might look:
> Think of a number between 1 and 100
> Is the number 50? (h/l/c): h
> Is the number 75? (h/l/c): l
> Is the number 62? (h/l/c): h
> Is the number 69? (h/l/c): h
> Is the number 72? (h/l/c): c
> You picked 72? Great pick.
> Do you want to play again? (y/n): y
> Think of a number Between 1 and 100
> Is the number 50? (h/l/c): l
> Is the number 25? (h/l/c): c
> You picked 25? Great pick.
> Do you want to play again: (y/n): n
> Goodbye.
Notice that the program is guessing numbers, and the user is responding by entering 'h', 'l', or 'c' for higher, lower, or correct.
The point of interest for us is not necessarily the game - which we have already implemented in a previous assignment - but rather the design of the program. The essential part of this assignment is writing a NumberGuesser class. This class will contain all of the logic for remembering the current state of the program’s guesses.
When a new instance of a NumberGuesser class is created the upper and lower bounds of the possible values should be passed into its constructor. From that point on, a NumberGuesser object will always return the midpoint of the possible values when the getCurrentGuess() member function is called. If the higher() or lower() member functions are called, the NumberGuesser object should adjust its private variables to represent the new possible range of values.
For example, if a NumberGuesser is created with the following line of code then the range will be the numbers from 1 to 100:
NumberGuesser guesser(1, 100);
If the getCurrentGuess() method is called it should return 50, which is the midpoint between 1 and 100. If the higher() method is then called, the object should adjust its state accordingly so that it knows that the correct value is now between 51 and 100, inclusive, and getCurrentGuess() would now return 75, the midpoint between 51 and 100. If the lower() method is then called, it should adjust its state to represent that the possible values are now between 51 and 74, and getCurrentGuess() should return 62, the midpoint between 51 and 74. By following this strategy the number guesser should be able to eventually guess the proper value that the user guessed.
As another example, here a different NumberGuesser is created with range between 25 and 35:
NumberGuesser littleGuesser(25, 35);
littleGuesser.getCurrentGuess(); // should return 30
littleGuesser.higher(); // adjusts range to 31-35
littleGuesser.getCurrentGuess(); // should return 33
littleGuesser.reset(); // reset range back to original range between 25-35
littleGuesser.getCurrentGuess(); // should return 30
Here is the basic design of the NumberGuesser class that you should write. The private data variables have been left up to you.
NumberGuesser Class
Private Data ?? |
Public Member Functions and Constructors NumberGuesser(); NumberGuesser(int lowerBound, int upperBound); void higher(); void lower(); int getCurrentGuess(); void reset(); |
The reset() method should return a NumberGuesser object to the state that it was in when it was constructed, i.e. with its original lowerBound and upperBound reset back to their original values. This allows reusing this same NumberGuesser object to play a new game. Hint: you can create extra member variables in your class to store these original values.
Write your NumberGuesser class and test it until you are sure that it is working properly. After it is working, write the rest of the program so that it plays the game by using an instance of your NumberGuesser class.
Note: Your NumberGuesser class should not contain any cin or cout statements. It is only responsible for handling the indicated methods. All of the input and output work should be handled elsewhere in your program, e.g. in main().
What to submit?
You should create three files:
- NumberGuesser.h: NumberGuesser class definition
- NumberGuesser.cpp: NumberGuesser class implementation
- main.cpp: main program to run the game using your NumberGuesser class, including sample output at the bottom
When you are finished, create a zip file containing these three files, and submit the zip file via Canvas. Do not submit the three files separately.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- in C++ here is my code that will ask to answer the multiplication fo teo random numbers when the user enter the right answer, it says very good then it keeps asking the next random set of numbers multiplication answer, till the user answer incorrectly, it says please try again then it exists, i want the program not to exit but to say please try again till the user puts the right answer then it says very good and does to the next question ...etc... can you implemnt what i just asked ? and thank you code: #include<iostream>// to be able to use input output stream#include<math.h>//h is a header file in the standard library of the C programming language designed for basic mathematical operations#include<cstdlib>//Converts a string into a long integer, also carry the library for definition of memory allocation and random processe#include<ctime>//converts the given time since epoch to a calendar local time and then to a character representation.using namespace…arrow_forwardC++arrow_forwardYou are working for a lumber company, and your employer would like a program that calculates the cost of lumber for an order. The company sells pine, fir, cedar, maple, and oak lumber. Lumber is priced by board feet. One board foot equals one square foot that is one inch thick. The price per board foot is given in the following table: The lumber is sold in different dimensions (specified in inches of width and height, and feet of length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4 inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). An entry from the user will be in the form of a letter and four integer numbers. The integers are the number of pieces, width, height, and length. The letter will be one of P, F, C, M, O (corresponding to the five kinds of wood) or T, meaning total. When the letter is T, there are no integers following it on the line. The program…arrow_forward
