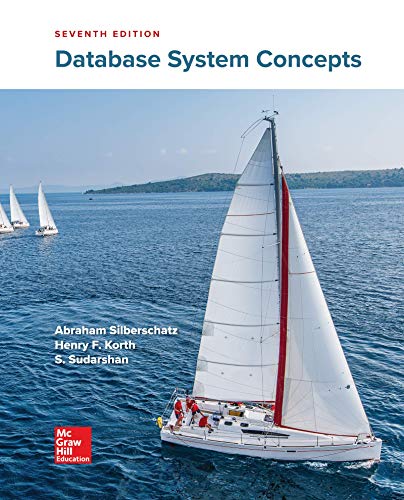
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a C++ or Java program that uses bit strings to find A ∪ B, A ∩ B, and A – B given subsets A and B of a universal set with 10 elements {0, 1, 2, 3, 4, 5, 6, 7, 8,9}. You have to use bit string
Print to the screen set A, set B ( be sure to print out the name of the sets), as well as the set operation results
(be sure to print out the name of the operations). The program requires that elements of subsets A and B are from user input. You can make the assumption that user input numbers are within the domain of the set {0, 1, 2, ..., 9} and there is no improper input.
Additional requirements and reminders:
• The use of STL, templates, and operator overloading is not permitted in any form.
• Remember: You must use a bit string when representing a set. You may not use an array of
Boolean variables.
• The elements of subset A and subset B must from user input. You may not hardcode the two subsets in your program.
Hint:
(1) It is a bit easier to program if we construct the bit string of a set from right to left. For
example, Let U = {1, 2, 3, 4, 5, 6, 7, 8 }. How would you represent the following sets?
A = {1, 2, 6}
B = {2, 4, 6, 8}
A: 00100011
B: 10101010
(be sure to print out the name of the operations). The program requires that elements of subsets A and B are from user input. You can make the assumption that user input numbers are within the domain of the set {0, 1, 2, ..., 9} and there is no improper input.
Additional requirements and reminders:
• The use of STL, templates, and operator overloading is not permitted in any form.
• Remember: You must use a bit string when representing a set. You may not use an array of
Boolean variables.
• The elements of subset A and subset B must from user input. You may not hardcode the two subsets in your program.
Hint:
(1) It is a bit easier to program if we construct the bit string of a set from right to left. For
example, Let U = {1, 2, 3, 4, 5, 6, 7, 8 }. How would you represent the following sets?
A = {1, 2, 6}
B = {2, 4, 6, 8}
A: 00100011
B: 10101010
(2) How to read elements of a set from user input and save it using a bit string?
int setA = 0; // a variable to save the bit string of set A
int userInput; // a variable to save the user input
// put the following code in a loop to continually read user input and
// construct the bit string of set A until encountering an invalid number to stop (e.g. -1)
setA |= (1 << userInput); // | is the bitwise-or; << is the left shift operator
(3) How to find the set operation results? Take the intersection ∩ as an example.
int setA, setB, intersection;
intersection = setA & setB;
Think about how to print to the screen all elements of a set from a bit string.
You may need the right shift operator (>>), the bitwise-and (&), an auxiliary bit string for the
purpose of “mask”, and a loop.
int setA = 0; // a variable to save the bit string of set A
int userInput; // a variable to save the user input
// put the following code in a loop to continually read user input and
// construct the bit string of set A until encountering an invalid number to stop (e.g. -1)
setA |= (1 << userInput); // | is the bitwise-or; << is the left shift operator
(3) How to find the set operation results? Take the intersection ∩ as an example.
int setA, setB, intersection;
intersection = setA & setB;
Think about how to print to the screen all elements of a set from a bit string.
You may need the right shift operator (>>), the bitwise-and (&), an auxiliary bit string for the
purpose of “mask”, and a loop.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In C++, write a program that outputs the nodes of a graph in a breadth first traversal. Data File: Please use this data file. Text to copy: 100 1 3 -9991 4 -9992 5 -9993 2 -9994 -9995 7 8 -9996 4 7 -9997 -9998 -9999 7 8 -999 Diagram: Also, please take a look at figure 20-6 on page 1414 and calculate the weights for the following edges: 0 -> 1 -> 4 0 -> 3 -> 2 -> 5 -> 7 0 -> 3 -> 2 -> 5 -> 8 6 -> 4 6 -> 7 9 -> 7 9 -> 8 To calculates these weights, please assume the following data: 0 -> 1 = 1 0 -> 3 = 2 1 -> 4 = 3 3 -> 2 = 4 2 -> 5 = 5 5 -> 7 = 6 5 -> 8 = 7 6 -> 4 = 8 6 -> 7 = 9 9 -> 7 = 10 9 -> 8 = 11arrow_forwardI need to write a Java ArrayList program called Search.java that counts the names of people that were interviewed from a standard input stream. The program reads people's names from the standard input stream and from time to time prints a sorted portion of the list of names. All the names in the input are given in the order in which they were found and interviewed. You can imagine a unique timestamp associated with each interview. The names are not necessarily unique (many of the people have the same name). Interspersed in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of names to be printed to the standard output stream. The list depends only on the names up to that point in the input stream and does include any people's names that appear in the input stream after the query. The list does not contain all the names of the previous interviews, but only a selection of them. A query provides the names of two people,…arrow_forwardWrite a program in C++ which allows the user to input 3 sets (A,B,C) and to display the cartesian product AxBxC of the 3 sets, and then calculate the number of elements in AxBxC. Keep in mind, if the the input looks like A={a,b,c}, B={1,2,3,4}, C={A,B,C,D,E}, the output should look like “AxBxC = {(a,1,A), … ,(c,4,E)} and it has 60 elements”arrow_forward
- Using c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forwardWrite a C++ program that stores a list of fruits enumerated in Fruits.txt in a STL list of strings. You need to solve the following problem: · output all the fruits in your STL list · output all fruits that start with letter smaller than ‘m’ · output all fruits that contain less than 6 character symbols · calculate the numeric sum of all letters in each fruit and store your answer in a histogram of length 10 where each element contains a value that is a multiple of 500(e.g. your histogram vector contains 10 values and each value represents the numeric value of each fruit (Note: the grand total of values in you histogram should be equal to the total number of fruits · take all the fruits in Fruits.txt and store the in a STL set<string> container · output all the (unique) fruits in Fruits.txt · output the total number of entries in your set Fruits.txt = {pina, naranja, melon, cereza, uva, ciruela, limon, frutabomba, aguacate, platano, manzana, fresa, lechuga, nuez, sandia, mango,…arrow_forwardin c++ please paste working code. the program should Input- Julia Lucas Mia -1 With an expected output of- Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Juliaarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
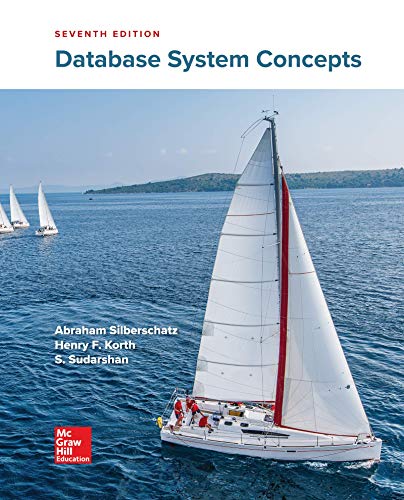
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
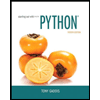
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
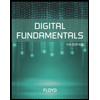
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
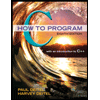
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
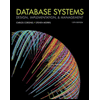
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
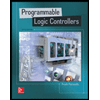
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education