Write a c++ function which is called GetCopy ans IsEqual(testing cannot be modified) charPtr getCopy(const charPtr s) { /* returns a new cstring that is a copy of the cstring s. That is a new cstring with as big memory as the size of the cstring s is created and then all the characters of s including the null char are copied to it. */ int lens = cstrlen(s); char *Array = new char[lens+1]; for(int i = 0; i < lens;i++) { Array[i] = s[i]; } Array[lens] = '\0'; return Array; } bool isEqual(charPtr s1, charPtr s2) { /* returns true if the cstring s1 is equal to the cstring s2 Definition: Two c-strings s1 and s2 are equal if they have the same length and characters of s1 and s2 at corresponding indexes are the same. */ } //Test getCopy function cout << endl << "Testing getCopy function"; cout << endl << "------------------------" << endl; char* s2 = getCopy("irregular"); cout << "A copy of \"irregular\" is s2=\"" << s2 << "\"" << endl; char* s3 = getCopy(s2); cout << "A copy of s2=\"" << s2 << "\" is s3=\"" << s3 << "\"" << endl; delete[] s2; s2 = new char('\0'); cout << "s2 is modified to s2=\"" << s2 << "\" but s3 is still s3=\"" << s3 << "\"" << endl; delete[] s3; s3 = getCopy(s2); cout << "A copy of s2=\"" << s2 << "\" is s3=\"" << s3 << "\"" << endl; //Test isEqual function cout << endl << "Testing isEqual function"; cout << endl << "------------------------" << endl; if (isEqual(s2, s3)) cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are equal" << endl; else cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are not equal" << endl; delete[] s3; s3 = getCopy(s2); if (isEqual(s2, s3)) cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are equal" << endl; else cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are not equal" << endl;
Write a c++ function which is called GetCopy ans IsEqual(testing cannot be modified)
charPtr getCopy(const charPtr s)
{
/*
returns a new cstring that is a copy of the cstring s.
That is a new cstring with as big memory as the size of
the cstring s is created and then all the characters of
s including the null char are copied to it.
*/
int lens = cstrlen(s);
char *Array = new char[lens+1];
for(int i = 0; i < lens;i++)
{
Array[i] = s[i];
}
Array[lens] = '\0';
return Array;
}
bool isEqual(charPtr s1, charPtr s2)
{
/*
returns true if the cstring s1 is equal to the cstring s2
Definition: Two c-strings s1 and s2 are equal if they have the same length
and characters of s1 and s2 at corresponding indexes are the same.
*/
}
//Test getCopy function
cout << endl << "Testing getCopy function";
cout << endl << "------------------------" << endl;
char* s2 = getCopy("irregular");
cout << "A copy of \"irregular\" is s2=\"" << s2 << "\"" << endl;
char* s3 = getCopy(s2);
cout << "A copy of s2=\"" << s2 << "\" is s3=\"" << s3 << "\"" << endl;
delete[] s2;
s2 = new char('\0');
cout << "s2 is modified to s2=\"" << s2 << "\" but s3 is still s3=\"" << s3 << "\"" << endl;
delete[] s3;
s3 = getCopy(s2);
cout << "A copy of s2=\"" << s2 << "\" is s3=\"" << s3 << "\"" << endl;
//Test isEqual function
cout << endl << "Testing isEqual function";
cout << endl << "------------------------" << endl;
if (isEqual(s2, s3))
cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are equal" << endl;
else
cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are not equal" << endl;
delete[] s3;
s3 = getCopy(s2);
if (isEqual(s2, s3))
cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are equal" << endl;
else
cout << "s2=\"" << s2 << "\" and s3=\"" << s3 << "\" are not equal" << endl;

Step by step
Solved in 4 steps with 2 images

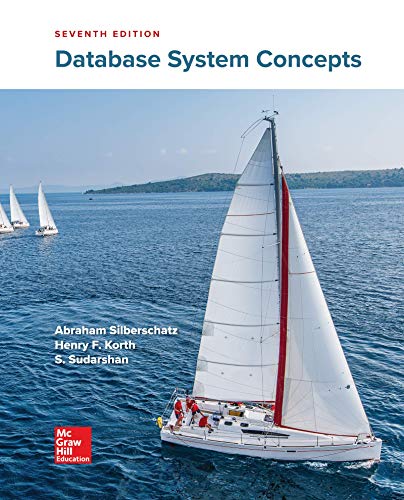
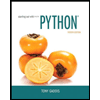
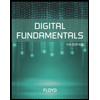
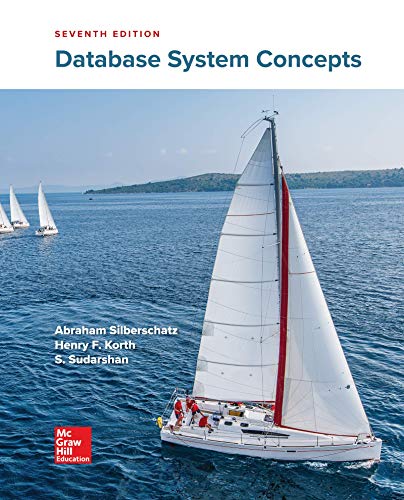
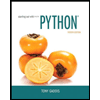
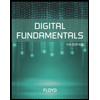
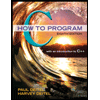
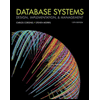
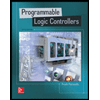