- Write a program that allows a player to play Rock, Paper, and Scissors against the computer. In this version, if there is a tie the computer wins. The user must beat the computer to win around.The player will provide their name and the number of rounds they want to play. They will begin by entering their name and the number of rounds they would like to play. For each round, the player will input a character to represent their play (‘R’ for rock, ‘P’ for paper, or ‘S’ for scissors). The program will randomly select its play and output whether the player won or lost. After all, rounds have been completed the program will output the match-winner. In the case that the player wins the match, it will output their percentage of wins otherwise it will output the percentage of losses. Use the following functions / descriptions for your code. You may (should) add more functions as you deem necessary, but you may not omit or modify the functionality described below (Don’t forget you will also…arrow_forwardCode a game of sudoku in C++ where the user needs to guess the missing numbers in the grid. The grid will be displayed with given random numbers and the empty places where the user needs to find the number. The user will be given three attempts to solve it, on the third attempt they will be prompted on whether they want to replay the game or quit.arrow_forwardIN PYTHON Write a script which uses the input function to read a string, an int, and a float, as input from keyboard prompts the user to enter his/her name as string, his/her age as integer value, and his/her income as a decimal. For example your output will display as: Mark is 30 years old and his income is 2000 Please show code.arrow_forward
- In C++ only Create a function called MultyDieRoll. It should take in an integer and run the function DieRoll that number of times, printing out each result to the console. So for instance, if you pass in a 7, it should output 7 random numbers; if you pass in a 21, it should output 21 random numbers.arrow_forwardUsing a Sentinel Value to Control a while Loop Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the average…arrow_forwardflowchart of Many treadmills output the speed of the treadmill in miles per hour (mph) on the console, but most runners think of speed in terms of a pace. A common pace is the number of minutes and seconds per mile instead of mph. You want to solve this problem by developing a program. Your program startswith a quantity in mph and converts the quantity into minutes and seconds per mile. As an example, the proper output for an input of 6.5 mph should be 9 minutes and 13.8 seconds per mile.arrow_forward
- Write a C++ program that will input temperatures for consecutive days. The program will store these values into an array and call a function that will return the average of the temperatures. It will also call a function that will return the highest temperature and a function that will return the lowest temperature. The user will input the number of temperatures to be read. There will be no more than 25 temperatures. For Full Credit You must have a comment block header at the top of your program. See the Standards document in Course Content You must use a constant to represent the maximum number of temperature readings You must use the correct data types for your constants and variables You must use an array of the correct data type You must validate your input. Check for number of invalid number of readings (> max, < 1, etc) and display appropriate error message. You must use one or more if/else branches for your decisions You must use a loop to read in the temperatures…arrow_forwardHelp me homework c++ The shots file holds a list of shots (imagine some hitscan weapon in a video game like a shotgun or something). Each shot has an origin and a direction. The origin is an (x,y) coordinate, like (5,3). The direction is a slope and whether the shot is traveling along that slope or in the reverse. A slope of "Vertical" means that the shot is travellingstraight up and down. The format is:x_location y_location slope(either a number like 2.1 or a non-number meaning"Vertical") forwards(1 meaning forwards, 0 meaning backwards) 0 0 0 00 0 0 10 0 Vertical 00 0 Squirrel 110 10 -1 1-10.1 -100.01 2.1 0 The first line is a horizontal line shooting left from the origin (0,0).The second line is a shot also travelling horizontally from the origin, but forward along the x axis instead of backwards.The third line is shooting straight down out of the originThe fourth line is shooting straight up out of the origin (any non-number means Vertical, not just Vertical)The fifth line is…arrow_forwardIn C++, write a program that calculates and prints a monthly paycheck for an employee. The net pay is calculated after taking the following deductions from the employee's gross salary: Federal Income Tax: 17.5% State Tax: 9.1% Social Security Tax: 6.2% Medicare Tax: 1.45% Retirement Plan: 9.29% Health Insurance: $51.48 Life Insurance: $3.71 Your program will prompt the user to input the employee ID, the employee name, and the employee's monthly gross salary. The program will calculate the deductions listed above, along with the percentage of take home pay (defined as the net pay divided by the gross salary). After calculating each deduction and percentage, the output will be printed to the screen (see example output below). Requirements All decimal numbers must be formatted to 2 decimal places. Ask for the employee's ID first, then full name (can include spaces), then the salary. The prompt to enter the salary must include the employee name. The employee ID MUST be…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
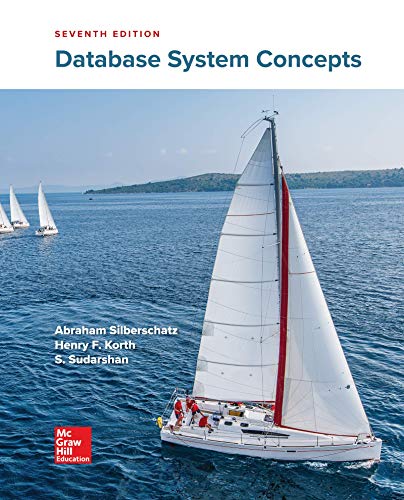
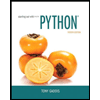
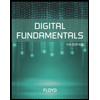
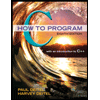
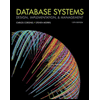
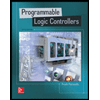